文件结构图 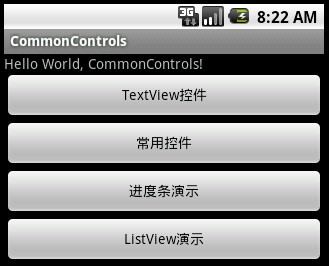
1 package com.bebig.activity04; 2 3 import com.bebig.activity04.R; 4 5 import android.app.Activity; 6 import android.content.Intent; 7 import android.os.Bundle; 8 import android.view.View; 9 import android.view.View.OnClickListener; 10 import android.widget.Button; 11 12 public class Activity04 extends Activity { 13 14 /** *//** Called when the activity is first created. */ 15 private Button btnTextViewDemo = null; 16 private Button btnCommonControlsDemo = null; 17 private Button btnProgressBarDemo = null; 18 private Button btnListViewDemo = null; 19 20 @Override 21 public void onCreate(Bundle savedInstanceState) { 22 super.onCreate(savedInstanceState); 23 setContentView(R.layout.main); 24 25 btnTextViewDemo = (Button) findViewById(R.id.btnTextViewDemo); 26 btnTextViewDemo 27 .setOnClickListener(new btnTextViewDemoOnClickListener()); 28 29 btnCommonControlsDemo = (Button) findViewById(R.id.btnCommonControlsDemo); 30 btnCommonControlsDemo 31 .setOnClickListener(new btnCommonControlsDemoOnClickListener()); 32 33 btnProgressBarDemo = (Button) findViewById(R.id.btnProgressBarDemo); 34 btnProgressBarDemo 35 .setOnClickListener(new btnProgressBarDemoOnClickListener()); 36 37 btnListViewDemo = (Button) findViewById(R.id.btnListViewDemo); 38 btnListViewDemo 39 .setOnClickListener(new btnListViewDemoOnClickListener()); 40 } 41 42 // TextView Demo 43 class btnTextViewDemoOnClickListener implements OnClickListener { 44 45 @Override 46 public void onClick(View arg0) { 47 Intent intent = new Intent(); 48 intent.setClass(Activity04.this, TextViewDemo.class); 49 Activity04.this.startActivity(intent); 50 } 51 52 } 53 54 // CommonControls Demo 55 class btnCommonControlsDemoOnClickListener implements OnClickListener { 56 57 @Override 58 public void onClick(View v) { 59 Intent intent = new Intent(); 60 intent.setClass(Activity04.this, CommonControls.class); 61 Activity04.this.startActivity(intent); 62 } 63 64 } 65 66 // ProgressBar demo 67 class btnProgressBarDemoOnClickListener implements OnClickListener { 68 69 @Override 70 public void onClick(View v) { 71 Intent intent = new Intent(); 72 intent.setClass(Activity04.this, ProgressBarDemo.class); 73 Activity04.this.startActivity(intent); 74 } 75 76 } 77 78 // ListView demo 79 class btnListViewDemoOnClickListener implements OnClickListener { 80 81 @Override 82 public void onClick(View v) { 83 Intent intent = new Intent(); 84 intent.setClass(Activity04.this, ListViewDemo.class); 85 Activity04.this.startActivity(intent); 86 } 87 88 } 89 }
1 package com.bebig.activity04; 2 3 import android.app.Activity; 4 import android.os.Bundle; 5 import android.view.View; 6 import android.view.View.OnClickListener; 7 import android.widget.Button; 8 import android.widget.CheckBox; 9 import android.widget.CompoundButton; 10 import android.widget.RadioButton; 11 import android.widget.RadioGroup; 12 import android.widget.Toast; 13 14 public class CommonControls extends Activity { 15 private StringBuffer hobby = new StringBuffer("爱好:\n"); 16 private String gender = null; 17 18 private Button btnViewResult = null; 19 private CheckBox chkReader = null; 20 private CheckBox chkSwiming = null; 21 private CheckBox chkPlayBasketBall = null; 22 23 private RadioGroup radiogrpGender = null; 24 private RadioButton radioMale = null; 25 private RadioButton radioFemale = null; 26 27 @Override 28 protected void onCreate(Bundle savedInstanceState) { 29 super.onCreate(savedInstanceState); 30 setContentView(R.layout.commoncontrols); 31 32 btnViewResult = (Button) findViewById(R.id.btnViewResult); 33 btnViewResult.setOnClickListener(new btnViewResultOnClickListener()); 34 35 chkReader = (CheckBox) findViewById(R.id.reader); 36 chkReader 37 .setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { 38 @Override 39 public void onCheckedChanged(CompoundButton buttonView, 40 boolean isChecked) { 41 if (isChecked) { 42 hobby.append("看书 "); 43 } else { 44 hobby.delete(hobby.indexOf("看书"), 45 hobby.indexOf("看书") + 3); 46 } 47 } 48 49 }); 50 chkSwiming = (CheckBox) findViewById(R.id.swiming); 