本篇是创建游戏内核(13)【OO改良版】的续篇,关于该内核的细节说明请参阅创建游戏内核(14)。
下载源码和工程
接口:
//================================================================================
// Defines for structures.
//================================================================================
typedef struct ROTATE_KEY
{
DWORD time;
D3DXQUATERNION quat;
} *ROTATE_KEY_PTR;
typedef struct POSITION_KEY
{
DWORD time;
D3DXVECTOR3 pos;
D3DXVECTOR3 pos_inter;
} *POSITION_KEY_PTR;
typedef struct SCALE_KEY
{
DWORD time;
D3DXVECTOR3 scale;
D3DXVECTOR3 scale_inter;
} *SCALE_KEY_PTR;
typedef struct MATRIX_KEY
{
DWORD time;
D3DXMATRIX matrix;
D3DXMATRIX mat_inter;
} *MATRIX_KEY_PTR;
//================================================================================
// Defines for structure ANIM_INFO.
//================================================================================
typedef class ANIM_INFO
{
public:
ANIM_INFO();
~ANIM_INFO();
void update_transform_matrix(DWORD time, BOOL is_smooth);
public:
char* m_name;
char* m_frame_name;
FRAME_INFO_PTR m_frame_info;
BOOL m_is_loop;
BOOL m_is_linear;
DWORD m_num_position_keys;
POSITION_KEY_PTR m_position_keys;
DWORD m_num_rotate_keys;
ROTATE_KEY_PTR m_rotate_keys;
DWORD m_num_scale_keys;
SCALE_KEY_PTR m_scale_keys;
DWORD m_num_matrix_keys;
MATRIX_KEY_PTR m_matrix_keys;
ANIM_INFO* m_next;
} *ANIM_INFO_PTR;
//================================================================================
// Defines for structure ANIM_INFO_SET.
//================================================================================
typedef class ANIM_INFO_SET
{
public:
ANIM_INFO_SET();
~ANIM_INFO_SET();
ANIM_INFO_SET* find_anim_info_set(const char* name);
void update_transform_matrix(DWORD time, BOOL is_smooth);
public:
char* m_name;
ANIM_INFO_PTR m_anim_info;
ulong m_time_length;
ANIM_INFO_SET* m_next;
} *ANIM_INFO_SET_PTR;
//================================================================================
// Defines for class OBJECT.
//================================================================================
typedef class OBJECT
{
public:
OBJECT();
BOOL create(MESH_PTR mesh);
void free();
void enable_billboard(BOOL enable);
void attach_frame_matrix_to_object(OBJECT* object, const char* frame_name);
void move(float x_pos, float y_pos, float z_pos);
void move_rel(float x_add, float y_add, float z_add);
void rotate(float x_rot, float y_rot, float z_rot);
void rotate_rel(float x_add, float y_add, float z_add);
void scale(float x_scale, float y_scale, float z_scale);
void scale_rel(float x_add, float y_add, float z_add);
LPD3DXMATRIX get_matrix();
float get_x_pos();
float get_y_pos();
float get_z_pos();
float get_x_rotation();
float get_y_rotation();
float get_z_rotation();
float get_x_scale();
float get_y_scale();
float get_z_scale();
BOOL get_bounds(float* min_x, float* min_y, float* min_z,
float* max_x, float* max_y, float* max_z,
float* radius);
void set_mesh(MESH_PTR mesh);
MESH_PTR get_mesh();
void set_anim_info_set(ANIMATION* animation, const char* name, ulong start_time);
char* get_anim_info_set_name();
void reset_anim_time(ulong start_time);
void update_anim_info_set(ulong time, BOOL is_smooth);
BOOL anim_complete(ulong time);
void update_world_pos();
BOOL render();
private:
MESH_PTR m_mesh;
ANIM_INFO_SET_PTR m_anim_info_set;
WORLD_POSITION m_world_pos;
ulong m_start_time;
void _update_frame_combined_matrix(FRAME_INFO_PTR frame_info, LPD3DXMATRIX matrix);
void _draw_frame(FRAME_INFO_PTR frame);
} *OBJECT_PTR;
//================================================================================
// Defines for class ANIMATION.
