Header
The following definitions should be used when directly
reading and writing the binary header.
Note Compressed data
streams are not currently supported and are therefore not detailed here.
#define XOFFILE_FORMAT_MAGIC \
((long)'x' + ((long)'o' << 8) + ((long)'f' << 16) + ((long)' ' << 24))
#define XOFFILE_FORMAT_VERSION \
((long)'0' + ((long)'3' << 8) + ((long)'0' << 16) + ((long)'2' << 24))
#define XOFFILE_FORMAT_BINARY \
((long)'b' + ((long)'i' << 8) + ((long)'n' << 16) + ((long)' ' << 24))
#define XOFFILE_FORMAT_TEXT \
((long)'t' + ((long)'x' << 8) + ((long)'t' << 16) + ((long)' ' << 24))
#define XOFFILE_FORMAT_COMPRESSED \
((long)'c' + ((long)'m' << 8) + ((long)'p' << 16) + ((long)' ' << 24))
#define XOFFILE_FORMAT_FLOAT_BITS_32 \
((long)'0' + ((long)'0' << 8) + ((long)'3' << 16) + ((long)'2' << 24))
#define XOFFILE_FORMAT_FLOAT_BITS_64 \
((long)'0' + ((long)'0' << 8) + ((long)'6' << 16) + ((long)'4' << 24))
IndexedColor
Consists of an index parameter and an RGBA color and is
used for defining mesh vertex colors. The index defines the vertex to which the
color is applied.
template IndexedColor
{
< 1630B820-7842-11cf-8F52-0040333594A3 >
DWORD index;
ColorRGBA indexColor;
}
Where:
- index - A DWORD. See the description above.
- indexColor - A ColorRGBA template.
Material
Defines a basic material color that can be applied to
either a complete mesh or a mesh's individual faces. The power is the specular
exponent of the material.
Note The ambient color
requires an alpha component.
TextureFilename is an optional data object. If this
object is not present, the face is untextured.
template Material
{
< 3D82AB4D-62DA-11CF-AB39-0020AF71E433 >
ColorRGBA faceColor;
FLOAT power;
ColorRGB specularColor;
ColorRGB emissiveColor;
[...]
}
Where:
- faceColor - Face color. A ColorRGBA template.
- power - Material specular color exponent.
- specularColor - Material specular color. A
ColorRGB template.
- emissiveColor - Material emissive color. A
ColorRGB template.
Matrix4x4
Defines a 4 x 4 matrix. This is used as a frame
transformation matrix.
template Matrix4x4
{
< F6F23F45-7686-11cf-8F52-0040333594A3 >
array float matrix[16];
}
Where:
- array float matrix[16] - Array of 16 floats.
Mesh
Defines a simple mesh. The first array is a list of
vertices, and the second array defines the faces of the mesh by indexing into
the vertex array.
template Mesh
{
<3D82AB44-62DA-11CF-AB39-0020AF71E433>
DWORD nVertices;
array Vector vertices[nVertices];
DWORD nFaces;
array MeshFace faces[nFaces];
[...]
}
Where:
- nVertices - Number of vertices.
- array Vector vertices[nVertices] - Array of
vertices, each of type Vector.
- nFaces - Number of faces.
- array MeshFace faces[nFaces] - Array of faces,
each of type MeshFace.
- [ ... ] - Any .x file template can be used here.
This makes the architecture extensible. Material and TextureFilename
templates are typically used.
MeshFace
Used by the Mesh template to define a mesh's faces.
Each element of the nFaceVertexIndices array references a mesh vertex used to
build the face.
template MeshFace
{
< 3D82AB5F-62DA-11cf-AB39-0020AF71E433 >
DWORD nFaceVertexIndices;
array DWORD faceVertexIndices[nFaceVertexIndices];
}
Where:
- nFaceVertexIndices - Number of indices.
- array DWORD faceVertexIndices[nFaceVertexIndices]
- Array of indices.
