select ShareAm.date,SharePm.code,ShareAm.opening,SharePm.open,100*(SharePm.open - ShareAm.opening)/ShareAm.opening as L
from SharePm,QQ,ShareAm
where ShareAm.date = QQ.date and
SharePm.code = QQ.code and
ShareAm.code = SharePm.code and
date(ShareAm.date,'+1 day') = SharePm.date
order by SharePm.date asc
新做一个选股软件 计算力惊人
一次可分析1400多股,3天涨幅远超泸深300
涨幅为6.8
同期泸深为-3.5
每日开盘自动邮件推送选股
想要的可以加群 蜗牛群:297919841
ps:本人不是卖软件的的!
每日数据在这里www.snail007.com/blog
#include <QHBoxLayout>
#include <QPushButton>
#include <QPlainTextEdit>
#include <QVBoxLayout>
#include <QTableView>
#include <QSqlQueryModel>
#include <QSpacerItem>
#include <QHeaderView>
#include <QMessageBox>
#include <QSqlDatabase>
#include <QSqlError>
#include <QSqlQuery>
static bool createConnection()
{
QSqlDatabase db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName(":memory:");
db.open();
QSqlQuery query;
query.exec("create table person (id int primary key, "
"firstname varchar(20), lastname varchar(20))");
query.exec("insert into person values(101, 'Danny', 'Young')");
query.exec("insert into person values(102, 'Christine', 'Holand')");
query.exec("insert into person values(103, 'Lars', 'Gordon')");
query.exec("insert into person values(104, 'Roberto', 'Robitaille')");
query.exec("insert into person values(105, 'Maria', 'Papadopoulos')");
query.exec("create table offices (id int primary key,"
"imagefile int,"
"location varchar(20),"
"country varchar(20),"
"description varchar(100))");
query.exec("insert into offices "
"values(0, 0, 'Oslo', 'Norway',"
"'Oslo is home to more than 500 000 citizens and has a "
"lot to offer.It has been called \"The city with the big "
"heart\" and this is a nickname we are happy to live up to.')");
query.exec("insert into offices "
"values(1, 1, 'Brisbane', 'Australia',"
"'Brisbane is the capital of Queensland, the Sunshine State, "
"where it is beautiful one day, perfect the next. "
"Brisbane is Australia''s 3rd largest city, being home "
"to almost 2 million people.')");
query.exec("insert into offices "
"values(2, 2, 'Redwood City', 'US',"
"'You find Redwood City in the heart of the Bay Area "
"just north of Silicon Valley. The largest nearby city is "
"San Jose which is the third largest city in California "
"and the 10th largest in the US.')");
query.exec("insert into offices "
"values(3, 3, 'Berlin', 'Germany',"
"'Berlin, the capital of Germany is dynamic, cosmopolitan "
"and creative, allowing for every kind of lifestyle. "
"East meets West in the metropolis at the heart of a "
"changing Europe.')");
query.exec("insert into offices "
"values(4, 4, 'Munich', 'Germany',"
"'Several technology companies are represented in Munich, "
"and the city is often called the \"Bavarian Silicon Valley\". "
"The exciting city is also filled with culture, "
"art and music. ')");
query.exec("insert into offices "
"values(5, 5, 'Beijing', 'China',"
"'Beijing as a capital city has more than 3000 years of "
"history. Today the city counts 12 million citizens, and "
"is the political, economic and cultural centre of China.')");
query.exec("create table images (locationid int, file varchar(20))");
query.exec("insert into images values(0, 'images/oslo.png')");
query.exec("insert into images values(1, 'images/brisbane.png')");
query.exec("insert into images values(2, 'images/redwood.png')");
query.exec("insert into images values(3, 'images/berlin.png')");
query.exec("insert into images values(4, 'images/munich.png')");
query.exec("insert into images values(5, 'images/beijing.png')");
return true;
}
SQLBrowser::SQLBrowser(QWidget* parent):
QWidget(parent)
{
createConnection();
QPushButton* button = new QPushButton("执行");
sql = new QPlainTextEdit();
sql->setPlainText("select * from person");
table = new QTableView();
table->setSortingEnabled(true);
connect(table->horizontalHeader(),SIGNAL(sectionClicked(int)),this,SLOT(sortByColumn(int)));
QVBoxLayout* boxLayout = new QVBoxLayout(this);
QHBoxLayout* hLayout = new QHBoxLayout();
hLayout->addWidget(sql,3);
hLayout->addWidget(button,1);
boxLayout->addLayout(hLayout,1);
boxLayout->addWidget(table,3);
connect(button,SIGNAL(clicked()),this,SLOT(execute()));
model.
