|
base.h
1 #ifndef BASE_H
2 #define BASE_H
3
4 #include <stdio.h>
5
6 class Base
7 {
8 public:
9 Base(int val) : m_val(val)
10 {
11 printf("Base::Base(int val) @ 0x%08X\n", this);
12 }
13
14 virtual void say()
15 {
16 printf("void Base::say() @ 0x%08X\n", this);
17 printf("val = %d\n", GetVal());
18 }
19
20 virtual ~Base()
21 {
22 printf("Base::~Base() @ 0x%08X\n", this);
23 }
24
25 protected:
26 int GetVal() { return m_val; }
27
28 private:
29 int m_val;
30 };
31
32 #endif
child.h
1 #ifndef CHILD_H
2 #define CHILD_H
3
4 #include <stdio.h>
5 #include "base.h"
6
7 class Child : public Base
8 {
9 public:
10 Child(int val) : Base(val)
11 {
12 printf("Child::Child(int val) @ 0x%08X\n", this);
13 }
14
15 void say()
16 {
17 printf("void Child::say() @ 0x%08X\n", this);
18 printf("val = %d\n", GetVal());
19 }
20
21 ~Child()
22 {
23 printf("Child::~Child() @ 0x%08X\n", this);
24 }
25 };
26
27 #endif
28
main.cpp
1 #include "base.h"
2 #include "child.h"
3
4 static void test0();
5 static void test();
6 static void virfunc_call(Base *base);
7
8 void test0()
9 {
10 int src = 1;
11 int dst;
12
13 #ifdef WIN32
14 dst = src;
15 #else
16 asm ("mov %1, %0\n\t"
17 "add $1, %0"
18 : "=r" (dst)
19 : "r" (src));
20 #endif
21
22 printf("dst=%d\n", dst);
23 }
24
25 void test(Base *base)
26 {
27 int addr = 0;
28
29 #ifdef WIN32
30 printf("[1] addr=0x%08X base=0x%P\n", addr, base);
31 #else
32 printf("[1] addr=0x%08X base=0x%08X\n", addr, base);
33 #endif
34
35 #ifdef WIN32
36 addr = *((int *)base);
37 #else
38 asm (
39 "movl %0, %1\n\t"
40 : "=r" (addr)
41 : "r" (base)
42 );
43 #endif
44
45 #ifdef WIN32
46 printf("[2] addr=0x%08X base=0x%P\n", addr, base);
47 #else
48 printf("[2] addr=0x%08X base=0x%08X\n", addr, base);
49 #endif
50 }
51
52 static void virfunc_call(Base *base)
53 {
54 typedef void (*FUNC)();
55
56 int *pAddrBase = (int *)base;
57 int addr = *(int *)base;
58 int *pVirtualFuncBase = (int *)(*pAddrBase);
59
60 #ifdef WIN32
61 printf("\nvirfunc=0x%P\npAddrBase=0x%P\npVirtualFuncBase=0x%P\n\n", virfunc_call, pAddrBase, pVirtualFuncBase);
62 #else
63 printf("\nvirfunc=0x%08X\npAddrBase=0x%08X\npVirtualFuncBase=0x%08X\n\n", virfunc_call, pAddrBase, pVirtualFuncBase);
64 #endif
65
66 FUNC func = reinterpret_cast<FUNC>(*pVirtualFuncBase);
67
68 #ifdef WIN32
69 __asm {
70 mov ecx, base
71 }
72 #else
73 __asm__ __volatile__(
74 "movl %0, %%ecx"
75 :
76 : "r" (base)
77 :
78 );
79 #endif
80
81 func();
82 }
83
84 int main(int argc, char *argv[])
85 {
86 Base *base1 = nullptr, *base2 = nullptr;
87
88 base1 = new Base(1);
89 // base1->say();
90 virfunc_call(base1);
91 // test(base1);
92 delete base1;
93
94 base2 = new Child(2);
95 // base2->say();
96 virfunc_call(base2);
97 // test(base2);
98 delete base2;
99
100 return 0;
101 }
VC++
cl /DWIN32 main.cpp
G++
g++ -o main main.cpp
bootloader.asm
org 0x7c00
start:
jmp main_entry
stack:
times 128 db 0
tos:
db 0
main_entry:
mov ax, cs
mov ds, ax
mov ax, stack
mov ss, ax
mov sp, start
; initialize es:bx, read data to 0x0000:0x7e00
mov ax, 0x0000
mov es, ax
mov bx, 0x7c00+0x200
mov ah, 0x02 ; read function