MeshFaceWraps
Used to define the texture topology of each face in a
wrap. The value of the nFaceWrapValues member should be equal to the number of
faces in a mesh.
template MeshFaceWraps
{
< ED1EC5C0-C0A8-11D0-941C-0080C80CFA7B >
DWORD nFaceWrapValues;
array Boolean2d faceWrapValues[nFaceWrapValues];
}
This template is no longer used.
MeshMaterialList
Used in a mesh object to specify which material applies
to which faces. The nMaterials member specifies how many materials are present,
and materials specify which material to apply.
template MeshMaterialList
{
< F6F23F42-7686-11CF-8F52-0040333594A3 >
DWORD nMaterials;
DWORD nFaceIndexes;
array DWORD faceIndexes[nFaceIndexes];
[Material <3D82AB4D-62DA-11CF-AB39-0020AF71E433>]
}
Where:
- nMaterials - A DWORD. The number of materials.
- nFaceIndexes - A DWORD. The number of indices.
- faceIndexes[nFaceIndexes] - An arrray of DWORDs
containing the face indices.
MeshNormals
Defines normals for a mesh. The first array of vectors
is the normal vectors themselves, and the second array is an array of indexes
specifying which normals should be applied to a given face. The value of the
nFaceNormals member should be equal to the number of faces in a mesh.
template MeshNormals
{
< F6F23F43-7686-11cf-8F52-0040333594A3 >
DWORD nNormals;
array Vector normals[nNormals];
DWORD nFaceNormals;
array MeshFace faceNormals[nFaceNormals];
}
Where:
- nNormals - Number of normals.
- array Vector normals[nNormals] - Array of normals.
- nFaceNormals - Number of face normals.
- array MeshFace faceNormals[nFaceNormals] - Array
of mesh face normals.
MeshTextureCoords
Defines a mesh's texture coordinates.
template MeshTextureCoords
{
< F6F23F40-7686-11cf-8F52-0040333594A3 >
DWORD nTextureCoords;
array Coords2d textureCoords[nTextureCoords] ;
}
Where:
- nTextureCoords - Number of texture coordinates.
- array Coords2d textureCoords[nTextureCoords] -
Array of 2D texture coordinates.
MeshVertexColors
Specifies vertex colors for a mesh, instead of applying
a material per face or per mesh.
template MeshVertexColors
{
<1630B821-7842-11cf-8F52-0040333594A3>
DWORD nVertexColors;
array IndexColor vertexColors[nVertexColors];
}
Where:
- nVertexColors - Number of colors. This matches the
number of vertices in the mesh.
- array IndexColor vertexColors[nVertexColors] -
Array of indexed colors.
Patch
Defines a Bézier control patch. The array defines the
control points for the patch.
template Patch
{
< A3EB5D44-FC22-429D-9AFB-3221CB9719A6 >
DWORD nControlIndices;
array DWORD controlIndices[nControlIndices];
}
Where:
- nControlIndices - Number of control point indices.
- array DWORD controlIndices[nControlIndices] -
Array of control point indices.
The type of patch is defined by the number of control
points, as shown in the following table.
Number of control points |
Type |
10 |
Cubic Bézier triangular patch |
15 |
Quartic Bézier triangular patch |
16 |
Cubic Bézier quad rectangle patch |
The order of the control points are given in a spiral
pattern, as shown in the following diagrams for triangular and rectangular
patches.
Triangular patches used the following pattern.
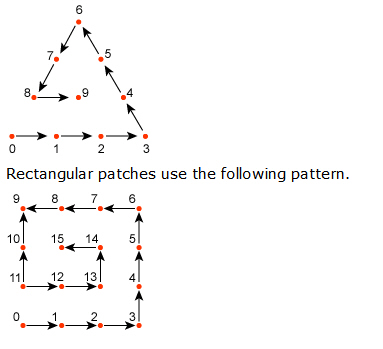
PatchMesh
Defines a mesh defined by Bézier patches. The first
array is a list of vertices, and the second array defines the patches for the
mesh by indexing into the vertex array.
template PatchMesh
{
< D02C95CC-EDBA-4305-9B5D-1820D7704BBF >
DWORD nVertices;
array Vector vertices[nVertices];
DWORD nPatches;
array Patch patches[nPatches];
[ ... ]
}
Where:
- nVertices - Number of vertices.
