Embedding Data Objects and Template Restrictions
Data referencing has one caveat−the template restrictions set in place must
allow you to use a reference. That might not make sense at first, but you can't
use a data reference without the proper restrictions. An .X file represents an
entire hierarchy of data objects, which can only be siblings or children of
other objects. Thus, data objects embedded in other objects need the proper
restrictions to be referenced or instanced. For example, consider the following
three template declarations:
template ClosedTemplate {
<4C9D055B−C64D−4bfe−A7D9−981F507E45FF>
DWORD ClosedData;
}
template OpenTemplate {
<4C9D055B−C64D−4bff−A7D9−981F507E45FF>
DWORD OpenData;
[...]
}
template RestrictedTemplate {
<4C9D055B−C64D−4c00−A7D9−981F507E45FF>
DWORD RestrictedData;
[ClosedTemplate]
[OpenTemplate]
}
They are pretty standard template declarations, except for the lines that
contain square brackets. The information inside those square brackets is
important. The first template, ClosedTemplate, doesn't have square brackets, so
it is considered a closed template. You can only instance and define the
ClosedData value inside ClosedTemplate.
The OpenTemplate, however, contains the [] line, which signifies that it is
an open template. An open template allows any data object to be embedded in
place of the [] line. For example, you can instance OpenTemplate, define the
OpenData variable, and then embed an instance of ClosedTemplate within the
OpenTemplate.
RestrictedTemplate has two lines of bracket text. Restricting templates only
allow data objects of those template types listed; in this case, those templates
are ClosedTemplate and OpenTemplate. Attempts to embed any other data object
other than the two listed will fail (causing the parse to fail).
Whew−you might have to reread this section a few times to fully understand
the ability to embed and restrict templates within other templates. Once you
have a firm grasp on embedding and restricting, it's time to move on and learn
about DirectX's pre−defined standard templates, which are packaged with the
DirectX SDK.
Working with the DirectX Standard Templates
Now that you've worked your way through templates and data objects, you can
move up a step and see what you can do with them in your project. If you've
taken the time to play around with the DirectX SDK, you'll notice that .X is
widely used for containing mesh information. To that end, Microsoft has packaged
DirectX with a number of templates, which I call the DirectX standard templates.
These templates are used to contain all mesh−related data.
The standard templates are useful because they define almost every aspect of
3D meshes, so take a moment to study them here.
The standard templates, shown in Table 3.2, each have a matching GUID macro
that you use to determine which data object is which in your program. These
macros are defined (using DEFINE_GUID) inside a special file named rmxfguid.h.
The standard templates' GUID macros are easy to remember because you just prefix
the template's name with D3DRM_TID. For instance, the Animation template is
defined by the macro D3DRM_TIDAnimation.
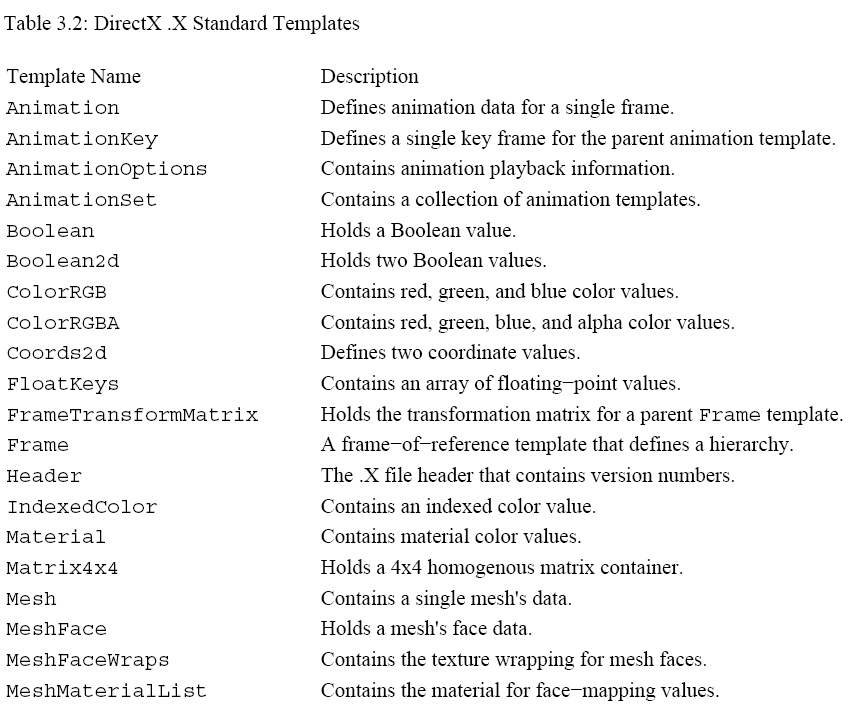
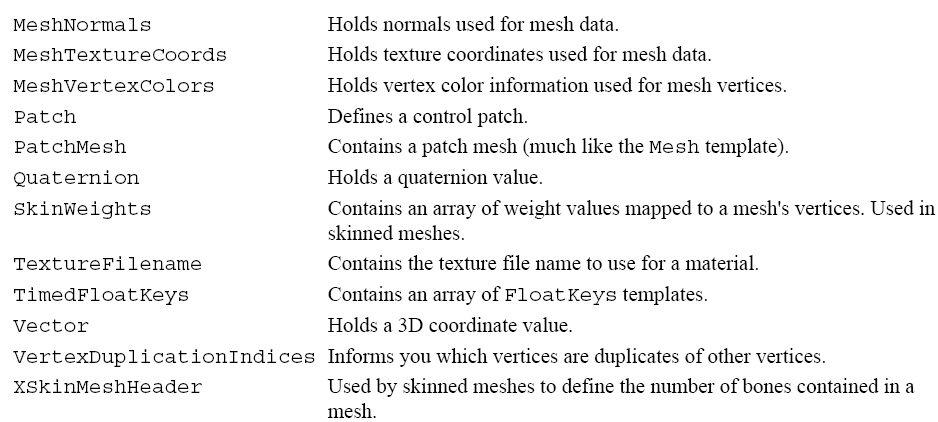
Animation
Contains animations referencing a previous frame. It
should contain one reference to a frame and at least one set of AnimationKey
templates. It also can contain an AnimationOptions data object.