}
void SQLBrowser::execute()
{
model.setQuery(sql->toPlainText().trimmed());
model.setHeaderData(0,Qt::Horizontal,QObject::tr("ID"));
model.setHeaderData(1,Qt::Horizontal,QObject::tr("First name"));
model.setHeaderData(2,Qt::Horizontal,QObject::tr("Last name"));
model.setHeaderData(3,Qt::Horizontal,QObject::tr("Last name"));
table->setModel(&model);
}
void SQLBrowser::sortByColumn(int col)
{
model.sort(col,Qt::DescendingOrder);
}
class Anim : public QWidget
{
Q_OBJECT
public:
Anim(QWidget *parent = 0, Qt::WFlags flags = 0);
~Anim();
public:
void createWidget();
void createWidgetV();
void createWidgetH();
void createTransitionV();
void createTransitionHRetrun();
void createTransitionVRetrun();
void createTransitionH();
private:
QStateMachine* machine;
QState* stateV;
QState* stateH;
QState* state;
QWidget* widget;
QWidget* widgetV;
QWidget* widgetH;
QPushButton* hButton;
QPushButton* vButton;
QPushButton* hReturn;
QPushButton* vReturn;
};
Anim::Anim(QWidget* parent,Qt::WFlags flags):
QWidget(parent,flags | Qt::MSWindowsFixedSizeDialogHint)
{
machine = new QStateMachine(this);
resize(320,280);
createWidget();
createWidgetV();
createWidgetH();
state = new QState(machine);
stateV = new QState(machine);
stateH = new QState(machine);
state->assignProperty(widget, "geometry",QRect(0,0,320,280));
state->assignProperty(widgetH,"geometry",QRect(320,0,0,280));
state->assignProperty(widgetV,"geometry",QRect(0,280,320,0));
stateH->assignProperty(widget, "geometry",QRect(0,0,0,280));
stateH->assignProperty(widgetH,"geometry",QRect(0,0,320,280));
stateH->assignProperty(widgetV,"geometry",QRect(0,280,320,0));
stateV->assignProperty(widget, "geometry",QRect(0,0,320,0));
stateV->assignProperty(widgetH,"geometry",QRect(0,0,0,280));
stateV->assignProperty(widgetV,"geometry",QRect(0,0,320,280));
createTransitionHRetrun();
createTransitionVRetrun();
createTransitionH();
createTransitionV();
machine->setInitialState(state);
machine->start();
}
Anim::~Anim()
{
}
void Anim::createWidget()
{
widget = new QWidget(this);
widget->resize(320,280);
QVBoxLayout* vLayout = new QVBoxLayout(widget);
vButton = new QPushButton("V Click");
hButton = new QPushButton("H Click");
QHBoxLayout* hLayout = new QHBoxLayout();
hLayout->addWidget(vButton);
hLayout->addWidget(hButton);
vLayout->addLayout(hLayout);
vLayout->addWidget(new QTextEdit());
}
void Anim::createWidgetV()