//================================================================================
typedef class ANIMATION
{
public:
ANIM_INFO_SET_PTR get_anim_info_set(const char* name)
{
if(m_anim_info_set == NULL)
return NULL;
return m_anim_info_set->find_anim_info_set(name);
}
private:
long m_num_anim;
ANIM_INFO_SET_PTR m_anim_info_set;
} *ANIMATION_PTR;
实现:
//--------------------------------------------------------------------
// Constructor, initialize member data.
//--------------------------------------------------------------------
ANIM_INFO::ANIM_INFO()
{
memset(this, 0, sizeof(*this));
m_is_linear = TRUE;
}
//--------------------------------------------------------------------
// Destructor, release resource.
//--------------------------------------------------------------------
ANIM_INFO::~ANIM_INFO()
{
delete[] m_name; m_name = NULL;
delete[] m_frame_name; m_frame_name = NULL;
delete[] m_position_keys; m_position_keys = NULL;
delete[] m_rotate_keys; m_rotate_keys = NULL;
delete[] m_scale_keys; m_scale_keys = NULL;
delete[] m_matrix_keys; m_matrix_keys = NULL;
delete m_next; m_next = NULL;
}
//--------------------------------------------------------------------
// update current frame's transformed matrix.
//--------------------------------------------------------------------
void ANIM_INFO::update_transform_matrix(DWORD time, BOOL is_smooth)
{
ulong _key_index;
DWORD _time_diff, _time_inter;
D3DXMATRIX _matrix, _mat_temp;
D3DXVECTOR3 _vector;
D3DXQUATERNION _quat;
if(m_frame_info == NULL)
return;
// update rotation, scale, and position keys.
if(m_num_rotate_keys != 0 || m_num_scale_keys != 0 || m_num_position_keys != 0)
{
D3DXMatrixIdentity(&_matrix);
// update rotation _matrix
if(m_num_rotate_keys != 0 && m_rotate_keys)
{
// find the key that fits this time
_key_index = 0;
for(ulong i = 0; i < m_num_rotate_keys; i++)
{
if(m_rotate_keys[i].time <= time)
_key_index = i;
else
break;
}
// If it's the last key or non-smooth animation, then just set the key value.
if(_key_index == m_num_rotate_keys - 1 || is_smooth == FALSE)
_quat = m_rotate_keys[_key_index].quat;
else
{
// calculate the time difference and interpolate time
_time_diff = m_rotate_keys[_key_index + 1].time - m_rotate_keys[_key_index].time;
_time_inter = time - m_rotate_keys[_key_index].time;
// Get the quarternion value
//
// Interpolates between two quaternions, using spherical linear interpolation.
D3DXQuaternionSlerp(&_quat, &m_rotate_keys[_key_index].quat,
&m_rotate_keys[_key_index+1].quat, (float)_time_inter / _time_diff);
}
// combine with the new _matrix
// builds a rotation _matrix from a quaternion.
D3DXMatrixRotationQuaternion(&_mat_temp, &_quat);
D3DXMatrixMultiply(&_matrix, &_matrix, &_mat_temp);
}
// update scale _matrix
if(m_num_scale_keys != 0 && m_scale_keys)
{
// find the key that fits this time
_key_index = 0;
for(ulong i = 0; i < m_num_scale_keys; i++)
{
if(m_scale_keys[i].time <= time)
_key_index = i;
else
break;
}
// If it's the last key or non-smooth animation, then just set the key value.
if(_key_index == m_num_scale_keys - 1 || is_smooth == FALSE)
_vector = m_scale_keys[_key_index].scale;
else
{
// calculate the time difference and interpolate time
_time_inter = time - m_scale_keys[_key_index].time;
// get the interpolated _vector value
_vector = m_scale_keys[_key_index].scale +
m_scale_keys[_key_index].scale_inter * (float)_time_inter;
}
// combine with the new _matrix
D3DXMatrixScaling(&_mat_temp, _vector.x, _vector.y, _vector.z);
D3DXMatrixMultiply(&_matrix, &_matrix, &_mat_temp);
}
// update translation _matrix
if(m_num_position_keys != 0 && m_position_keys)
{
// find the key that fits this time
_key_index = 0;
for(ulong i = 0; i < m_num_position_keys; i++)
{
if(m_position_keys[i].time <= time)
_key_index = i;
else
break;
}
// If it's the last key or non-smooth animation, then just set the key value.