51 chkSwiming 52 .setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { 53 @Override 54 public void onCheckedChanged(CompoundButton buttonView, 55 boolean isChecked) { 56 if (isChecked) { 57 hobby.append("游泳 "); 58 } else { 59 hobby.delete(hobby.indexOf("游泳"), 60 hobby.indexOf("游泳") + 3); 61 } 62 } 63 64 }); 65 chkPlayBasketBall = (CheckBox) findViewById(R.id.playbasketball); 66 chkPlayBasketBall 67 .setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { 68 @Override 69 public void onCheckedChanged(CompoundButton buttonView, 70 boolean isChecked) { 71 if (isChecked) { 72 hobby.append("打篮球 "); 73 } else { 74 hobby.delete(hobby.indexOf("打篮球"), 75 hobby.indexOf("打篮球") + 3); 76 } 77 } 78 79 }); 80 81 radioMale = (RadioButton) findViewById(R.id.male); 82 radioFemale = (RadioButton) findViewById(R.id.female); 83 radiogrpGender = (RadioGroup) findViewById(R.id.gender); 84 radiogrpGender 85 .setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() { 86 87 @Override 88 public void onCheckedChanged(RadioGroup group, int checkedId) { 89 if (radioMale.getId() == checkedId) { 90 gender = "性别:男"; 91 } else if (radioFemale.getId() == checkedId) { 92 gender = "性别:女"; 93 } else { 94 gender = ""; 95 } 96 } 97 98 }); 99 } 100 101 class btnViewResultOnClickListener implements OnClickListener { 102 103 @Override 104 public void onClick(View v) { 105 // 查看结果 106 Toast.makeText(CommonControls.this, 107 hobby.toString() + "\n" + gender, Toast.LENGTH_SHORT) 108 .show(); 109 } 110 111 } 112 113 } 114
1 package com.bebig.activity04; 2 3 import java.util.ArrayList; 4 import java.util.HashMap; 5 6 import android.app.ListActivity; 7 import android.os.Bundle; 8 import android.view.View; 9 import android.widget.ListView; 10 import android.widget.SimpleAdapter; 11 12 public class ListViewDemo extends ListActivity { 13 private ArrayList<HashMap<String, String>> goods; 14 @Override 15 protected void onCreate(Bundle savedInstanceState) { 16 super.onCreate(savedInstanceState); 17 setContentView(R.layout.listviewdemo); 18 19 goods = new ArrayList<HashMap<String,String>>(); 20 HashMap<String,String> goods1 = new HashMap<String,String>(); 21 goods1.put("商品编码", "690001"); 22 goods1.put("商品名称", "笔记本"); 23 24 HashMap<String,String> goods2 = new HashMap<String,String>(); 25 goods2.put("商品编码", "690002"); 26 goods2.put("商品名称", "铅笔"); 27 HashMap<String,String> goods3 = new HashMap<String,String>(); 28 goods3.put("商品编码", "690003"); 29 goods3.put("商品名称", "圆珠笔"); 30 HashMap<String,String> goods4 = new HashMap<String,String>(); 31 goods4.put("商品编码", "690004"); 32 goods4.put("商品名称", "钢笔"); 33 HashMap<String,String> goods5 = new HashMap<String,String>(); 34 goods5.put("商品编码", "690005"); 35 goods5.put("商品名称", "毛笔"); 36 HashMap<String,String> goods6 = new HashMap<String,String>(); 37 goods6.put("商品编码", "690006"); 38 goods6.put("商品名称", "黑墨水"); 39 40 41 42 goods.add(goods1); 43 goods.add(goods2); 44 goods.add(goods3); 45 goods.add(goods4); 46 goods.add(goods5); 47 goods.add(goods6); 48 SimpleAdapter listAdapter = new SimpleAdapter(this,goods,R.layout.goods,new String[] {"商品编码","商品名称"},new int[] {R.id.GoodsCode,R.id.GoodsName}); 49 setListAdapter(listAdapter); 50 51 } 52 53 @Override 54 protected void onListItemClick(ListView l, View v, int position, long id) { 55 super.onListItemClick(l, v, position, id); 56 setTitle(goods.get(position).get("商品名称")); 57 } 58 59 } 60
1 package com.bebig.activity04; 2 3 import android.app.Activity; 4 import android.os.Bundle; 5 import android.view.View; 6 import android.view.View.OnClickListener; 7 import android.widget.Button; 8 import android.widget.ProgressBar; 9 10 public class ProgressBarDemo extends Activity { 11 private Button btnBeginDemo = null; 12 private ProgressBar firstProgressBar = null; 13 private ProgressBar secondProgressBar = null; 14 private int progress = 0; 15 16 @Override 17 protected void onCreate(Bundle savedInstanceState) { 18 super.onCreate(savedInstanceState); 19 setContentView(R.layout.progressbardemo); 20 21 firstProgressBar = (ProgressBar) findViewById(R.id.firstProgressBar); 22 