{
widgetV = new QWidget(this);
widgetV->resize(320,0);
widgetV->move(0,280);
QVBoxLayout* vLayout = new QVBoxLayout(widgetV);
vReturn = new QPushButton("V Return");
vLayout->addWidget(vReturn);
vLayout->addWidget(new QTextEdit());
}
void Anim::createWidgetH()
{
widgetH = new QWidget(this);
widgetH->resize(0,280);
widgetH->move(320,0);
QVBoxLayout* vLayout = new QVBoxLayout(widgetH);
hReturn = new QPushButton("H Return");
vLayout->addWidget(hReturn);
vLayout->addWidget(new QTextEdit());
}
void Anim::createTransitionH()
{
QPropertyAnimation* widgetAnim = new QPropertyAnimation(widget,"geometry");
widgetAnim->setDuration(800);
widgetAnim->setEasingCurve(QEasingCurve::Linear);
QPropertyAnimation* widgetHAnim = new QPropertyAnimation(widgetH,"geometry");
widgetHAnim->setDuration(800);
widgetHAnim->setEasingCurve(QEasingCurve::Linear);
QSignalTransition* transition = state->addTransition(hButton,SIGNAL(clicked()),stateH);
transition->addAnimation(widgetAnim);
transition->addAnimation(widgetHAnim);
}
void Anim::createTransitionV()
{
QPropertyAnimation* widgetAnim = new QPropertyAnimation(widget,"geometry");
widgetAnim->setDuration(800);
widgetAnim->setEasingCurve(QEasingCurve::Linear);
QPropertyAnimation* widgetVAnim = new QPropertyAnimation(widgetV,"geometry");
widgetVAnim->setDuration(800);
widgetVAnim->setEasingCurve(QEasingCurve::Linear);
QSignalTransition* transition = state->addTransition(vButton,SIGNAL(clicked()),stateV);
transition->addAnimation(widgetAnim);
transition->addAnimation(widgetVAnim);
}
void Anim::createTransitionHRetrun()
{
QPropertyAnimation* widgetAnim = new QPropertyAnimation(widget,"geometry");
widgetAnim->setDuration(800);
widgetAnim->setEasingCurve(QEasingCurve::Linear);
QPropertyAnimation* widgetHAnim = new QPropertyAnimation(widgetH,"geometry");
widgetHAnim->setDuration(800);
widgetHAnim->setEasingCurve(QEasingCurve::Linear);
QSignalTransition* transition = stateH->addTransition(hReturn,SIGNAL(clicked()),state);
transition->addAnimation(widgetAnim);
transition->addAnimation(widgetHAnim);
}
void Anim::createTransitionVRetrun()
{
QPropertyAnimation* widgetAnim = new QPropertyAnimation(widget,"geometry");
widgetAnim->setDuration(800);
widgetAnim->setEasingCurve(QEasingCurve::Linear);
QPropertyAnimation* widgetVAnim = new QPropertyAnimation(widgetV,"geometry");
widgetVAnim->setDuration(800);
