本篇是
创建游戏内核(13)的续篇,其中涉及到的网格绘制知识请参阅D3D中网格模型的运用。
使用.X样式三维动画
三维动画与二维动画相比较是一个全新的处理过程。不用再奢侈地绘制图像,然后按顺序显示来创建动画。在三维世界中,一个对象可以从任何方向进行观察。
基本上,三维动画改变那些在运行期间用于为网格模型指明方向的框架变换矩阵,进而产生出在框架中移动的各式各样的网格模型,这个运动的网格模型就是动画。可以使用任何方式平移、旋转、甚至缩放网格模型。
在处理蒙皮网格模型时,框架变换的使用只不过是使网格模型产生动画的一种方法。因为一个蒙皮网格模型就是一个单一的网格模型(并非由多个网格模型组成),需要改变框架比便进行顶点的变形。修改框架变换矩阵最简单的方法就是使用一种称之为关键桢(key
frame)的技术。
关键桢技术
在计算机动画中,关键桢描述了这样一种技术,它使用两个完整的、单独的方位(关键桢),以及两者之间基于某一系数(例如时间)的插值。另一方面,通过使用两个不同框架的方位(每个方位称之为一个关键点key),就可以计算出那些关键点任何位置以及任何时间所在的方位。
如下所示,框架的方位,从开始时的方位到结束时的方位,随时间变化进行插值计算。
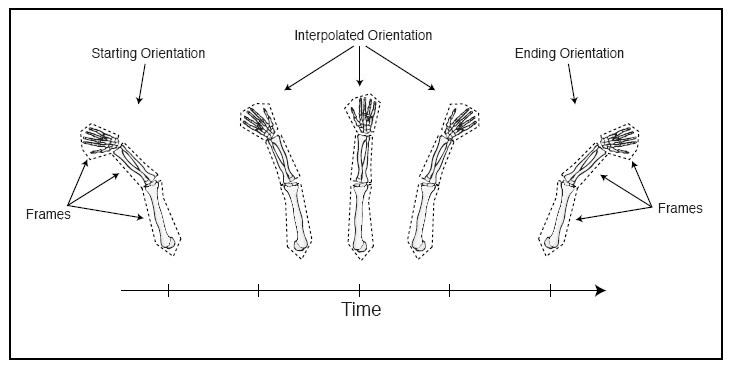
关键帧的效率非常高,它确保了动画能够以相同的速度运行在所有的系统中。运行较慢的计算机会减少帧数(以动画的跳跃为代价),而较快的计算机能够产生出更多的帧,从而提供更平滑的动画。
只需要在那些帧之间进行插值计算便可以产生平滑的动画,插值(interpolating)是一种计算两个数值之间,随时间变化的过渡数值的方法。这里使用的关键桢形式是矩阵关键桢(matrix key
framing),因为使用的是D3DX矩阵对象,所以使用这种格式的关键桢会十分容易。比如说,有两个矩阵mat1和mat2,分别表示开始和结束的矩阵,他们之间的时间距离表示为time_length,同时当前的时间表示为time(其范围从0到length),计算插值矩阵,就像下面代码所示:
D3DXMATRIX mat1, mat2;
DWORD time_length, time;
D3DXMATRIX mat_inter; // 最终的插值矩阵
// 计算插值矩阵
mat_inter = mat1 + ((mat2 - mat1) / time_length) * time;
.X中的动画
微软公司在.X文件中提供了动画数据,该动画数据位于一个特定数据对象的集合里,同时,也可以使用与加载蒙皮网格模型相同的技术去加载那些动画对象的数据。特定的数据对象包含了在关键帧技术中所使用到的各种各样的关键点,每个关键点代表了一种变换:旋转、缩放、平移。为了使之更加容易,可以指定同时组合了所有变换的矩阵关键点。
每个关键点都有一个与之相关联的激活时间。换句话说,在time=0的旋转关键点意味着在时间为0时,关键点中的旋转数值被使用。而第二个旋转关键点在time=200时激活。当计时继续时,位于第一个和第二个旋转关键点之间某个位置的旋转插值被计算出来。当时间到达200时,旋转数值与第二个旋转关键点相等。这种形式的插值计算可以被运用到所有的关键点类型中。
动画以集合的形式出现,同时每个集合被分配特定的框架,可以将多个关键点分配给相同的集合,以便使多个动画关键点能够同时影响到框架。举例来说,一个框架可以同时被旋转关键点和平移关键点的集合来修改,以便同时旋转并平移框架。
使用OBJECT绘制对象
要绘制网格,必须在网格定义和显示器之间搭设一道桥梁。为什么不使用MESH对象处理渲染呢?原因在于内存使用(memory
usage),如果要反复使用相同的网格,该怎么办?解决的办法就是使用OBJECT对象。
首先定义表示插值旋转变换、平移变换、缩放、变换矩阵的数据结构:
struct S_ROTATE_KEY
{
DWORD time;
D3DXQUATERNION quat;
};
struct S_POSITION_KEY
{
DWORD time;
D3DXVECTOR3 pos;
D3DXVECTOR3 pos_inter;
};
struct S_SCALE_KEY
{
DWORD time;
D3DXVECTOR3 scale;
D3DXVECTOR3 scale_inter;
};
struct S_MATRIX_KEY
{
DWORD time;
D3DXMATRIX matrix;
D3DXMATRIX mat_inter;
};
其次定义一个叫做S_ANIATION的结构体来封装框架的变换矩阵。
struct S_ANIMATION
{
public:
char* m_name;
char* m_frame_name;
S_FRAME* m_frame;
BOOL m_is_loop;
BOOL m_is_linear;
DWORD m_num_position_keys;
S_POSITION_KEY* m_position_keys;
DWORD m_num_rotate_keys;
S_ROTATE_KEY* m_rotate_keys;
DWORD m_num_scale_keys;
S_SCALE_KEY* m_scale_keys;
DWORD m_num_matrix_keys;