if(_key_index == m_num_position_keys - 1 || is_smooth == FALSE)
_vector = m_position_keys[_key_index].pos;
else
{
// calculate the time difference and interpolate time
_time_inter = time - m_position_keys[_key_index].time;
// get the interpolated _vector value
_vector = m_position_keys[_key_index].pos +
m_position_keys[_key_index].pos_inter * (float)_time_inter;
}
// combine with the new _matrix
D3DXMatrixTranslation(&_mat_temp, _vector.x, _vector.y, _vector.z);
D3DXMatrixMultiply(&_matrix, &_matrix, &_mat_temp);
}
// set the new _matrix
m_frame_info->m_mat_transformed = _matrix;
}
// update _matrix keys
if(m_num_matrix_keys != 0 && m_matrix_keys)
{
// find the key that fits this time
_key_index = 0;
for(ulong i = 0; i < m_num_matrix_keys; i++)
{
if(m_matrix_keys[i].time <= time)
_key_index = i;
else
break;
}
// If it's the last key or non-smooth animation, then just set the _matrix.
if(_key_index == m_num_matrix_keys - 1 || is_smooth == FALSE)
m_frame_info->m_mat_transformed = m_matrix_keys[_key_index].matrix;
else
{
// calculate the time difference and interpolated time
_time_inter = time - m_matrix_keys[_key_index].time;
// set the new interpolation _matrix
_matrix = m_matrix_keys[_key_index].mat_inter * (float) _time_inter;
m_frame_info->m_mat_transformed = m_matrix_keys[_key_index].matrix + _matrix;
}
}
}
///////////////////////////////////// Define for class ANIM_INFO_SET /////////////////////////////////////
//-----------------------------------------------------------------------------
// Constructor, initialize member data.
//-----------------------------------------------------------------------------
ANIM_INFO_SET::ANIM_INFO_SET()
{
memset(this, 0, sizeof(*this));
}
//-----------------------------------------------------------------------------
// Destructor, release resource.
//-----------------------------------------------------------------------------
ANIM_INFO_SET::~ANIM_INFO_SET()
{
delete[] m_name; m_name = NULL;
delete m_anim_info; m_anim_info = NULL;
delete m_next; m_next = NULL;
}
//-----------------------------------------------------------------------------
// Find animation set information which match specified name in animation
// information set link list.
//-----------------------------------------------------------------------------
ANIM_INFO_SET* ANIM_INFO_SET::find_anim_info_set(const char* name)
{
// return this instance if name is NULL
if(name == NULL)
return this;
// compare names and return if exact match
if(m_name != NULL && STREQ(name, m_name))
return this;
// search next in list
if(m_next)
{
ANIM_INFO_SET* _anim_set;
if((_anim_set = m_next->find_anim_info_set(name)) != NULL)
return _anim_set;
}
return NULL;
}
//-----------------------------------------------------------------------------
// update all animations in current animation set.
//-----------------------------------------------------------------------------
void ANIM_INFO_SET::update_transform_matrix(DWORD time, BOOL is_smooth)
{
ANIM_INFO_PTR _anim_info = m_anim_info;
while(_anim_info)
{
if(m_time_length == 0)
{
// If time length of the animation set is zero, just use first frame.
_anim_info->update_transform_matrix(0, FALSE);
}
else if(time >= m_time_length && _anim_info->m_is_loop == FALSE)
{
// If time beyonds max time length of the animation set and is not loop animation, use last frame.
_anim_info->update_transform_matrix(time, FALSE);
}
else
{
// Now, update animation usually.
_anim_info->update_transform_matrix(time % m_time_length, is_smooth);
}
_anim_info = _anim_info->m_next;
}
}
/////////////////////////////////// Define for class OBJECT ///////////////////////////////////
//-------------------------------------------------------------------
// Constructor, initialize member data.