mov al, 0x01 ; read 1 sectors
mov ch, 0x00 ;
mov cl, 0x02 ; read from 2nd section
mov dh, 0x00 ;
mov dl, 0x80 ; read from 1st hard disk
int 0x13
jc .die
; install interrupt 0x80
call 0x7e00
; call interrupt 0x80
mov ax, msg1
int 0x80
; call interrupt 0x80
mov ax, msg2
int 0x80
.die:
jmp $
print_char:
mov ah, 0x0e
int 0x10
ret
msg1: db "Loading system ", 0x0d, 0x0a, 0
msg2: db "Hello, World!", 0x0d, 0x0a, 0
crlf: db 0x0d, 0x0a, 0
fill_zero:
times 510-($-$$) db 0
magic:
db 0x55, 0xAA
lib16.asm
org 0x7e00
install_int:
mov ax, 0
mov es, ax
mov ax, int_0x80
mov bx, 0x80*4
mov word [es:bx], ax ; ip
mov word [es:(bx+2)], 0 ; cs
ret
int_0x80:
pusha
mov si, ax
mov ah, 0x0e
mov al, [ds:si]
.loop:
cmp al, 0
je .exit
int 0x10
inc si
mov al, [ds:si]
jmp .loop
.exit:
popa
iret
fill_zero:
times 512-($-$$) db 0
结果图: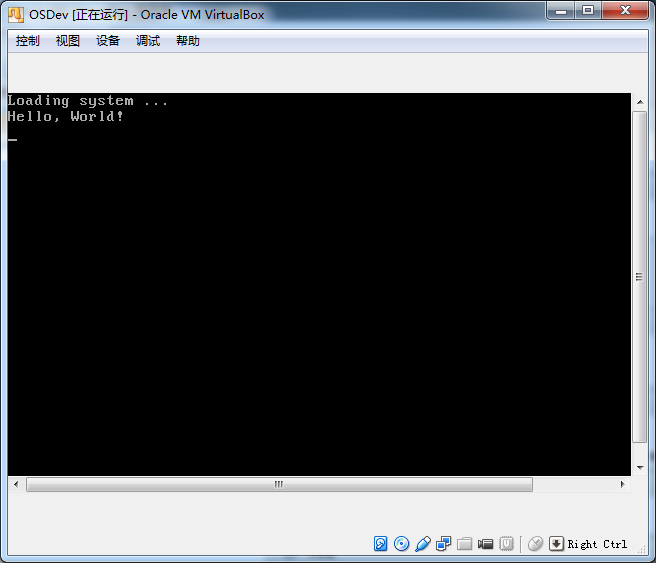
main: org 0x0100 jmp start
stack: db 256 dup(0) tos: dw 0
start: mov ax, cs mov ds, ax mov ss, ax mov sp, tos mov ax, end mov bx, main sub ax, bx mov bx, 2 div bx cmp dx, 0 je next_step inc ax next_step: mov bx, ax mov si, 0 mov di, 0 .0: cmp di, 0 je .1 mov ax, 0x0000 push ax call print_char pop ax
.1: mov dx, cs:[si] mov ch, 0x04 mov cl, 0x04 .2: mov ax, 0 rol dx, cl mov al, dl and al, 0x0f add al, 0x30 cmp al, 0x3a jl .3 add al, 0x07 .3: push ax call print_char pop ax dec ch cmp ch, 0 jne .2 inc di add si, 2 dec bx cmp bx, 0 jne .0 hlt
print_char: push bp push ax push bx mov bp, sp mov ax, [bp+8] mov ah, 0x0e mov bx, 0x0000 int 0x10
pop bx pop ax pop bp ret
end: dw 0
; You may customize this and other start-up templates; ; The location of this template is c:\emu8086\inc\0_com_template.txt
org 100h start: jmp main
stack: db 256 dup(0) code_len: dw 0 ; add your code here main: mov ax, cs mov ds, ax mov ss, ax mov sp, stack+256 mov ax, end mov bx, main sub ax, bx mov bx, 2 div bx cmp dx, 0 je next_step inc ax next_step: mov bx, code_len mov [bx], ax mov bx, main push ax push bx push cs call dump_memory pop ax pop bx pop ax hlt dump_memory: push bp push ax push bx push cx push dx push ds mov bp, sp mov ax, [bp+14] mov bx, [bp+16] mov cx, [bp+18] xor dx, dx mov ds, ax rotate_memory: mov ax, ds:[bx] push ax call write_hex pop ax add bx, 2 loop rotate_memory pop ax mov ds, ax pop dx pop cx pop bx pop ax pop bp ret write_hex: push bp push ax push bx push cx push dx mov bp, sp mov bx, [bp+12] xor ax, ax xor cx, cx mov ch, 4 rotate: mov cl, 4 rol bx, cl mov al, bl and al, 0x0f add al, 0x30 cmp al, 0x3a jl printit add al, 0x07 printit: mov dl, al push dx call write_char pop dx dec ch jnz rotate mov dx, 0x0000 push dx call write_char pop dx pop dx pop cx pop bx pop ax pop bp ret write_char: push bp push ax push dx mov bp, sp mov ah, 0x02 mov dx, [bp+8] int 0x21 pop dx pop ax pop bp ret end: db 0x55,0xaa
; You may customize this and other start-up templates; ; The location of this template is c:\emu8086\inc\0_com_template.txt
org 100h jmp start
data: dw 32 dup(0) stack: dw 256 dup(0)