S_MATRIX_KEY* m_matrix_keys;
S_ANIMATION* m_next;
//--------------------------------------------------------------------
// Constructor, initialize member data.
//--------------------------------------------------------------------
S_ANIMATION()
{
m_name = NULL;
m_frame_name = NULL;
m_frame = NULL;
m_is_loop = FALSE;
m_is_linear = TRUE;
m_num_position_keys = 0;
m_num_rotate_keys = 0;
m_num_scale_keys = 0;
m_num_matrix_keys = 0;
m_position_keys = NULL;
m_rotate_keys = NULL;
m_scale_keys = NULL;
m_matrix_keys = NULL;
m_next = NULL;
}
//--------------------------------------------------------------------
// Destructor, release resource.
//--------------------------------------------------------------------
~S_ANIMATION()
{
delete[] m_name; m_name = NULL;
delete[] m_frame_name; m_frame_name = NULL;
delete[] m_position_keys; m_position_keys = NULL;
delete[] m_rotate_keys; m_rotate_keys = NULL;
delete[] m_scale_keys; m_scale_keys = NULL;
delete[] m_matrix_keys; m_matrix_keys = NULL;
delete[] m_next; m_next = NULL;
}
//--------------------------------------------------------------------
// Update current frame's transformed matrix.
//--------------------------------------------------------------------
void Update(DWORD time, BOOL is_smooth)
{
unsigned long key_index;
DWORD time_diff, time_inter;
D3DXMATRIX matrix, mat_temp;
D3DXVECTOR3 vector;
D3DXQUATERNION quat;
if(m_frame == NULL)
return;
// Update rotation, scale, and position keys.
if(m_num_rotate_keys != 0 || m_num_scale_keys != 0 || m_num_position_keys != 0)
{
D3DXMatrixIdentity(&matrix);
// update rotation matrix
if(m_num_rotate_keys != 0 && m_rotate_keys != NULL)
{
// find the key that fits this time
key_index = 0;
for(unsigned long i = 0; i < m_num_rotate_keys; i++)
{
if(m_rotate_keys[i].time <= time)
key_index = i;
else
break;
}
// If it's the last key or non-smooth animation, then just set the key value.
if(key_index == m_num_rotate_keys - 1 || is_smooth == FALSE)
quat = m_rotate_keys[key_index].quat;
else
{
// calculate the time difference and interpolate time
time_diff = m_rotate_keys[key_index + 1].time - m_rotate_keys[key_index].time;
time_inter = time - m_rotate_keys[key_index].time;
// Get the quarternion value
//
// Interpolates between two quaternions, using spherical linear interpolation.
