模板模式(Template)定义一个操作中算法的骨架,将一些步骤的执行延迟到其子类中。结构图如下:
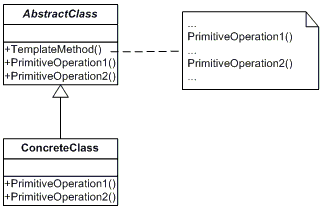
我们将模板模式应用到泡冒排序算法中,将算法中的位置交换及大小比较独立出来作为模板使用,使算法在不改变主程序骨架的情况下支持多种数据类型,结构图如下:
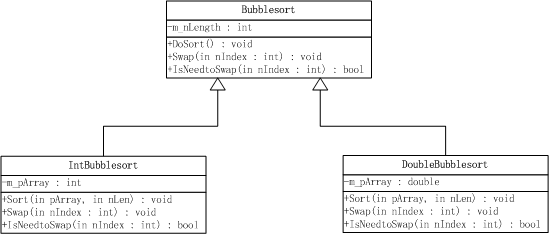
实现代码:
//Bubblesort.h
class Bubblesort
{
public:
virtual ~Bubblesort();
void DoSort();
virtual void Swap(int) = 0;
virtual bool IsNeedtoSwap(int) = 0;
protected:
Bubblesort();
int m_nLength;
};
//Bubblesort.cpp
#include "stdafx.h"
#include "Bubblesort.h"
Bubblesort::Bubblesort()
{
m_nLength = 0;
}
Bubblesort::~Bubblesort()
{
}
void Bubblesort::DoSort()
{
for (int i = m_nLength - 2; i >= 0; --i)
{
for(int j = 0; j <= i; ++j)
{
if(IsNeedtoSwap(j))
{
Swap(j);
}
}
}
}
//IntBubblesort.h
#include <iostream>
#include "Bubblesort.h"
class IntBubblesort : public Bubblesort
{
public:
IntBubblesort();
virtual ~IntBubblesort();
void Sort(int*, int);
void Swap(int);
bool IsNeedtoSwap(int);
friend std::ostream& operator<<(std::ostream& os, IntBubblesort& bubble);
private:
int* m_pArray;
};
//IntBubblesort.cpp
#include "stdafx.h"
#include "IntBubblesort.h"
IntBubblesort::IntBubblesort()
{
}
IntBubblesort::~IntBubblesort()
{
}
void IntBubblesort::Sort(int* pArray, int nLength)
{
this->m_pArray = pArray;
this->m_nLength = nLength;
this->DoSort();
}
void IntBubblesort::Swap(int nIndex)
{
int nTemp = m_pArray[nIndex];
m_pArray[nIndex] = m_pArray[nIndex + 1];
m_pArray[nIndex + 1] = nTemp;
}
bool IntBubblesort::IsNeedtoSwap(int nIndex)
{
return m_pArray[nIndex] > m_pArray[nIndex + 1];
}
std::ostream& operator<<(std::ostream& os, IntBubblesort& bubble)
{
for(int i = 0; i < bubble.m_nLength; ++i)
{
os << bubble.m_pArray[i] << " ";
}
return os;
}
//DoubleBubblesort.h
#include <iostream>
#include "Bubblesort.h"
class DoubleBubblesort : public Bubblesort
{
public:
DoubleBubblesort();
virtual ~DoubleBubblesort();
void Sort(double*, int);
void Swap(int);
bool IsNeedtoSwap(int);
friend std::ostream& operator<<(std::ostream& os, DoubleBubblesort& bubble);
private:
double* m_pArray;
};
//DoubleBubblesort.cpp
#include "stdafx.h"
#include "DoubleBubblesort.h"
#include <iomanip>
DoubleBubblesort::DoubleBubblesort()
{
}
DoubleBubblesort::~DoubleBubblesort()
{
}
void DoubleBubblesort::Sort(double* pArray, int nLength)
{
this->m_pArray = pArray;
this->m_nLength = nLength;
this->DoSort();
}
void DoubleBubblesort::Swap(int nIndex)
{
int nTemp = m_pArray[nIndex];
m_pArray[nIndex] = m_pArray[nIndex + 1];
m_pArray[nIndex + 1] = nTemp;
}
bool DoubleBubblesort::IsNeedtoSwap(int nIndex)
{
return m_pArray[nIndex] > m_pArray[nIndex + 1];
}
std::ostream& operator<<(std::ostream& os, DoubleBubblesort& bubble)
{
for(int i = 0; i < bubble.m_nLength; ++i)
{
os << std::fixed << std::setprecision(2) << bubble.m_pArray[i] << " ";
}
return os;
}
//main.cpp
#include "stdafx.h"
#include "Bubblesort.h"
#include "IntBubblesort.h"
#include "DoubleBubblesort.h"
#include <iostream>
using namespace std;
int main(int argc, char* argv[])
{
int nArray[] = {20, 14, 16, 9, 10, 13, 18};
int nLen = sizeof nArray / sizeof nArray[0];
IntBubblesort intBubble;
intBubble.Sort(nArray, nLen);
cout << "int排序后的结果:" << intBubble << endl;
double dArray[] = {9.6, 10.7, 13.8, 18.5, 28.5};
nLen = sizeof dArray / sizeof dArray[0];
DoubleBubblesort dlBubble;
dlBubble.Sort(dArray, nLen);
cout << "double排序后的结果:" << dlBubble << endl;
return 0;
}
最后输出为:
int排序后的结果:9 10 13 14 16 18 20
double排序后的结果:9.60 10.70 13.80 18.50 28.50