widgetVAnim->setEasingCurve(QEasingCurve::Linear);
QSignalTransition* transition = stateV->addTransition(vReturn,SIGNAL(clicked()),state);
transition->addAnimation(widgetAnim);
transition->addAnimation(widgetVAnim);
}
class Widget : public QWidget
{
Q_OBJECT
public:
explicit Widget(QWidget *parent = 0);
~Widget();
private slots:
void readMessage();
void displayError(QAbstractSocket::SocketError);
void sendMessage();
void connected();
void disconnected();
void onError(QAbstractSocket::SocketError);
void onProgress();
private:
QTcpSocket* tcpsocket;
bool flag;
QTimer timer;
QString message;
QPushButton* button;
};
Widget::Widget(QWidget* parent):
QWidget(parent)
{
button = new QPushButton("Send");
QVBoxLayout* layout = new QVBoxLayout(this);
layout->addWidget(button);
connect(button,SIGNAL(clicked()),this,SLOT(sendMessage()));
tcpsocket = new QTcpSocket(this);
flag = false;
//tcpsocket->abort();
//tcpsocket->connectToHost("10.2.100.63",80);
connect(tcpsocket,SIGNAL(readyRead()),this,SLOT(readMessage()));
connect(tcpsocket,SIGNAL(connected()),this,SLOT(connected()));
connect(tcpsocket,SIGNAL(disconnected()),this,SLOT(disconnected()));
connect(tcpsocket,SIGNAL(error(QAbstractSocket::SocketError)),this,SLOT(onError(QAbstractSocket::SocketError)));
connect(&timer,SIGNAL(timeout()),this,SLOT(onProgress()));
timer.start(1800);
}
Widget::~Widget()
{
}
void Widget::onProgress()
{
if(flag == false)
{
tcpsocket->abort();
tcpsocket->connectToHost("10.2.100.63",80);
}
}
void Widget::readMessage()
{
QString tag = tcpsocket->readAll();
std::cout<<"tag:"<<tag.size()<<std::endl;
}
void Widget::displayError(QAbstractSocket::SocketError)
{
qDebug() << tcpsocket->errorString();
}
void Widget::sendMessage()
{
std::cout<<"write callback data."<<std::endl;
tcpsocket->write("ok");
}
void Widget::connected()
{
flag = true;
}
void Widget::disconnected()
{
flag = false;
}
void Widget::onError(QAbstractSocket::SocketError)
{
}
class Widget : public QWidget
{
Q_OBJECT
public:
explicit Widget(QWidget *parent = 0);
~Widget();
public slots:
void newConnect();
void readMessage(int i);
void onClick();
void displayError(QAbstractSocket::SocketError);
private:
Ui::Widget* ui;
QSignalMapper* mapper;
QTcpServer* tcpServer;
QTcpSocket* tcpSocket[8];
int socketID;
};
Widget::Widget(QWidget* parent):
QWidget(parent),
ui(new Ui::Widget)
{
ui->setupUi(this);