- vertices[nVertices] - Array of vertices.
- nPatches - Number of patches.
- patches[nPatches] - Array of patches.
- [ ... ] - Any .x file template can be used here.
This makes the architecture extensible.
The patches use the vertices in the array of vertices
as the control points for each patch. This is a legacy template. The latest
patch mesh template is PatchMesh9.
SkinWeights
This template is instantiated on a per-mesh basis.
Within a mesh, a sequence of n instances of this template will appear, where n
is the number of bones (X file frames) that influence the vertices in the mesh.
Each instance of the template basically defines the influence of a particular
bone on the mesh. There is a list of vertex indices, and a corresponding list of
weights.
template SkinWeights
{
< 6F0D123B-BAD2-4167-A0D0-80224F25FABB >
STRING transformNodeName;
DWORD nWeights;
array DWORD vertexIndices[nWeights];
array float weights[nWeights];
Matrix4x4 matrixOffset;
}
Where:
- The name of the bone whose influence is being
defined is transformNodeName, and nWeights is the number of vertices
affected by this bone.
- The vertices influenced by this bone are contained
in vertexIndices, and the weights for each of the vertices influenced by
this bone are contained in weights.
- The matrix matrixOffset transforms the mesh
vertices to the space of the bone. When concatenated to the bone's
transform, this provides the world space coordinates of the mesh as affected
by the bone.
TextureFilename
Enables you to specify the file name of a texture to
apply to a mesh or a face. This template should appear within a material object.
template TextureFilename
{
< A42790E1-7810-11cf-8F52-0040333594A3 >
string filename;
}
TimedFloatKeys
Defines a set of floats and a positive time used in
animations.
template TimedFloatKeys
{
< F406B180-7B3B-11cf-8F52-0040333594A3 >
DWORD time;
FloatKeys tfkeys;
}
Where:
Vector
Defines a vector.
template Vector
{
< 3D82AB5E-62DA-11cf-AB39-0020AF71E433 >
float x;
float y;
float z;
}
VertexDuplicationIndices
This template is instantiated on a per-mesh basis,
holding information about which vertices in the mesh are duplicates of each
other. Duplicates result when a vertex sits on a smoothing group or material
boundary. The purpose of this template is to allow the loader to determine which
vertices exhibiting different peripheral parameters are actually the same
vertexes in the model. Certain applications (mesh simplification, for example)
can make use of this information.
template VertexDuplicationIndices
{
< B8D65549-D7C9-4995-89CF-53A9A8B031E3 >
DWORD nIndices;
DWORD nOriginalVertices;
array DWORD indices[nIndices];
}
Where:
- nIndices - Number of vertex indices. This is the
number of vertices in the mesh.
- nOriginalVertices - Number of vertices in the mesh
before any duplication occurs.
- The value indices[n] holds the vertex index that
vertex[n] in the vertex array for the mesh would have had if no duplication
had occurred. Indices in this array that are the same, therefore, indicate
duplicate vertices.
XSkinMeshHeader
This template is instantiated on a per-mesh basis only
in meshes that contain exported skinning information. The purpose of this
template is to provide information about the nature of the skinning information
that was exported.
template XSkinMeshHeader
{
< 3CF169CE-FF7C-44ab-93C0-F78F62D172E2 >
WORD nMaxSkinWeightsPerVertex;
WORD nMaxSkinWeightsPerFace;
WORD nBones;
}
Where:
- nMaxSkinWeightsPerVertex - Maximum number of
transforms that affect a vertex in the mesh.
- nMaxSkinWeightsPerFace - Maximum number of unique
transforms that affect the three vertices of any face.
- nBones - Number of bones that affect vertices in
this mesh.