Posted on 2012-07-08 16:56
linzheng 阅读(371)
评论(0) 编辑 收藏 引用
下面使用VS2012来编写了自定义数组的程序:
程序目录如下:
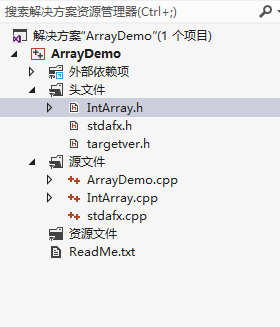
IntArray.h
#include "stdafx.h"
#include <iostream>
using namespace std;

class IntArray;
ostream& operator<<( ostream &, IntArray& );//自定义输出操作符


class IntArray
{
public:
//构造函数

IntArray( int sz = DefaultArraySize )
{ init( 0, sz ); }

IntArray( const int *ar, int sz )
{ init( ar, sz ); }

IntArray( const IntArray &iA )
{ init( iA._ia, iA._size ); }
//析构函数 每个类对象在被程序,最后一次使用之后它的析构函数就会被自动调用

virtual ~IntArray()
{ delete[] _ia; }
//赋值操作符
IntArray& operator=( const IntArray& );
//访问数组的大小

int size() const
{ return _size; }
//相等与不相等操作符
bool operator==( const IntArray& ) const;
bool operator!=( const IntArray& ) const;


virtual int& operator[]( int ix ) const
{ return _ia[ix]; }

ostream &print( ostream& os = cout ) const;
void grow();
virtual void sort( int, int );
virtual int find( int ) const;

virtual int min() const;
virtual int max() const;

protected:
void init( const int*, int );
void swap( int, int );

// 内部数据
static const int DefaultArraySize;//数组的默认大小
int _size;//数组的实际大小
int *_ia;//数组的指针
};
IntArray.cpp
#include "stdafx.h"
#include <assert.h>
#include "IntArray.h"
using namespace std;
const int IntArray::DefaultArraySize = 12;
//初始化方法
void IntArray::init( const int *array, int sz )
{
if ( ! array ) { _size = 0; _ia = 0; }
if ( sz < 1 ) sz = 1;
//初始化数据
_size = sz;
_ia = new int[ _size ];
//初始化内存
for ( int ix = 0; ix < _size; ++ix )
_ia[ ix ] = array[ ix ];
}
IntArray& IntArray::operator=( const IntArray &iA )
{
//如果是相同的指针,则返回本身的指针
if ( this == &iA )
return *this;
//否则,释放原来指针的内存,并重新初始化,返回当前的指针
delete[] _ia;
init( iA._ia, iA._size );
return *this;
}
ostream& operator<<( ostream &os, IntArray &ar )
{
return ar.print( os );
}
ostream& IntArray::print( ostream &os ) const
{
const int lineLength = 12;
os << "( " << _size << " )< ";
for ( int ix = 0; ix < _size; ++ix )
{
if ( ix % lineLength == 0 && ix )
os << "\n\t";
os << _ia[ ix ];
if ( ix % lineLength != lineLength-1 && ix != _size-1 )
os << ", ";
}
os << " >\n";
return os;
}
//增加一半的数组元素
void IntArray::grow()
{
int *oldia = _ia;
int oldSize = _size;
_size = oldSize + oldSize/2 + 1;
_ia = new int[_size];
int ix;
for ( ix = 0; ix < oldSize; ++ix)
_ia[ix] = oldia[ix];
for ( ; ix < _size; ++ix )
_ia[ix] = 0;
//释放掉临时的指针
delete[] oldia;
}
//最小值
int IntArray::min() const
{
assert( _ia != 0 );
int min_val = _ia[0];
for ( int ix = 1; ix < _size; ++ix )
if ( _ia[ix] < min_val )
min_val = _ia[ix];
return min_val;
}
//最大值
int IntArray::max() const
{
assert( _ia != 0 );
int max_val = _ia[0];
for ( int ix = 1; ix < _size; ++ix )
if ( max_val < _ia[ix] )
max_val = _ia[ix];
return max_val;
}
//查找
int IntArray::find( int val ) const
{
for ( int ix = 0; ix < _size; ++ix )
if ( val == _ia[ix] )
return ix;
return -1;
}
//交换值
void IntArray::swap( int i, int j )
{
int tmp = _ia[i];
_ia[i] = _ia[j];
_ia[j] = tmp;
}
//排序
void IntArray::sort( int low, int high )
{
if ( low >= high ) return;
int lo = low;
int hi = high + 1;
int elem = _ia[low];
for ( ;; ) {
while ( _ia[++lo] < elem ) ;
while ( _ia[--hi] > elem ) ;
if ( lo < hi )
swap( lo,hi );
else break;
}
swap( low, hi );
sort( low, hi-1 );
sort( hi+1, high );
}
// ArrayDemo.cpp : 定义控制台应用程序的入口点。
//
#include "stdafx.h"
#include "IntArray.h"
using namespace std;
int _tmain(int argc, _TCHAR* argv[])
{
int array[ 4 ] = { 0, 1, 2, 3 };
IntArray ia1( array, 4 );
ia1.grow();
ia1.print();
int search_value;
cout << "please enter search value: ";
cin >> search_value;
return 0;
}
运行的效果:
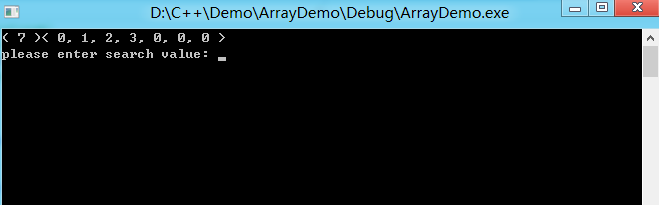