template Animation
{
< 3D82AB4F-62DA-11cf-AB39-0020AF71E433 >
[...]
}
Where:
- [ ... ] - Any .x file template can be used here.
This makes the architecture extensible.
AnimationKey
Defines a set of animation keys. A matrix key is useful
for sets of animation data that need to be represented as transformation
matrices.
template AnimationKey
{
< 10DD46A8-775B-11CF-8F52-0040333594A3 >
DWORD keyType;
DWORD nKeys;
array TimedFloatKeys keys[nKeys];
}
Where:
- keyType - Specifies whether the keys are rotation,
scale, position, or matrix keys (using the integers 0, 1, 2, or 3,
respectively).
- nKeys - Number of keys.
- keys - An array of keys. See TimedFloatKeys.
AnimationOptions
Enables you to set animation options.
template AnimationOptions
{
< E2BF56C0-840F-11cf-8F52-0040333594A3 >
DWORD openclosed;
DWORD positionquality;
}
Where:
- openclosed - Use 0 for a closed animation, or 1
for an open animation. By default, an animation is closed.
- positionquality - Set the position quality for any
position keys specified. Use 0 for spline positions or 1 for linear
positions.
AnimationSet
Contains one or more Animation objects. Each animation
within an animation set has the same time at any given point. Increasing the
animation set's time increases the time for all the animations it contains.
template AnimationSet
{
< 3D82AB50-62DA-11cf-AB39-0020AF71E433 >
[ Animation < 3D82AB4F-62DA-11cf-AB39-0020AF71E433 > ]
}
Where:
- [ Animation < 3D82AB4F-62DA-11cf-AB39-0020AF71E433
> ] - Optional animation template.
Boolean
Defines a simple Boolean type.
template Boolean
{
< 537da6a0-ca37-11d0-941c-0080c80cfa7b >
DWORD truefalse;
}
Where:
- truefalse - True for a Boolean, false otherwise.
Boolean2d
Defines a set of two Boolean values used in the
MeshFaceWraps template to define the texture topology of an individual face.
template Boolean2d
{
< 4885AE63-78E8-11cf-8F52-0040333594A3 >
Boolean u;
Boolean v;
}
Where:
- u - Boolean value. See Boolean.
- v - Boolean value. See Boolean.
ColorRGB
Defines the basic RGB color object.
template ColorRGB
{
< D3E16E81-7835-11cf-8F52-0040333594A3 >
float red;
float green;
float blue;
}
ColorRGBA
Defines a color object with an alpha component. This is
used for the face color in the material template definition.
template ColorRGBA
{
< 35FF44E0-6C7C-11cf-8F52-0040333594A3 >
float red;
float green;
float blue;
float alpha;
}
Coords2d
Defines a two dimensional vector used to define a
mesh's (u, v) texture coordinates.
template Coords2d
{
< F6F23F44-7686-11cf-8F52-0040333594A3 >
float u;
float v;
}
- u - u coordinate value.
- v - v coordinate value.
FloatKeys
Defines an array of floating-point numbers (floats) and
the number of floats in that array. This is used for defining sets of animation
keys.
template FloatKeys
{
< 10DD46A9-775B-11cf-8F52-0040333594A3 >
DWORD nValues;
array float values[nValues];
}
- nValues - Number of floats.
- values[nValues] - Array of float values.
FrameTransformMatrix
Defines a local transform for a frame (and all its
child objects).
template FrameTransformMatrix
{
< F6F23F41-7686-11cf-8F52-0040333594A3 >
Matrix4x4 frameMatrix;
}
Where:
- frameMatrix - A Matrix4x4 template.
Frame
Defines a coordinate frame, or "frame of reference."
The Frame template is open and can contain any object. The D3DX mesh-loading
functions recognize Mesh, FrameTransformMatrix, and Frame template
instances as child objects when loading a Frame instance.
template Frame
{
< 3D82AB46-62DA-11CF-AB39-0020AF71E433 >
[...]
}
The frame template recognizes child Frame and
Mesh nodes inside a frame and can recognize user-defined templates through a
callback function.