start: ; add your code here mov ax, cs mov ds, ax mov bx, data mov ss, ax mov sp, stack+31 push 0x0001 push 0x0002 push 0x0003 call test_proc hlt test_proc: push bp mov bp, sp mov ax, [bp+8] push ax call write_char mov ax, [bp+6] push ax call write_char mov ax, [bp+4] push ax call write_char pop bp ret 6 write_char: push bp mov bp, sp mov ax, [bp+4] mov dl, al add dl, 0x30 cmp dl, 0x3a jl printit add dl, 0x07 printit: mov ah, 0x02 int 0x21 pop bp ret 2
org 100h
; add your code here mov ax, cs mov ds, ax mov ss, ax mov ax, 0x0000 mov bx, 0x106f mov ch, 0x04 rotate: mov cl, 0x04 rol bx, cl mov al, bl and al, 0x0f add al, 0x30 cmp al, 0x3a jl printit add al, 0x07 printit: mov dl, al mov ah, 0x02 int 0x21 dec ch jnz rotate
ret
; You may customize this and other start-up templates; ; The location of this template is c:\emu8086\inc\0_com_template.txt
org 100h
; add your code here mov ax, cs mov ds, ax mov ss, ax mov sp, stack + 256 - 1 mov si, 0 push 0x105e call write_bin pop ax call write_space2 push 0x0002 push 0x78fd call write_num pop ax pop ax call write_space2 push 0x0008 push 0x78fd call write_num pop ax pop ax call write_space2 push 0x000A push 0x78fd call write_num pop ax pop ax call write_space2 push 0x0010 push 0x78fd call write_num pop ax pop ax ret
write_space2: mov cx, 2 loop_write_char: call write_space loop loop_write_char ret
write_space: push 0x0000 call write_char pop ax ret write_char: push ax push bx push cx push dx mov bp, sp mov ah, 0x0e mov bx, ss:[bp+10] ; parameter 1 mov al, bl mov bx, 0x000c int 0x10 pop dx pop cx pop bx pop ax ret
write_bin: mov bp, sp mov ax, ss:[bp+2] ; parameter 1 mov cx, 16 s: xor dx, dx rcl ax, 1 adc dl, 0x30 push dx call write_char pop dx loop s ret write_num: mov bp, sp mov ax, ss:[bp+2] ; parameter 1 mov bx, ss:[bp+4] ; parameter 2 mov cx, 0 loop_num_div: mov dx, 0 div bx push dx inc cx cmp ax, 0 jne loop_num_div loop_num_disp: pop dx add dl, 0x30 cmp dl, 0x3A jl disp_char add dl, 0x07
disp_char: push dx call write_char pop dx loop loop_num_disp ret stack: dw 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 dw 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 dw 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 dw 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 dw 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 dw 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 dw 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 dw 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 end_flag: db 0x11
org 100h
; add your code here
mov ax, cs mov ds, ax mov ax, 0xb800 mov es, ax xor ax, ax xor bx, bx xor cx, cx xor dx, dx mov ax, 0x105e ;除数 mov bx, 10 ;被除数
s: mov dx, 0 ;清空余数 div bx ;除以被除数 push dx ;把余数压栈 inc cx ;循环次数加1 cmp ax, 0 ;商不为0继续除 jne s mov bx, 0 p1: pop dx add dl, 0x30 mov es:[bx], dl inc bx mov es:[bx], 0x0c inc bx loop p1 ret
org 100h
; add your code here
mov ax, cs mov ds, ax mov ax, 0xb800 mov es, ax xor ax, ax xor bx, bx xor dx, dx mov dl, 0x2f mov al, dl shr al, 4 add al, 0x30 cmp al, 0x3a jl p1 add al, 0x07
p1: mov es:[bx], al inc bx mov es:[bx], 0x0c inc bx mov al, dl and al, 0x0f add al, 0x30 cmp al, 0x3a jl p2 add al, 0x07 p2: mov es:[bx], al inc bx mov es:[bx], 0x0c inc bx ret
|