secondProgressBar = (ProgressBar) findViewById(R.id.secondProgressBar); 23 24 btnBeginDemo = (Button) findViewById(R.id.btnBegin); 25 btnBeginDemo.setOnClickListener(new btnBeginDemoOnClickListener()); 26 } 27 28 class btnBeginDemoOnClickListener implements OnClickListener { 29 30 @Override 31 public void onClick(View v) { 32 if (firstProgressBar.getProgress() == firstProgressBar.getMax()) { 33 34 firstProgressBar.setProgress(0); 35 firstProgressBar.setSecondaryProgress(0); 36 secondProgressBar.setProgress(0); 37 progress = 0; 38 } else if (firstProgressBar.getProgress() < firstProgressBar 39 .getMax()) { 40 progress += 5; 41 firstProgressBar.setProgress(progress); 42 firstProgressBar.setSecondaryProgress(progress + 10); 43 secondProgressBar.setProgress(progress); 44 } 45 46 } 47 48 } 49 50 } 51
1 package com.bebig.activity04; 2 3 import com.bebig.activity04.R; 4 5 import android.app.Activity; 6 import android.os.Bundle; 7 8 public class TextViewDemo extends Activity { 9 10 @Override 11 protected void onCreate(Bundle savedInstanceState) { 12 super.onCreate(savedInstanceState); 13 setContentView(R.layout.textviewdemo); 14 } 15 16 } 17
以下为控件布局文件代码 commoncontrols.xml
1 <?xml version="1.0" encoding="utf-8"?> 2 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" 3 android:layout_width="wrap_content" 4 android:layout_height="wrap_content" 5 android:orientation="vertical"> 6 7 <EditText android:layout_width="fill_parent" 8 android:layout_height="wrap_content" 9 android:text="@string/commoncontrols" 10 /> 11 <TextView 12 android:layout_width="fill_parent" 13 android:layout_height="wrap_content" 14 android:text="@string/hobby" 15 android:textSize="10pt" 16 android:textStyle="bold" 17 /> 18 <CheckBox 19 android:id="@+id/reader" 20 android:layout_width="fill_parent" 21 android:layout_height="wrap_content" 22 android:text="@string/reader" 23 /> 24 <CheckBox 25 android:id="@+id/swiming" 26 android:layout_width="fill_parent" 27 android:layout_height="wrap_content" 28 android:text="@string/swiming" 29 /> 30 <CheckBox 31 android:id="@+id/playbasketball" 32 android:layout_width="fill_parent" 33 android:layout_height="wrap_content" 34 android:text="@string/playbasketball" 35 /> 36 <TextView 37 android:layout_width="fill_parent" 38 android:layout_height="wrap_content" 39 android:text="@string/sex" 40 android:textSize="10pt" 41 android:textStyle="bold" 42 /> 43 <RadioGroup 44 android:id="@+id/gender" 45 android:layout_width="fill_parent" 46 android:layout_height="wrap_content" 47 > 48 <RadioButton 49 android:id="@+id/male" 50 android:text="@string/male" 51 android:layout_width="fill_parent" 52 android:layout_height="wrap_content" 53 /> 54 <RadioButton 55 android:id="@+id/female" 56 android:text="@string/female" 57 android:layout_width="fill_parent" 58 android:layout_height="wrap_content" 59 /> 60 </RadioGroup> 61 <Button 62 android:id="@+id/btnViewResult" 63 android:layout_width="fill_parent" 64 android:layout_height="wrap_content" 65 android:text="@string/viewresult" 66 /> 67 </LinearLayout> 68
goods.xml
1 <?xml version="1.0" encoding="utf-8"?> 2 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" 3 android:layout_width="wrap_content" android:layout_height="wrap_content" 4 android:orientation="horizontal"> 5 6 <TextView android:id="@+id/GoodsCode" android:layout_width="160px" 7 android:layout_height="wrap_content" 8 android:textSize="10pt" 9 android:gravity="left" 10 android:padding="20dip" 11 /> 12 13 <TextView android:id="@+id/GoodsName" android:layout_width="170px" 14 android:layout_height="wrap_content" 15 android:textSize="10pt" 16 android:gravity="right" 17 android:padding="20dip" 18 /> 19 </LinearLayout> 20
listviewdemo.xml
