EJB3 supports the three types of inheritance:
EJB3支持三种类型的继承:
-
1.Table per Class Strategy: the <union-class> element in Hibernate
每个类分别一张表
-
2.Single Table per Class Hierarchy Strategy: the <subclass> element in Hibernate
一张总表,即将所有类属性放入一张表
-
3.Joined Subclass Strategy: the <joined-subclass> element in Hibernate
每个子类一张表
下面是实例:Student类(id,name,score)、Teacher类(id,name,title)分别继承自Person类(id,name)
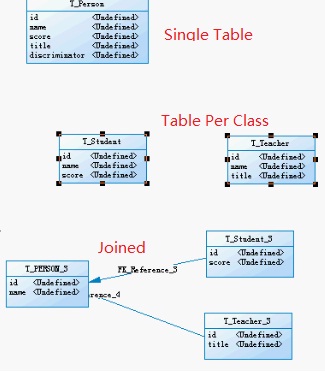 1、Table Per Class Person.java
1 package com.bebig.hibernate.model; 2 3 import javax.persistence.Entity; 4 import javax.persistence.GeneratedValue; 5 import javax.persistence.GenerationType; 6 import javax.persistence.Id; 7 import javax.persistence.Inheritance; 8 import javax.persistence.InheritanceType; 9 import javax.persistence.TableGenerator; 10 11 @Entity 12 @Inheritance(strategy = InheritanceType.TABLE_PER_CLASS) 13 //用表来管理ID 14 @TableGenerator(name = "t_gen", table = "t_gen_table", pkColumnName = "pk_key", valueColumnName = "pk_value", pkColumnValue = "person_pk", initialValue = 1, allocationSize = 1) 15 public class Person { 16 private int id; 17 18 private String name; 19 20 @Id 21 @GeneratedValue(generator = "t_gen", strategy = GenerationType.TABLE) 22 public int getId() { 23 return id; 24 } 25 26 public String getName() { 27 return name; 28 } 29 30 public void setId(int id) { 31 this.id = id; 32 } 33 34 public void setName(String name) { 35 this.name = name; 36 } 37 38 } 39
Student.java
1 package com.bebig.hibernate.model; 2 3 import javax.persistence.DiscriminatorValue; 4 import javax.persistence.Entity; 5 6 @Entity 7 //如果记录为Student,则标识为student 8 @DiscriminatorValue("student") 9 public class Student extends Person { 10 private int score; 11 12 public void setScore(int score) { 13 this.score = score; 14 } 15 16 public int getScore() { 17 return score; 18 } 19 } 20
Teacher.java
1 package com.bebig.hibernate.model; 2 3 import javax.persistence.DiscriminatorValue; 4 import javax.persistence.Entity; 5 6 @Entity 7 //如果记录为Teacher,则标识为teacher 8 @DiscriminatorValue("teacher") 9 public class Teacher extends Person { 10 private String title; 11 12 public void setTitle(String title) { 13 this.title = title; 14 } 15 16 public String getTitle() { 17 return title; 18 } 19 20 } 21
hibernate.cfg.xml
1 <mapping class="com.bebig.hibernate.model.Person" /> 2 <mapping class="com.bebig.hibernate.model.Teacher" /> 3 <mapping class="com.bebig.hibernate.model.Student" />
2、Single Table Person.java
1 package com.bebig.hibernate.model; 2 3 import javax.persistence.DiscriminatorColumn; 4 import javax.persistence.DiscriminatorType; 5 import javax.persistence.DiscriminatorValue; 6 import javax.persistence.Entity; 7 import javax.persistence.GeneratedValue; 8 import javax.persistence.Id; 9 import javax.persistence.Inheritance; 10 import javax.persistence.InheritanceType; 11 12 @Entity 13 @Inheritance(strategy = InheritanceType.SINGLE_TABLE) 14 //加了一个区别字段来标识每条记录是哪一种类型 15 @DiscriminatorColumn(name = "discriminator", discriminatorType = DiscriminatorType.STRING) 16 @DiscriminatorValue("person") 17 public class Person { 18 private int id; 19 20 private String name; 21 22 @Id 23 @GeneratedValue 24 public int getId() { 25 return id; 26 } 27 28 public String getName() { 29 return name; 30 } 31 32 public void setId(int id) { 33 this.id = id; 34 } 35 36 public void setName(String name) { 37 this.name = name; 38 } 39 40 } 41
其它三个文件同上。 3、Joined
Person.java
1 package com.bebig.hibernate.model; 2 3 import javax.persistence.Entity; 4 import javax.persistence.GeneratedValue; 5 import javax.persistence.Id; 6 import javax.persistence.Inheritance; 7 import javax.persistence.InheritanceType; 8 9 @Entity 10 @Inheritance(strategy = InheritanceType.JOINED) 11 public class Person { 12 private int id; 13 14 private String name; 15 16 @Id 17 @GeneratedValue 18 public int getId() { 19 return id; 20 } 21 22 public String getName() { 23 return name; 24 } 25 26 public void setId(int id) { 27 this.id = id; 28 } 29 30 public void setName(String name) { 31 this.name = name; 32 } 33 34 } 35
其它三个文件同上。
|
|
CALENDER
| 日 | 一 | 二 | 三 | 四 | 五 | 六 |
---|
27 | 28 | 29 | 30 | 31 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | 29 | 30 | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
|
常用链接
留言簿
随笔分类
随笔档案
文章分类
文章档案
新闻档案
相册
搜索
最新评论

阅读排行榜
评论排行榜
Powered By: 博客园 模板提供:沪江博客
|