//-------------------------------------------------------------------
OBJECT::OBJECT()
{
memset(this, 0, sizeof(*this));
}
//-------------------------------------------------------------------
// create an object with specified mesh object.
//-------------------------------------------------------------------
BOOL OBJECT::create(MESH_PTR mesh)
{
if(g_d3d_device == NULL)
return FALSE;
m_mesh = mesh;
move(0.0f, 0.0f, 0.0f);
rotate(0.0f, 0.0f, 0.0f);
scale(1.0f, 1.0f, 1.0f);
return TRUE;
}
//-------------------------------------------------------------------
// Enable or disable billboard.
//-------------------------------------------------------------------
void OBJECT::enable_billboard(BOOL enable)
{
m_world_pos.enable_billboard(enable);
}
//-------------------------------------------------------------------
// Attach another object's frame transformed matrix which match specified
// name to current object.
//-------------------------------------------------------------------
void OBJECT::attach_frame_matrix_to_object(OBJECT* object, const char* frame_name)
{
if(object == NULL || object->m_mesh == NULL)
{
m_world_pos.set_combine_matrix_1(NULL);
m_world_pos.set_combine_matrix_2(NULL);
}
else
{
FRAME_INFO_PTR _frame_info = object->m_mesh->get_frame_info(frame_name);
if(_frame_info == NULL)
{
m_world_pos.set_combine_matrix_1(NULL);
m_world_pos.set_combine_matrix_2(NULL);
}
else
{
m_world_pos.set_combine_matrix_1(&_frame_info->m_mat_combined);
m_world_pos.set_combine_matrix_2(object->m_world_pos.get_matrix());
}
}
}
//-------------------------------------------------------------------
// move object to new world position with specified relative value.
//-------------------------------------------------------------------
void OBJECT::move(float x_pos, float y_pos, float z_pos)
{
m_world_pos.move(x_pos, y_pos, z_pos);
}
//-------------------------------------------------------------------
// move object to new world position relative current position.
//-------------------------------------------------------------------
void OBJECT::move_rel(float x_add, float y_add, float z_add)
{
m_world_pos.move_rel(x_add, y_add, z_add);
}
//-------------------------------------------------------------------
// rotate around x, y, z, axis with specified degree.
//-------------------------------------------------------------------
void OBJECT::rotate(float x_rot, float y_rot, float z_rot)
{
m_world_pos.rotate(x_rot, y_rot, z_rot);
}
//-------------------------------------------------------------------
// rotate around x, y, z, axis relative to current rotation.
//-------------------------------------------------------------------
void OBJECT::rotate_rel(float x_add, float y_add, float z_add)
{
m_world_pos.rotate_rel(x_add, y_add, z_add);
}
//-------------------------------------------------------------------
// scale object with specified scale value.
//-------------------------------------------------------------------
void OBJECT::scale(float x_scale, float y_scale, float z_scale)
{
m_world_pos.scale(x_scale, y_scale, z_scale);
}
//-------------------------------------------------------------------
// scale object with specified scale value relative current scale value.
//-------------------------------------------------------------------
void OBJECT::scale_rel(float x_add, float y_add, float z_add)
{
m_world_pos.scale_rel(x_add, y_add, z_add);
}
//-------------------------------------------------------------------
// Get current world transform matrix.
//-------------------------------------------------------------------
LPD3DXMATRIX OBJECT::get_matrix()
{
return m_world_pos.get_matrix();
}
//-------------------------------------------------------------------
// set mesh to current object.
//-------------------------------------------------------------------
void OBJECT::set_mesh(MESH_PTR mesh)
{
m_mesh = mesh;
}
//-------------------------------------------------------------------
// Get current object's mesh.
//-------------------------------------------------------------------
MESH_PTR OBJECT::get_mesh()
{
return m_mesh;
}
//-------------------------------------------------------------------
// set animation information set with specified name and start time.
//-------------------------------------------------------------------
void OBJECT::set_anim_info_set(ANIMATION* animation, const char* name, ulong start_time)
{
m_start_time = start_time;
if(animation == NULL)
m_anim_info_set = NULL;
else
m_anim_info_set = animation->get_anim_info_set(name);
}
//-------------------------------------------------------------------
// Get the name of animation set.