D3DXQuaternionSlerp(&quat, &m_rotate_keys[key_index].quat,
&m_rotate_keys[key_index+1].quat, (float)time_inter / time_diff);
}
// combine with the new matrix
// builds a rotation matrix from a quaternion.
D3DXMatrixRotationQuaternion(&mat_temp, &quat);
D3DXMatrixMultiply(&matrix, &matrix, &mat_temp);
}
// update scale matrix
if(m_num_scale_keys != 0 && m_scale_keys != NULL)
{
// find the key that fits this time
key_index = 0;
for(unsigned long i = 0; i < m_num_scale_keys; i++)
{
if(m_scale_keys[i].time <= time)
key_index = i;
else
break;
}
// If it's the last key or non-smooth animation, then just set the key value.
if(key_index == m_num_scale_keys - 1 || is_smooth == FALSE)
vector = m_scale_keys[key_index].scale;
else
{
// calculate the time difference and interpolate time
time_inter = time - m_scale_keys[key_index].time;
// get the interpolated vector value
vector = m_scale_keys[key_index].scale +
m_scale_keys[key_index].scale_inter * (float)time_inter;
}
// combine with the new matrix
D3DXMatrixScaling(&mat_temp, vector.x, vector.y, vector.z);
D3DXMatrixMultiply(&matrix, &matrix, &mat_temp);
}
// update translation matrix
if(m_num_position_keys != 0 && m_position_keys != NULL)
{
// find the key that fits this time
key_index = 0;
for(unsigned long i = 0; i < m_num_position_keys; i++)
{
if(m_position_keys[i].time <= time)
key_index = i;
else
break;
}
// If it's the last key or non-smooth animation, then just set the key value.
if(key_index == m_num_position_keys - 1 || is_smooth == FALSE)
vector = m_position_keys[key_index].pos;
else
{
// calculate the time difference and interpolate time
time_inter = time - m_position_keys[key_index].time;
// get the interpolated vector value
vector = m_position_keys[key_index].pos +
m_position_keys[key_index].pos_inter * (float)time_inter;
}
// combine with the new matrix
D3DXMatrixTranslation(&mat_temp, vector.x, vector.y, vector.z);
D3DXMatrixMultiply(&matrix, &matrix, &mat_temp);
}
// set the new matrix
m_frame->m_mat_transformed = matrix;
}
// update matrix keys
if(m_num_matrix_keys != 0 && m_matrix_keys != NULL)
{
// find the key that fits this time
key_index = 0;
for(unsigned long i = 0; i < m_num_matrix_keys; i++)
{
if(m_matrix_keys[i].time <= time)
key_index = i;
else
break;
}
// If it's the last key or non-smooth animation, then just set the matrix.
if(key_index == m_num_matrix_keys - 1 || is_smooth == FALSE)
m_frame->m_mat_transformed = m_matrix_keys[key_index].matrix;
else
{
// calculate the time difference and interpolated time
time_inter = time - m_matrix_keys[key_index].time;
// set the new interpolation matrix
matrix = m_matrix_keys[key_index].mat_inter * (float) time_inter;
m_frame->m_mat_transformed = m_matrix_keys[key_index].matrix + matrix;
}
}
}
};
接着再定义一个结构体S_ANIMATION_SET来封装动画集合。
struct S_ANIMATION_SET
{
public:
char* m_name;
S_ANIMATION* m_animation;
unsigned long m_time_length;
S_ANIMATION_SET* m_next;
//-----------------------------------------------------------------------------
// Constructor, initialize member data.
//-----------------------------------------------------------------------------
S_ANIMATION_SET()
{
m_name = NULL;
m_animation = NULL;
m_next = NULL;
m_time_length = 0;
}
//-----------------------------------------------------------------------------
// Destructor, release resource.
//-----------------------------------------------------------------------------
~S_ANIMATION_SET()
{
delete[] m_name; m_name = NULL;
delete m_animation; m_animation = NULL;
delete m_next; m_next = NULL;
}
//-----------------------------------------------------------------------------
// Find animation set in animation set link list which match specified name.