tcpServer = new QTcpServer(this);
if(!tcpServer->listen(QHostAddress("10.2.100.63"),80))
{
QString error = tcpServer->errorString();
std::cout<<"error:"<<qPrintable(error)<<std::endl;
close();
}
socketID = -1;
mapper = new QSignalMapper(this);
connect(tcpServer,SIGNAL(newConnection()),this,SLOT(newConnect()));
connect(ui->ok,SIGNAL(clicked()),this,SLOT(onClick()));
connect(mapper,SIGNAL(mapped(int)),this,SLOT(readMessage(int)));
}
Widget::~Widget()
{
delete ui;
}
void Widget::readMessage(int i)
{
QString tag = tcpSocket[i]->readAll();
std::cout<<"result[0]:"<<tag.size()<<std::endl;
}
void Widget::displayError(QAbstractSocket::SocketError)
{
}
void Widget::newConnect()
{
socketID ++;
tcpSocket[socketID] = tcpServer->nextPendingConnection();
std::cout<<"connect:"<<tcpSocket[socketID]<<std::endl;
mapper->setMapping(tcpSocket[socketID],socketID);
connect(tcpSocket[socketID],SIGNAL(readyRead()),mapper,SLOT(map()));
}
void Widget::onClick()
{
if(socketID == -1)
return;
std::cout<<"send data:"<<std::endl;
QByteArray block;
for(int i=0;i<3000;i++)
{
block.append("123456");
}
for(int i=0;i<socketID;i++)
{
tcpSocket[i]->write(block.mid(i*block.size()/(i+2),block.size()/(i+2)));
}
ui->content->setPlainText(block.mid(block.size()*(socketID-1)/(2+socketID)));
}
例子为Server发送数据给Client,Client处理后返回数据给Server
1.Server
class Widget : public QWidget
{
Q_OBJECT
public:
explicit Widget(QWidget *parent = 0);
~Widget();
public slots:
void newConnect();
void readMessage();
void onClick();
void displayError(QAbstractSocket::SocketError);
private:
Ui::Widget* ui;
QTcpServer* tcpServer;
QTcpSocket* tcpSocket;
};
Widget::Widget(QWidget* parent):
QWidget(parent),
ui(new Ui::Widget)
{
ui->setupUi(this);
tcpServer = new QTcpServer(this);
if(!tcpServer->listen(QHostAddress("10.2.100.67"),80))
{
QString error = tcpServer->errorString();
std::cout<<"error:"<<qPrintable(error)<<std::endl;
close();
}
connect(tcpServer,SIGNAL(newConnection()),this,SLOT(newConnect()));
connect(ui->ok,SIGNAL(clicked()),this,SLOT(onClick()));
}
Widget::~Widget()
{
delete ui;
}
void Widget::readMessage()
{
QString tag = tcpSocket->read(6);
std::cout<<"result:"<<qPrintable(tag)<<std::endl;
}
void Widget::displayError(QAbstractSocket::SocketError)
{
}
void Widget::newConnect()
{
std::cout<<"connect."<<std::endl;
tcpSocket = tcpServer->nextPendingConnection();