//-------------------------------------------------------------------
char* OBJECT::get_anim_info_set_name()
{
if(m_anim_info_set == NULL)
return NULL;
return m_anim_info_set->m_name;
}
//-------------------------------------------------------------------
// Reset start time of animation set.
//-------------------------------------------------------------------
void OBJECT::reset_anim_time(ulong start_time)
{
m_start_time = start_time;
}
//-------------------------------------------------------------------
// Get x coordinate of current object.
//-------------------------------------------------------------------
float OBJECT::get_x_pos()
{
return m_world_pos.get_x_pos();
}
//-------------------------------------------------------------------
// Get y coordinate of current object.
//-------------------------------------------------------------------
float OBJECT::get_y_pos()
{
return m_world_pos.get_y_pos();
}
//-------------------------------------------------------------------
// Get z coordinate of current object.
//-------------------------------------------------------------------
float OBJECT::get_z_pos()
{
return m_world_pos.get_z_pos();
}
//-------------------------------------------------------------------
// Get current rotation value which rotate around x axis.
//-------------------------------------------------------------------
float OBJECT::get_x_rotation()
{
return m_world_pos.get_x_rotation();
}
//-------------------------------------------------------------------
// Get current rotation value which rotate around y axis.
//-------------------------------------------------------------------
float OBJECT::get_y_rotation()
{
return m_world_pos.get_y_rotation();
}
//-------------------------------------------------------------------
// Get current rotation value which rotate around z axis.
//-------------------------------------------------------------------
float OBJECT::get_z_rotation()
{
return m_world_pos.get_z_rotation();
}
//-------------------------------------------------------------------
// Get current scale value which around x axis.
//-------------------------------------------------------------------
float OBJECT::get_x_scale()
{
return m_world_pos.get_x_scale();
}
//-------------------------------------------------------------------
// Get current scale value which around y axis.
//-------------------------------------------------------------------
float OBJECT::get_y_scale()
{
return m_world_pos.get_y_scale();
}
//-------------------------------------------------------------------
// Get current scale value which around z axis.
//-------------------------------------------------------------------
float OBJECT::get_z_scale()
{
return m_world_pos.get_z_scale();
}
//-------------------------------------------------------------------
// Get bound coordinate and radius after scale.
//-------------------------------------------------------------------
BOOL OBJECT::get_bounds(float* min_x, float* min_y, float* min_z,
float* max_x, float* max_y, float* max_z,
float* radius)
{
if(m_mesh == NULL)
return FALSE;
// Get bound box coordiante and radius.
m_mesh->get_bounds(min_x, min_y, min_z, max_x, max_y, max_z, radius);
// scale bounds
float x_scale = m_world_pos.get_x_scale();
float y_scale = m_world_pos.get_y_scale();
float z_scale = m_world_pos.get_z_scale();
if(min_x != NULL) *min_x *= x_scale;
if(min_y != NULL) *min_y *= y_scale;
if(min_z != NULL) *min_z *= z_scale;
if(max_x != NULL) *max_x *= x_scale;
if(max_y != NULL) *max_y *= y_scale;
if(max_z != NULL) *max_z *= z_scale;
if(radius != NULL)
{
float length = (float) sqrt(x_scale * x_scale + y_scale * y_scale + z_scale * z_scale);
(*radius) *= length;
}
return TRUE;
}
//-------------------------------------------------------------------
// update world tranform matrix.
//-------------------------------------------------------------------
void OBJECT::update_world_pos()
{
m_world_pos.update();
}
//-----------------------------------------------------------------------------
// update all animations in current animation set.
//-----------------------------------------------------------------------------
void OBJECT::update_anim_info_set(ulong time, BOOL is_smooth)
{
if(m_anim_info_set)
{
// reset all frames's transformed matrices to original matrices
m_mesh->get_root_frame_info()->reset_matrices();
// update all animations in current animation set
m_anim_info_set->update_transform_matrix(time - m_start_time, is_smooth);
}
}
//-----------------------------------------------------------------------------
// Judge whether animation has completed.