//-----------------------------------------------------------------------------
S_ANIMATION_SET* Find_Set(char* name)
{
// return first instance if name is NULL
if(name == NULL)
return this;
// compare names and return if exact match
if(m_name != NULL && !strcmp(name, m_name))
return this;
// search next in list
if(m_next != NULL)
{
S_ANIMATION_SET* anim_set;
if((anim_set = m_next->Find_Set(name)) != NULL)
return anim_set;
}
return NULL;
}
//-----------------------------------------------------------------------------
// Update all animations in current animation set.
//-----------------------------------------------------------------------------
void Update(DWORD time, BOOL is_smooth)
{
S_ANIMATION* anim = m_animation;
while(anim != NULL)
{
if(m_time_length == 0)
{
// If time length of the animation set is zero, just use first frame.
anim->Update(0, FALSE);
}
else if(time >= m_time_length && anim->m_is_loop == FALSE)
{
// If time beyonds max time length of the animation set and is not loop animation, use last frame.
anim->Update(time, FALSE);
}
else
{
// Now, update animation usually.
anim->Update(time % m_time_length, is_smooth);
}
anim = anim->m_next;
}
}
};
在定义好以上数据结构的方法的基础上,我们定义一个叫做OBJECT的类来封装网格对象的绘制:
class OBJECT
{
protected:
GRAPHICS* _graphics;
MESH* _mesh;
S_ANIMATION_SET* _animation_set;
WORLD_POSITION _pos;
BOOL _use_billboard;
unsigned long _start_time;
void _Update_Frame(S_FRAME* frame, D3DXMATRIX* matrix);
void _Draw_Frame(S_FRAME* frame);
public:
OBJECT();
~OBJECT();
BOOL Create(GRAPHICS* graphics, MESH* mesh = NULL);
void Free();
void Enable_Billboard(BOOL enable = TRUE);
void Attach_To_Object(OBJECT* object, char* frame_name = NULL);
void Move(float x_pos, float y_pos, float z_pos);
void Move_Rel(float x_add, float y_add, float z_add);
void Rotate(float x_rot, float y_rot, float z_rot);
void Rotate_Rel(float x_add, float y_add, float z_add);
void Scale(float x_scale, float y_scale, float z_scale);
void Scale_Rel(float x_add, float y_add, float z_add);
D3DXMATRIX* Get_Matrix();
float Get_X_Pos();
float Get_Y_Pos();
float Get_Z_Pos();
float Get_X_Rotation();
float Get_Y_Rotation();
float Get_Z_Rotation();
float Get_X_Scale();
float Get_Y_Scale();
float Get_Z_Scale();
BOOL Get_Bounds(float* min_x, float* min_y, float* min_z, float* max_x, float* max_y, float* max_z, float* radius);
void Set_Mesh(MESH* mesh);
MESH* Get_Mesh();
void Set_Animation(ANIMATION* animation, char* name = NULL, unsigned long start_time = 0);
char* Get_Animation_Name();
void Reset_Animation(unsigned long start_time = 0);
void Update_Animation(unsigned long time, BOOL is_smooth = TRUE);
BOOL Animation_Complete(unsigned long time);
void Update();
BOOL Render();
};
接着是类OBJECT的实现:
//-------------------------------------------------------------------
// Constructor, initialize member data.
//-------------------------------------------------------------------
OBJECT::OBJECT()
{
_graphics = NULL;
_mesh = NULL;
_animation_set = NULL;
}
//-------------------------------------------------------------------
// Destructor, release resource.
//-------------------------------------------------------------------
OBJECT::~OBJECT()
{
Free();
}
//-------------------------------------------------------------------
// Create an object with specified mesh object.
//-------------------------------------------------------------------
BOOL OBJECT::Create(GRAPHICS* graphics, MESH* mesh)
{
if((_graphics = graphics) == NULL)
return FALSE;
_mesh = mesh;
Move(0.0f, 0.0f, 0.0f);
Rotate(0.0f, 0.0f, 0.0f);
Scale(1.0f, 1.0f, 1.0f);
return TRUE;
}
//-------------------------------------------------------------------
// Release resource.
//-------------------------------------------------------------------
void OBJECT::Free()
{
_graphics = NULL;
_mesh = NULL;
_animation_set = NULL;
}
//-------------------------------------------------------------------
// Enable or disable billboard.
