外观模式(Facade)的定义是:为子系统中的一组接口提供一个一致的界面,Facade模式定义了一个高层接口,这个接口使得这一子系统更加容易使用。结构图如下:
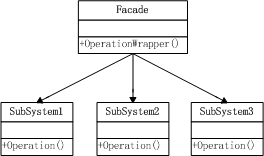
假设有一个抵押系统,存在三个子系统,银行子系统(查询是否有足够多的存款)、信用子系统(查询是否有良好的信用)以及贷款子系统(查询有无贷款劣迹),只有这三个子系统都通过时才可进行抵押。
当客户申请抵押贷款时,他需要分别去这三个子系统办理相关手续,此时若使用外观模式为三个子系统中的接口提供一个统一的服务,这时,客户再办理手续就只需要去一个地方了,结构图如下:
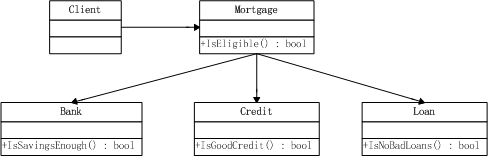
实现代码:
//Bank.h
class Bank
{
public:
Bank();
virtual ~Bank();
bool IsSavingsEnought();
};
//Bank.cpp
#include "stdafx.h"
#include "Mortgage.h"
int main(int argc, char* argv[])
{
Mortgage* pMortgage = new Mortgage;
pMortgage->IsEligible();
return 0;
}
//Credit.h
class Credit
{
public:
Credit();
virtual ~Credit();
bool IsGoodCredit();
};
//Credit.cpp
#include "stdafx.h"
#include "Credit.h"
#include <iostream>
using namespace std;
Credit::Credit()
{
}
Credit::~Credit()
{
}
bool Credit::IsGoodCredit()
{
cout << "信用系统检查是否有良好的信用" << endl;
return true;
}
//Loan.h
class Loan
{
public:
Loan();
virtual ~Loan();
bool IsNoBadLoans();
};
//Loan.cpp
#include "stdafx.h"
#include "Loan.h"
#include <iostream>
using namespace std;
Loan::Loan()
{
}
Loan::~Loan()
{
}
bool Loan::IsNoBadLoans()
{
cout << "贷款系统检查是否没有贷款劣迹" << endl;
return true;
}
//Mortgage.h
class Mortgage
{
public:
Mortgage();
virtual ~Mortgage();
bool IsEligible();
};
//Mortgage.cpp
#include "stdafx.h"
#include "Mortgage.h"
#include "Bank.h"
#include "Credit.h"
#include "Loan.h"
Mortgage::Mortgage()
{
}
Mortgage::~Mortgage()
{
}
bool Mortgage::IsEligible()
{
Bank* pBank = new Bank;
Credit* pCredit = new Credit;
Loan* pLoan = new Loan;
return pBank->IsSavingsEnought() && pCredit->IsGoodCredit() && pLoan->IsNoBadLoans();
}
//main.cpp
#include "stdafx.h"
#include "Mortgage.h"
int main(int argc, char* argv[])
{
Mortgage* pMortgage = new Mortgage;
pMortgage->IsEligible();
return 0;
}
最后输出为:
银行系统检查是否有足够的存款
信用系统检查是否有良好的信用
贷款系统检查是否没有贷款劣迹