1 <?xml version="1.0" encoding="utf-8"?> 2 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" 3 android:scrollbars="horizontal" android:layout_width="fill_parent" 4 android:orientation="vertical" android:layout_height="wrap_content"> 5 6 <LinearLayout android:layout_width="fill_parent" 7 android:orientation="horizontal" android:layout_height="wrap_content"> 8 <TextView android:text="商品编码" android:gravity="center" 9 android:layout_width="160px" android:layout_height="wrap_content" 10 android:padding="20dip" android:textSize="10pt" android:textStyle="bold" 11 android:background="#7CFC00"> 12 </TextView> 13 <TextView android:text="商品名称" android:layout_width="170px" 14 android:padding="20dip" android:textSize="10pt" android:gravity="center" 15 android:layout_height="wrap_content" android:textStyle="bold" 16 android:background="#F0E68C"> 17 </TextView> 18 </LinearLayout> 19 20 <!-- 由于ListViewDemo类继承自ListActivity,因此注意这里的ListView id值为@id/android:list --> 21 <ListView android:id="@id/android:list" android:layout_width="fill_parent" 22 android:layout_height="wrap_content" android:scrollbars="vertical" /> 23 24 </LinearLayout> 25
main.xml
1 <?xml version="1.0" encoding="utf-8"?> 2 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" 3 android:orientation="vertical" 4 android:layout_width="fill_parent" 5 android:layout_height="fill_parent" 6 > 7 <TextView 8 android:layout_width="fill_parent" 9 android:layout_height="wrap_content" 10 android:text="@string/hello" 11 /> 12 <Button 13 android:id="@+id/btnTextViewDemo" 14 android:layout_width="fill_parent" 15 android:layout_height="wrap_content" 16 android:text="@string/textview" 17 /> 18 <Button 19 android:id="@+id/btnCommonControlsDemo" 20 android:layout_width="fill_parent" 21 android:layout_height="wrap_content" 22 android:text="@string/commoncontrols" 23 /> 24 <Button 25 android:id="@+id/btnProgressBarDemo" 26 android:layout_width="fill_parent" 27 android:layout_height="wrap_content" 28 android:text="@string/progressbar" 29 /> 30 <Button 31 android:id="@+id/btnListViewDemo" 32 android:layout_width="fill_parent" 33 android:layout_height="wrap_content" 34 android:text="@string/listview" 35 /> 36 </LinearLayout>
progressbardemo.xml
1 <?xml version="1.0" encoding="utf-8"?> 2 <LinearLayout 3 xmlns:android="http://schemas.android.com/apk/res/android" 4 android:layout_width="fill_parent" 5 android:layout_height="wrap_content" 6 android:orientation="vertical" 7 > 8 9 <ProgressBar 10 android:id="@+id/firstProgressBar" 11 android:layout_width="300dp" 12 android:layout_height="wrap_content" 13 android:max="100" 14 android:progress="0" 15 style="?android:attr/progressBarStyleHorizontal" 16 android:visibility="visible" 17 /> 18 <ProgressBar 19 android:id="@+id/secondProgressBar" 20 android:layout_width="wrap_content" 21 android:layout_height="wrap_content" 22 android:max="100" 23 android:progress="0" 24 style="?android:attr/progressBarStyle" 25 android:visibility="visible" 26 /> 27 <Button 28 android:id="@+id/btnBegin" 29 android:layout_width="fill_parent" 30 android:layout_height="wrap_content" 31 android:text="@string/progressbar" 32 /> 33 </LinearLayout> 34
textviewdemo.xml
1 <?xml version="1.0" encoding="utf-8"?> 2 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" 3 android:orientation="vertical" 4 android:layout_width="fill_parent" 5 android:layout_height="fill_parent" 6 > 7 <TextView 8 android:layout_width="fill_parent" 9 android:layout_height="wrap_content" 10 android:text="@string/textview" 11 /> 12 </LinearLayout>
字符串文件 string.xml
1 <?xml version="1.0" encoding="utf-8"?> 2 <resources> 3 <string name="hello">Hello World, CommonControls!</string> 4 <string name="app_name">CommonControls</string> 5 <string name="textview">TextView控件</string> 6 <string name="commoncontrols">常用控件</string> 7 <string name="hobby">爱好</string> 8 <string name="reader">看书</string> 9 <string name="swiming">游泳</string> 10 <string name="playbasketball">打篮球</string> 11 <string name="sex">性别</string> 12 <string name="male">男</string> 13 <string name="female">女</string> 14 <string name="viewresult">查看结果</string> 15 <string name="progressbar">进度条演示</string> 16 <string name="listview">ListView演示</string> 17 18 </resources> 19
|
|
CALENDER
| 日 | 一 | 二 | 三 | 四 | 五 | 六 |
---|
29 | 30 | 31 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | 29 | 30 | 31 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 |
|
常用链接
留言簿
随笔分类
随笔档案
文章分类
文章档案
新闻档案
相册
搜索
最新评论

阅读排行榜
评论排行榜
Powered By: 博客园 模板提供:沪江博客
|