//-------------------------------------------------------------------
void OBJECT::Enable_Billboard(BOOL enable)
{
_pos.Enable_Billboard(enable);
}
//-------------------------------------------------------------------
// Attach another object's transformed matrix to current object.
//-------------------------------------------------------------------
void OBJECT::Attach_To_Object(OBJECT* object, char* frame_name)
{
if(object == NULL || object->_mesh == NULL)
{
_pos.Set_Combine_Matrix_1(NULL);
_pos.Set_Combine_Matrix_2(NULL);
}
else
{
S_FRAME* frame = object->_mesh->Get_Frame(frame_name);
if(frame == NULL)
{
_pos.Set_Combine_Matrix_1(NULL);
_pos.Set_Combine_Matrix_2(NULL);
}
else
{
_pos.Set_Combine_Matrix_1(&frame->m_mat_combined);
_pos.Set_Combine_Matrix_2(object->_pos.Get_Matrix());
}
}
}
//-------------------------------------------------------------------
// Move object to new world position with specified relative value.
//-------------------------------------------------------------------
void OBJECT::Move(float x_pos, float y_pos, float z_pos)
{
_pos.Move(x_pos, y_pos, z_pos);
}
//-------------------------------------------------------------------
// Move object to new world position which is specified by new relative
// value and current position.
//-------------------------------------------------------------------
void OBJECT::Move_Rel(float x_add, float y_add, float z_add)
{
_pos.Move_Rel(x_add, y_add, z_add);
}
//-------------------------------------------------------------------
// Rotate around x, y, z, axis with specified degree.
//-------------------------------------------------------------------
void OBJECT::Rotate(float x_rot, float y_rot, float z_rot)
{
_pos.Rotate(x_rot, y_rot, z_rot);
}
//-------------------------------------------------------------------
// Rotate around x, y, z, axis which is specified with new relative
// degree and current rotation value.
//-------------------------------------------------------------------
void OBJECT::Rotate_Rel(float x_add, float y_add, float z_add)
{
_pos.Rotate_Rel(x_add, y_add, z_add);
}
//-------------------------------------------------------------------
// Build scaling matrix.
//-------------------------------------------------------------------
void OBJECT::Scale(float x_scale, float y_scale, float z_scale)
{
_pos.Scale(x_scale, y_scale, z_scale);
}
//-------------------------------------------------------------------
// Build scaling matrix with specified value and current scaling value.
//-------------------------------------------------------------------
void OBJECT::Scale_Rel(float x_add, float y_add, float z_add)
{
_pos.Scale_Rel(x_add, y_add, z_add);
}
//-------------------------------------------------------------------
// Get current world transform matrix.
//-------------------------------------------------------------------
D3DXMATRIX* OBJECT::Get_Matrix()
{
return _pos.Get_Matrix();
}
//-------------------------------------------------------------------
// Set mesh to current object.
//-------------------------------------------------------------------
void OBJECT::Set_Mesh(MESH *mesh)
{
_mesh = mesh;
}
//-------------------------------------------------------------------
// Get current object's mesh.
//-------------------------------------------------------------------
MESH* OBJECT::Get_Mesh()
{
return _mesh;
}
//-------------------------------------------------------------------
// Set animation set with specified name and start time.
//-------------------------------------------------------------------
void OBJECT::Set_Animation(ANIMATION* animation, char* name, unsigned long start_time)
{
_start_time = start_time;
if(animation == NULL)
_animation_set = NULL;
else
_animation_set = animation->Get_Animation_Set(name);
}
//-------------------------------------------------------------------
// Get the name of animation set.
//-------------------------------------------------------------------
char* OBJECT::Get_Animation_Name()
{
if(_animation_set == NULL)
return NULL;
return _animation_set->m_name;
}
//-------------------------------------------------------------------
// Reset start time of animation set.
//-------------------------------------------------------------------
void OBJECT::Reset_Animation(unsigned long start_time)
{
_start_time = start_time;
}
//-------------------------------------------------------------------
// Get x coordinate of current obejct.
//-------------------------------------------------------------------
float OBJECT::Get_X_Pos()
{
return _pos.Get_X_Pos();
}
//-------------------------------------------------------------------
// Get y coordinate of current obejct.