connect(tcpSocket,SIGNAL(readyRead()),this,SLOT(readMessage()));
}
void Widget::onClick()
{
if(!tcpSocket)
return;
std::cout<<"send data:"<<std::endl;
QByteArray block;
QString tag("6");
block.append(tag);
block.append(",");
for(int i=0;i<6;i++)
{
block.append("123456");
block.append(",");
}
block = block.mid(0,block.size()-1);
tcpSocket->write(block);
ui->content->setPlainText("send success");
}
Client
class Widget : public QWidget
{
Q_OBJECT
public:
explicit Widget(QWidget *parent = 0);
~Widget();
private slots:
void readMessage();
void displayError(QAbstractSocket::SocketError);
void sendMessage();
private:
QTcpSocket* tcpsocket;
QString message;
QPushButton* button;
};
Widget::Widget(QWidget* parent):
QWidget(parent)
{
button = new QPushButton("Send");
QVBoxLayout* layout = new QVBoxLayout(this);
layout->addWidget(button);
connect(button,SIGNAL(clicked()),this,SLOT(sendMessage()));
tcpsocket = new QTcpSocket(this);
tcpsocket->abort();
tcpsocket->connectToHost("10.2.100.67",80);
connect(tcpsocket,SIGNAL(readyRead()),this,SLOT(readMessage()));
connect(tcpsocket,SIGNAL(error(QAbstractSocket::SocketError)),this,SLOT(displayError(QAbstractSocket::SocketError)));
}
Widget::~Widget()
{
}
void Widget::readMessage()
{
QString tag = tcpsocket->read(1);
int count = tag.toInt();
if(count > 0)
{
std::cout<<"data length:"<<count<<std::endl;
int i = 0;
QString data;
while(true)
{
QString block = tcpsocket->read(60);
if(block.isEmpty())
break;
data += block;
i ++;
}
data = data.mid(1);
QStringList list = data.split(",");
foreach(QString item,list)
std::cout<<qPrintable(item)<<std::endl;
}
}
void Widget::displayError(QAbstractSocket::SocketError)
{
qDebug() << tcpsocket->errorString();
}
void Widget::sendMessage()
{
std::cout<<"write callback data."<<std::endl;
tcpsocket->write("ok");
}
#include <QtDebug>
#include <QFile>
#include <QTextStream>
#include <QCoreApplication>
static void customMessageHandler(QtMsgType type,const char* msg)
{
QString txt;
switch(type)
{
case QtDebugMsg:
txt = QString("Debug: %1").arg(msg);
break;
case QtWarningMsg:
txt = QString("Warning: %1").arg(msg);
break;
case QtCriticalMsg:
txt = QString("Critical: %1").arg(msg);
break;
case QtFatalMsg:
txt = QString("Fatal: %1").arg(msg);
abort();
default:
break;
}
QFile outFile("log.txt");
outFile.open(QIODevice::WriteOnly | QIODevice::Append);
QTextStream ts(&outFile);
ts << txt << endl;
}