//-----------------------------------------------------------------------------
BOOL OBJECT::anim_complete(ulong time)
{
if(m_anim_info_set == NULL)
return TRUE;
if((time - m_start_time) >= m_anim_info_set->m_time_length)
return TRUE;
return FALSE;
}
//-----------------------------------------------------------------------------
// render all meshes in this object.
//-----------------------------------------------------------------------------
BOOL OBJECT::render()
{
// error checking
if(g_d3d_device == NULL || m_mesh == NULL)
return FALSE;
FRAME_INFO_PTR _root_frame_info = m_mesh->get_root_frame_info();
MESH_INFO_PTR _root_mesh_info = m_mesh->get_root_mesh_info();
if(_root_frame_info == NULL || _root_mesh_info == NULL)
return FALSE;
// update the object matrix
update_world_pos();
D3DXMATRIX _matrix;
// update the frame matrices
D3DXMatrixIdentity(&_matrix);
_update_frame_combined_matrix(_root_frame_info, &_matrix);
// copy frame matrices to bone matrices
_root_mesh_info->create_frame_transform_matrices();
// draw all frame meshes
_draw_frame(_root_frame_info);
return TRUE;
}
//-----------------------------------------------------------------------------
// update transformation matrix of all frames, call recursively.
//-----------------------------------------------------------------------------
void OBJECT::_update_frame_combined_matrix(FRAME_INFO_PTR frame_info, LPD3DXMATRIX matrix)
{
// return if no more frames
if(frame_info == NULL)
return;
// calculate frame matrix based on animation or not
if(m_anim_info_set == NULL)
D3DXMatrixMultiply(&frame_info->m_mat_combined, &frame_info->m_mat_original, matrix);
else
D3DXMatrixMultiply(&frame_info->m_mat_combined, &frame_info->m_mat_transformed, matrix);
_update_frame_combined_matrix(frame_info->m_child, &frame_info->m_mat_combined);
_update_frame_combined_matrix(frame_info->m_sibling, matrix);
}
//-----------------------------------------------------------------------------
// Draw all meshes in frames which under current specified frame, call recursively.
//-----------------------------------------------------------------------------
void OBJECT::_draw_frame(FRAME_INFO_PTR frame_info)
{
if(frame_info == NULL)
return;
D3DXMATRIX _mat_world;
MESH_INFO_PTR _mesh_info = frame_info->m_mesh_info;
// draw all meshes in this frame
while(_mesh_info)
{
// generate the mesh if using bones and set world matrix
if(_mesh_info->get_num_bones() && _mesh_info->m_d3d_skin_mesh)
{
void* _src_vertices;
void* _dest_vertices;
ID3DXMesh* _d3d_mesh = _mesh_info->m_d3d_mesh;
ID3DXMesh* _d3d_skin_mesh = _mesh_info->m_d3d_skin_mesh;
// lock the source and destination vertex buffers
_d3d_mesh->LockVertexBuffer(D3DLOCK_READONLY, (void**) &_src_vertices);
_d3d_skin_mesh->LockVertexBuffer(0, (void**) &_dest_vertices);
// perfrom skinned mesh update
_mesh_info->m_d3d_skin_info->UpdateSkinnedMesh(_mesh_info->m_matrices, NULL, _src_vertices, _dest_vertices);
// unlock vertex buffers
_d3d_skin_mesh->UnlockVertexBuffer();
_d3d_mesh->UnlockVertexBuffer();
// set object world transformation
g_d3d_device->SetTransform(D3DTS_WORLD, m_world_pos.get_matrix());
}
else
{
// set the world transformation matrix for this frame
D3DXMatrixMultiply(&_mat_world, &frame_info->m_mat_combined, m_world_pos.get_matrix());
g_d3d_device->SetTransform(D3DTS_WORLD, &_mat_world);
}
// loop through materials and draw the mesh
for(DWORD i = 0; i < _mesh_info->m_num_materials; i++)
{
// don't draw materials with alpha (0.0)
if(_mesh_info->m_d3d_materials[i].Diffuse.a != 0.0f)
{
g_d3d_device->SetMaterial(&_mesh_info->m_d3d_materials[i]);
g_d3d_device->SetTexture(0, _mesh_info->m_d3d_textures[i]);
// enabled alpha blending based on material alpha
if(_mesh_info->m_d3d_materials[i].Diffuse.a != 1.0f)
enable_alpha_blending(TRUE, D3DBLEND_SRCCOLOR, D3DBLEND_ONE);
// draw _mesh_info or skinned mehs
if(_mesh_info->m_d3d_skin_mesh != NULL)
_mesh_info->m_d3d_skin_mesh->DrawSubset(i);
else
_mesh_info->m_d3d_mesh->DrawSubset(i);
// disable alpha blending based on material alpha
if(_mesh_info->m_d3d_materials[i].Diffuse.a != 1.0f)
enable_alpha_blending(FALSE, D3DBLEND_SRCALPHA, D3DBLEND_INVSRCALPHA);
}
}
// next _mesh_info in lsit
_mesh_info = _mesh_info->m_next;
}
// draw child frames and sibling frames
_draw_frame(frame_info->m_child);
_draw_frame(frame_info->m_sibling);
}
测试代码:
/***********************************************************************************
PURPOSE:
Test for object class.
