The intent of the Builder design pattern is to separate the construction of a complex object from its representation. By doing so, the same construction process can create different representations.
struct:
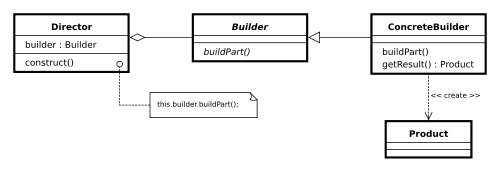
- Builder
- Abstract interface for creating objects (product).
- Concrete Builder
- Provides implementation for Builder. It is an object able to construct other objects. Constructs and assembles parts to build the objects.
1 #include <string>
2 #include <iostream>
3 using namespace std;
4
5 // "Product"
6 class Pizza {
7 public:
8 void dough(const string& dough) {
9 dough_ = dough;
10 }
11
12 void sauce(const string& sauce) {
13 sauce_ = sauce;
14 }
15
16 void topping(const string& topping) {
17 topping_ = topping;
18 }
19
20 void open() const {
21 cout << "Pizza with " << dough_ << " dough, " << sauce_ << " sauce and "
22 << topping_ << " topping. Mmm." << endl;
23 }
24
25 private:
26 string dough_;
27 string sauce_;
28 string topping_;
29 };
30
31 // "Abstract Builder"
32 class PizzaBuilder {
33 public:
34 const Pizza& pizza() {
35 return pizza_;
36 }
37
38 virtual void buildDough() = 0;
39 virtual void buildSauce() = 0;
40 virtual void buildTopping() = 0;
41
42 protected:
43 Pizza pizza_;
44 };
45
46 //----------------------------------------------------------------
47
48 class HawaiianPizzaBuilder : public PizzaBuilder {
49 public:
50 void buildDough() {
51 pizza_.dough("cross");
52 }
53
54 void buildSauce() {
55 pizza_.sauce("mild");
56 }
57
58 void buildTopping() {
59 pizza_.topping("ham+pineapple");
60 }
61 };
62
63 class SpicyPizzaBuilder : public PizzaBuilder {
64 public:
65 void buildDough() {
66 pizza_.dough("pan baked");
67 }
68
69 void buildSauce() {
70 pizza_.sauce("hot");
71 }
72
73 void buildTopping() {
74 pizza_.topping("pepperoni+salami");
75 }
76 };
77
78 //----------------------------------------------------------------
79
80 class Cook {
81 public:
82 Cook()
83 : pizzaBuilder_(nullptr)
84 { }
85
86 ~Cook() {
87 if (pizzaBuilder_)
88 delete pizzaBuilder_;
89 }
90
91 void pizzaBuilder(PizzaBuilder* pizzaBuilder) {
92 if (pizzaBuilder_)
93 delete pizzaBuilder_;
94
95 pizzaBuilder_ = pizzaBuilder;
96 }
97
98 const Pizza& getPizza() {
99 return pizzaBuilder_->pizza();
100 }
101
102 void constructPizza() {
103 pizzaBuilder_->buildDough();
104 pizzaBuilder_->buildSauce();
105 pizzaBuilder_->buildTopping();
106 }
107
108 private:
109 PizzaBuilder* pizzaBuilder_;
110 };
111
112 int main() {
113 Cook cook;
114 cook.pizzaBuilder(new HawaiianPizzaBuilder);
115 cook.constructPizza();
116
117 Pizza hawaiian = cook.getPizza();
118 hawaiian.open();
119
120 cook.pizzaBuilder(new SpicyPizzaBuilder);
121 cook.constructPizza();
122
123 Pizza spicy = cook.getPizza();
124 spicy.open();
125 }
posted on 2012-11-13 17:44
老马驿站 阅读(347)
评论(0) 编辑 收藏 引用 所属分类:
Design pattern