int main(int argc,char* argv[])
{
QCoreApplication app(argc,argv);
qInstallMsgHandler(customMessageHandler);
qDebug("This is a debug message");
qWarning("This is a warning message");
return app.exec();
}
如果需要按照数字排序,使用以下方法即可
定制一个项代理
class MyTableWidgetItem : public QTableWidgetItem
{
public:
MyTableWidgetItem(const QString& text):
QTableWidgetItem(text)
{
}
public:
bool operator <(const QTableWidgetItem &other) const
{
return text().toFloat() < other.text().toFloat();
}
};
调用
这样增加数据
MyTableWidgetItem* item = new MyTableWidgetItem(QString("%1").arg(i));
table->setItem(r,l,item);
使用中点击表头即可排序
主要解决了很多故障,增加了一点功能
API上参考了Qt
- 支持png格式
- 支持控件序列化,反序列化
- 支持换肤
- 代码整洁,风格良好
- 提供StackLayout,BoxLayout,GridLayout,FlowLayout
- 提供CheckBox,Panel,StaticText,ImageBox,ProgressBar,TableWidget,Slider,EditFiled,Combox等常用控件
截图如下:
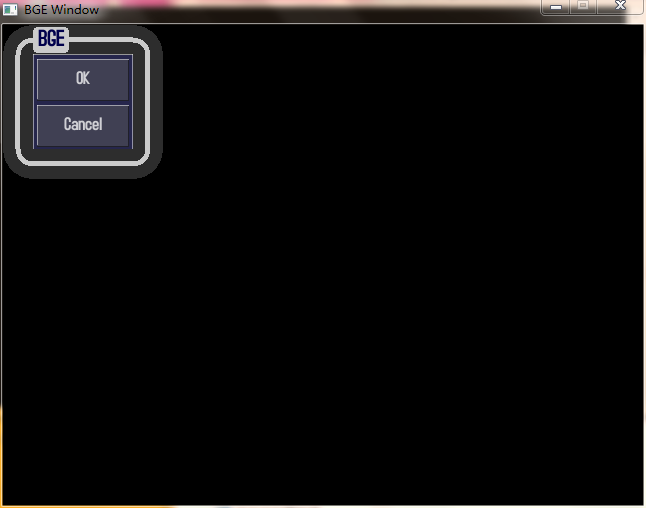
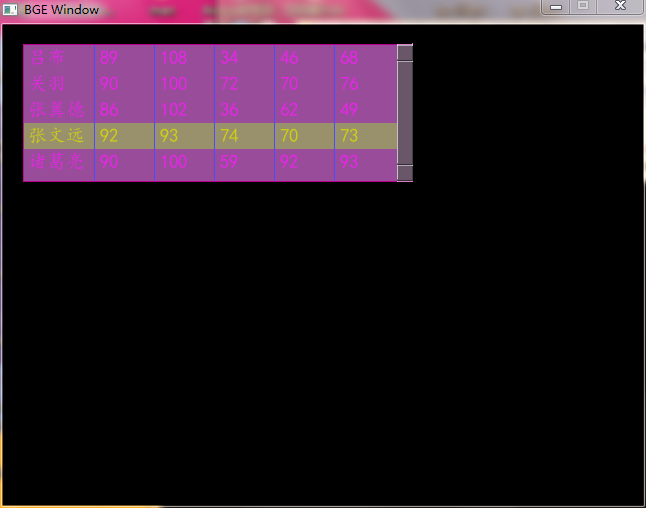
例子1
#include <BGE/All>
using namespace bge;
class Dispatcher : public SlotHolder
{
public:
Dispatcher(ImageBox* image,Button* button)
{
image_ = image;
button_ = button;
count_ = 1;
}
void click()
{
std::string file = ":box/side";
file += toString(count_);
file += ".png";
image_->setImage(file);
count_ ++;
if(count_ == 7)
count_ = 1;
}
private:
ImageBox* image_;
Button* button_;
int count_;
};
int main(int argc,char* argv[])
{
FileSystem::instance().initial(argv[0]);
FileSystem::instance().setResourcePackage("data.zip");
WindowManager::instance().initialize(":accid.ttf",false);
Device* device = Device::create();
device->initial();
device->createWindow(640,480,String("BGE Window"));
Panel* panel = new Panel();
panel->setPosition(Vector2f(20,20));
panel->setSize(Vector2f(128,128+24));
ImageBox* image = new ImageBox();
image->setImage(":box/side6.png");
image->setPosition(Vector2f(0,0));
image->setSize(Vector2f(128,128));
Button* button = new Button();
button->loadAppearance(":buttonskin1.xml");
button->setSize(Vector2f(128,24));
button->setText(L"Click");
Dispatcher dispacher(image,button);
button->clicked.connect(&dispacher,&Dispatcher::click);
BoxLayout* layout = new BoxLayout();
layout->setSpacing(.0f);
panel->setLayout(layout);
layout->setJustification(Orientation_vertical);
layout->addWindow(image);
layout->addWindow(button);
panel->adjust();
WindowManager::instance().addWindow(panel);
while(device->isRunning())
{
device->preRender();