***********************************************************************************/
#include "core_common.h"
#include "core_framework.h"
#include "core_graphics.h"
class APP : public FRAMEWORK
{
public:
BOOL init()
{
// Create Direct3D and Direct3DDevice object
if(! create_display(g_hwnd, get_client_width(g_hwnd), get_client_height(g_hwnd), 16, TRUE, FALSE))
return FALSE;
// set perspective projection transform matrix
set_perspective(D3DX_PI/4.0f, 1.33333f, 1.0f, 1000.0f);
D3DXMATRIX _mat_view;
// create and set the view matrix
D3DXMatrixLookAtLH(&_mat_view,
&D3DXVECTOR3(0.0, 50.0, -150.0),
&D3DXVECTOR3(0.0, 50.0, 0.0),
&D3DXVECTOR3(0.0, 1.0, 0.0));
g_d3d_device->SetTransform(D3DTS_VIEW, &_mat_view);
if(! m_mesh.load("warrior.x", ".\\"))
return FALSE;
if(! m_object.create(&m_mesh))
return FALSE;
return TRUE;
}
BOOL frame()
{
clear_display_buffer(D3DCOLOR_RGBA(0, 0, 0, 255));
if(SUCCEEDED(g_d3d_device->BeginScene()))
{
// rotate object along x-axis, y-axis.
m_object.rotate((float) (timeGetTime() / 2000.0), (float) (timeGetTime() / 1000.0), 0);
m_object.render();
g_d3d_device->EndScene();
}
present_display();
return TRUE;
}
BOOL shutdown()
{
release_com(g_d3d_device);
release_com(g_d3d);
return TRUE;
}
private:
MESH m_mesh;
OBJECT m_object;
};
int PASCAL WinMain(HINSTANCE inst, HINSTANCE, LPSTR cmd_line, int cmd_show)
{
APP app;
if(! build_window(inst, "MainClass", "MainWindow", WS_OVERLAPPEDWINDOW, 0, 0, 640, 480))
return -1;
app.run();
return 0;
}
程序截图:
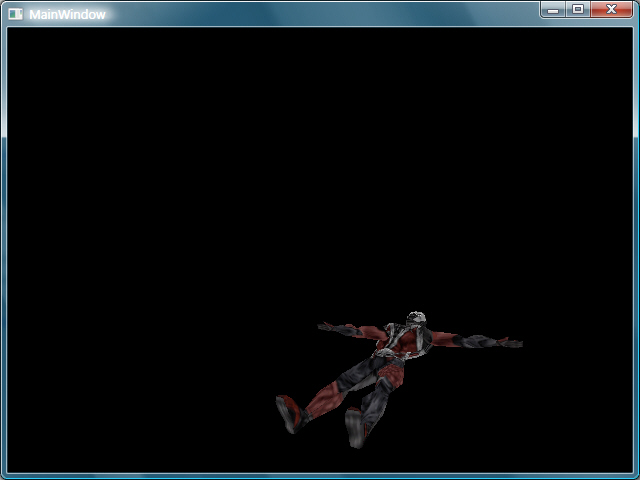