//-------------------------------------------------------------------
float OBJECT::Get_Y_Pos()
{
return _pos.Get_Y_Pos();
}
//-------------------------------------------------------------------
// Get z coordinate of current obejct.
//-------------------------------------------------------------------
float OBJECT::Get_Z_Pos()
{
return _pos.Get_Z_Pos();
}
//-------------------------------------------------------------------
// Get current rotation value which rotate around x axis.
//-------------------------------------------------------------------
float OBJECT::Get_X_Rotation()
{
return _pos.Get_X_Rotation();
}
//-------------------------------------------------------------------
// Get current rotation value which rotate around y axis.
//-------------------------------------------------------------------
float OBJECT::Get_Y_Rotation()
{
return _pos.Get_Y_Rotation();
}
//-------------------------------------------------------------------
// Get current rotation value which rotate around z axis.
//-------------------------------------------------------------------
float OBJECT::Get_Z_Rotation()
{
return _pos.Get_Z_Rotation();
}
//-------------------------------------------------------------------
// Get current scale value which around x axis.
//-------------------------------------------------------------------
float OBJECT::Get_X_Scale()
{
return _pos.Get_X_Scale();
}
//-------------------------------------------------------------------
// Get current scale value which around y axis.
//-------------------------------------------------------------------
float OBJECT::Get_Y_Scale()
{
return _pos.Get_Y_Scale();
}
//-------------------------------------------------------------------
// Get current scale value which around z axis.
//-------------------------------------------------------------------
float OBJECT::Get_Z_Scale()
{
return _pos.Get_Z_Scale();
}
//-------------------------------------------------------------------
// Get bound coordinate and radius after scale.
//-------------------------------------------------------------------
BOOL OBJECT::Get_Bounds(float *min_x, float *min_y, float *min_z, float *max_x, float *max_y, float *max_z,
float *radius)
{
if(_mesh == NULL)
return FALSE;
// Get bound box coordiante and radius.
_mesh->Get_Bounds(min_x, min_y, min_z, max_x, max_y, max_z, radius);
// scale bounds
float x_scale = _pos.Get_X_Scale();
float y_scale = _pos.Get_Y_Scale();
float z_scale = _pos.Get_Z_Scale();
if(min_x != NULL) *min_x *= x_scale;
if(min_y != NULL) *min_y *= y_scale;
if(min_z != NULL) *min_z *= z_scale;
if(max_x != NULL) *max_x *= x_scale;
if(max_y != NULL) *max_y *= y_scale;
if(max_z != NULL) *max_z *= z_scale;
if(radius != NULL)
{
float length = (float) sqrt(x_scale * x_scale + y_scale * y_scale + z_scale * z_scale);
(*radius) *= length;
}
return TRUE;
}
//-------------------------------------------------------------------
// Update world tranform matrix.
//-------------------------------------------------------------------
void OBJECT::Update()
{
_pos.Update(_graphics);
}
//-----------------------------------------------------------------------------
// Update all animations in current animation set.
//-----------------------------------------------------------------------------
void OBJECT::Update_Animation(unsigned long time, BOOL is_smooth)
{
if(_animation_set)
{
// reset all frames's transformed matrices to original matrices
_mesh->Get_Root_Frame()->Reset_Matrices();
// update all animations in current animation set
_animation_set->Update(time - _start_time, is_smooth);
}
}
//-----------------------------------------------------------------------------
// Judge whether animation has completed.
//-----------------------------------------------------------------------------
BOOL OBJECT::Animation_Complete(unsigned long time)
{
if(_animation_set == NULL)
return TRUE;
if((time - _start_time) >= _animation_set->m_time_length)
return TRUE;
return FALSE;
}
//-----------------------------------------------------------------------------
// Render all meshes in this object.
//-----------------------------------------------------------------------------
BOOL OBJECT::Render()
{
D3DXMATRIX matrix;
// error checking
if(_graphics == NULL || _mesh == NULL || _mesh->Get_Root_Frame() == NULL || _mesh->Get_Root_Mesh() == NULL)
return FALSE;
// update the object matrix
Update();
// update the frame matrices
D3DXMatrixIdentity(&matrix);
_Update_Frame(_mesh->Get_Root_Frame(), &matrix);
// copy frame matrices to bone matrices
_mesh->Get_Root_Mesh()->Copy_Frame_To_Bone_Matrices();
// draw all frame meshes
_Draw_Frame(_mesh->Get_Root_Frame());
return TRUE;
}
//-----------------------------------------------------------------------------
// Update transformation matrix of all frames, call recursively.