WindowManager::instance().update();
device->swapBuffers();
device->pollEvents();
}
WindowManager::instance().terminate();
device->closeWindow();
device->terminate();
device->deleteLater();
return 0;
}
例子2
#include <BGE/All>
using namespace bge;
class Dispatcher : public SlotHolder
{
public:
Dispatcher(StackLayout* layout,Button* button)
{
layout_ = layout;
button_ = button;
count_ = 0;
}
void click()
{
layout_->setCurrentIndex(count_);
count_ ++;
if(count_ > 5)
count_ = 0;
}
private:
StackLayout* layout_;
Button* button_;
int count_;
};
int main(int argc,char* argv[])
{
FileSystem::instance().initial(argv[0]);
FileSystem::instance().setResourcePackage("data.zip");
WindowManager::instance().initialize(":accid.ttf",false);
Device* device = Device::create();
device->initial();
device->createWindow(640,480,String("BGE Window"));
Panel* panel = new Panel();
panel->setPosition(Vector2f(20,20));
panel->setSize(Vector2f(128,128+24));
Panel* stackPanel = new Panel();
stackPanel->setSize(Vector2f(128,128));
StackLayout* stackLayout = new StackLayout();
stackPanel->setLayout(stackLayout);
stackLayout->setSpacing(.0f);
for(int i=1;i<7;i++)
{
ImageBox* image = new ImageBox();
std::string file = ":box/side";
file += toString(i);
file += ".png";
image->setImage(file);
image->setPosition(Vector2f(0,0));
image->setSize(Vector2f(128,128));
stackLayout->insertWindow(i,image);
}
Button* button = new Button();
button->loadAppearance(":buttonskin1.xml");
button->setSize(Vector2f(128,24));
button->setText(L"Click");
Dispatcher dispacher(stackLayout,button);
button->clicked.connect(&dispacher,&Dispatcher::click);
BoxLayout* layout = new BoxLayout();
layout->setSpacing(.0f);
panel->setLayout(layout);
layout->setJustification(Orientation_vertical);
layout->addWindow(stackPanel);
layout->addWindow(button);
panel->adjust();
WindowManager::instance().addWindow(panel);
while(device->isRunning())
{
device->preRender();
WindowManager::instance().update();
device->swapBuffers();
device->pollEvents();
}
WindowManager::instance().terminate();
device->closeWindow();
device->terminate();
device->deleteLater();
return 0;
}
这2个显示效果完全一样
欢迎下载
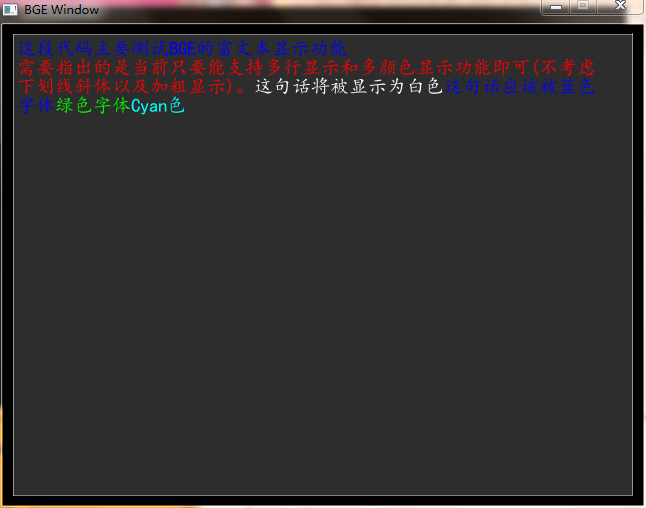
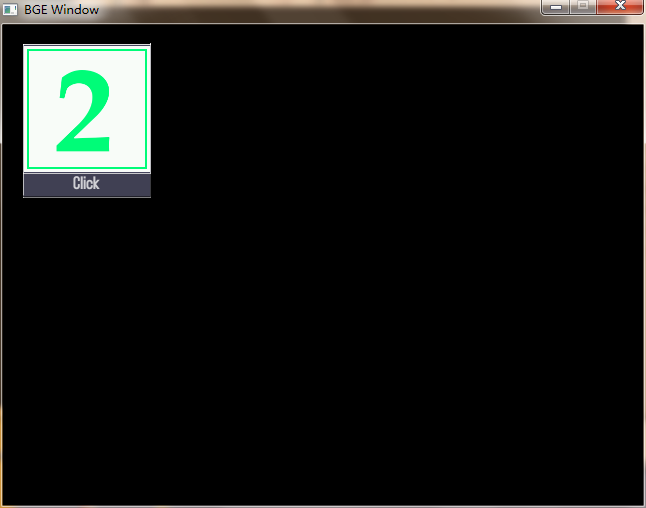
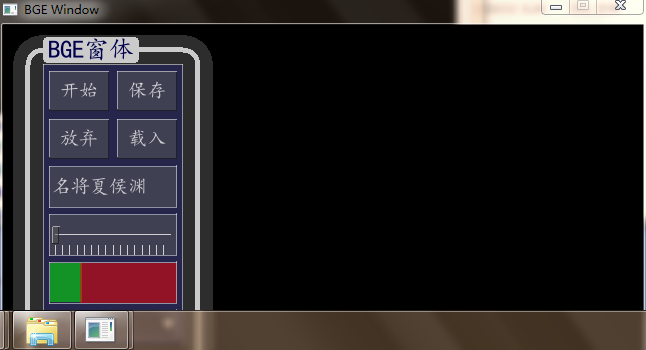
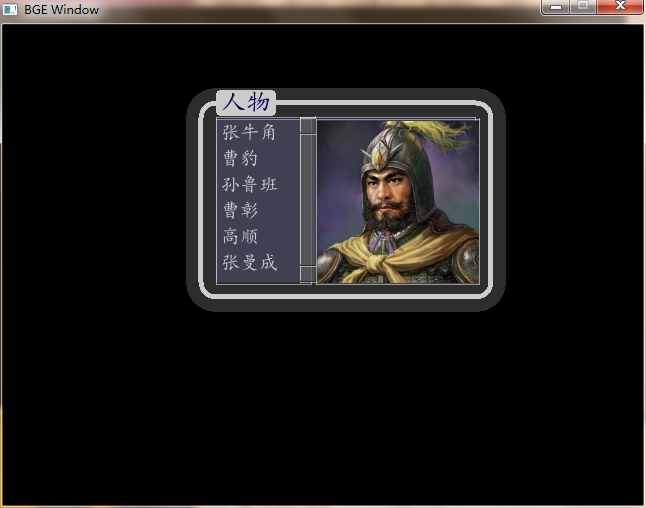
在这里下载:http://download.csdn.net/detail/ccsdu2004/9464121
代码有点大 这里放不下