//-----------------------------------------------------------------------------
void OBJECT::_Update_Frame(S_FRAME *frame, D3DXMATRIX *matrix)
{
// return if no more frames
if(frame == NULL)
return;
// calculate frame matrix based on animation or not
if(_animation_set == NULL)
D3DXMatrixMultiply(&frame->m_mat_combined, &frame->m_mat_original, matrix);
else
D3DXMatrixMultiply(&frame->m_mat_combined, &frame->m_mat_transformed, matrix);
// update child frames
_Update_Frame(frame->m_child, &frame->m_mat_combined);
// update sibling frames
_Update_Frame(frame->m_sibling, matrix);
}
//-----------------------------------------------------------------------------
// Draw all meshes in frames which under current specified frame, call recursively.
//-----------------------------------------------------------------------------
void OBJECT::_Draw_Frame(S_FRAME *frame)
{
S_MESH_LIST* list;
S_MESH* mesh;
D3DXMATRIX mat_world;
if(frame == NULL)
return;
if((list = frame->m_mesh_list) != NULL)
{
// draw all meshes in this frame
while(list != NULL)
{
// see if there's a mesh to draw
if((mesh = list->m_mesh) != NULL)
{
// generate the mesh if using bones and set world matrix
if(mesh->m_num_bones && mesh->m_skin_mesh)
{
void* src_ptr;
void* dest_ptr;
// lock the source and destination vertex buffers
mesh->m_mesh->LockVertexBuffer(D3DLOCK_READONLY, (void**) &src_ptr);
mesh->m_skin_mesh->LockVertexBuffer(0, (void**) &dest_ptr);
// perfrom skinned mesh update
mesh->m_skin_info->UpdateSkinnedMesh(mesh->m_matrices, NULL, src_ptr, dest_ptr);
// unlock vertex buffers
mesh->m_skin_mesh->UnlockVertexBuffer();
mesh->m_mesh->UnlockVertexBuffer();
// set object world transformation
_graphics->Get_Device_COM()->SetTransform(D3DTS_WORLD, _pos.Get_Matrix());
}
else
{
// set the world transformation matrix for this frame
D3DXMatrixMultiply(&mat_world, &frame->m_mat_combined, _pos.Get_Matrix());
_graphics->Get_Device_COM()->SetTransform(D3DTS_WORLD, &mat_world);
}
// loop through materials and draw the mesh
for(DWORD i = 0; i < mesh->m_num_materials; i++)
{
// don't draw materials with no alpha (0.0)
if(mesh->m_materials[i].Diffuse.a != 0.0f)
{
_graphics->Get_Device_COM()->SetMaterial(&mesh->m_materials[i]);
_graphics->Get_Device_COM()->SetTexture(0, mesh->m_textures[i]);
// enabled alpha blending based on material alpha
if(mesh->m_materials[i].Diffuse.a != 1.0f)
_graphics->Enable_Alpha_Blending(TRUE, D3DBLEND_SRCCOLOR, D3DBLEND_ONE);
// draw mesh or skinned mehs
if(mesh->m_skin_mesh != NULL)
mesh->m_skin_mesh->DrawSubset(i);
else
mesh->m_mesh->DrawSubset(i);
// disable alpha blending based on material alpha
if(mesh->m_materials[i].Diffuse.a != 1.0f)
_graphics->Enable_Alpha_Blending(FALSE);
}
}
}
// next mesh in lsit
list = list->m_next;
}
}
// draw child frames and sibling frames
_Draw_Frame(frame->m_child);
_Draw_Frame(frame->m_sibling);
}
其中涉及到网格动画组件类ANIMATION,其简要定义如下:
class ANIMATION
{
protected:
long _num_animations;
S_ANIMATION_SET* _animation_set;
public:
ANIMATION();
~ANIMATION();
S_ANIMATION_SET* Get_Animation_Set(char* name = NULL);
void Free();
};
//-------------------------------------------------------------------
// Constructor, initialize member data.
//-------------------------------------------------------------------
ANIMATION::ANIMATION()
{
_num_animations = 0;
_animation_set = NULL;
}
//-------------------------------------------------------------------
// Destructor, free resource.
//-------------------------------------------------------------------
ANIMATION::~ANIMATION()
{
Free();
}
//-------------------------------------------------------------------
// Free resource.
//-------------------------------------------------------------------
void ANIMATION::Free()
{
delete _animation_set;
_animation_set = NULL;
_num_animations = 0;
}
//-------------------------------------------------------------------
// Get animation set which match specified name.
//-------------------------------------------------------------------
S_ANIMATION_SET* ANIMATION::Get_Animation_Set(char* name)
{
if(_animation_set == NULL)
return NULL;
return _animation_set->Find_Set(name);
}
接着,我们编写测试代码来测试类OBJECT:
点击下载源码和工程
/*****************************************************************************
PURPOSE:
Test for class OBJECT.
*****************************************************************************/
#include "Core_Global.h"
#pragma warning(disable : 4996)
//===========================================================================
// Defines class APP which public inherits from class APPLICATION.
//===========================================================================
class APP : public APPLICATION
{
private:
GRAPHICS _graphics;
MESH _mesh;
OBJECT _object;
public:
BOOL Init();
BOOL Shutdown();
BOOL Frame();
};
//-----------------------------------------------------------------------------
// Initialize graphics, set display mode, set vertex buffer, load texture file.
//-----------------------------------------------------------------------------
BOOL APP::Init()
{
D3DXMATRIX mat_view;
// initialize graphics
if (! _graphics.Init())
return FALSE;
// set display mode for graphics
if(! _graphics.Set_Mode(Get_Hwnd(), TRUE, FALSE, 400, 400, 16))
return FALSE;
// disable D3D lighting
_graphics.Enable_Lighting(FALSE);
// set perspective projection transform matrix.
_graphics.Set_Perspective(D3DX_PI/4.0f, 1.33333f, 1.0f, 1000.0f);
// create and set the view matrix
D3DXMatrixLookAtLH(&mat_view,
&D3DXVECTOR3(0.0, 50.0, -150.0),
&D3DXVECTOR3(0.0, 50.0, 0.0),
&D3DXVECTOR3(0.0, 1.0, 0.0));
_graphics.Get_Device_COM()->SetTransform(D3DTS_VIEW, &mat_view);
// load mesh
if(! _mesh.Load(&_graphics, "warrior.x"))
return FALSE;
// create object to draw
if(! _object.Create(&_graphics, &_mesh))
return FALSE;
return TRUE;
}
//-----------------------------------------------------------------------------
// Release all d3d resource.
//-----------------------------------------------------------------------------
BOOL APP::Shutdown()
{
return TRUE;
}
//-----------------------------------------------------------------------------
// Render a frame.
//-----------------------------------------------------------------------------
BOOL APP::Frame()
{
D3DXMATRIX mat_world;
// clear display with specified color
_graphics.Clear_Display(D3DCOLOR_RGBA(0, 128, 0, 255));
// begin scene
if(_graphics.Begin_Scene())
{
// rotate object along x-axis, y-axis.
_object.Rotate((float) (timeGetTime() / 2000.0), (float) (timeGetTime() / 1000.0), 0);
// draw object
_object.Render();
// end the scene
_graphics.End_Scene();
}
// display video buffer
_graphics.Display();
return TRUE;
}
int PASCAL WinMain(HINSTANCE inst, HINSTANCE, LPSTR cmd_line, int cmd_show)
{
APP app;
return app.Run();
}
运行截图:
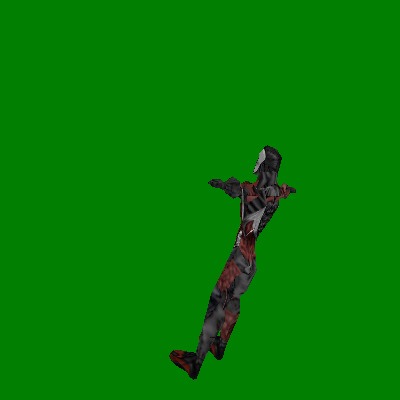