目的:测试MAX_PATH路径与文件名的关系。
结论:MAX_PATH代表从盘符开始到文件名结尾的C字符串长度(长度+1)的最大长度。也就是假设C:\a.txt共8个字符,长度为9,MAX_PATH通常为260,其中这个文件全名的长度不能大于260。
测试代码:
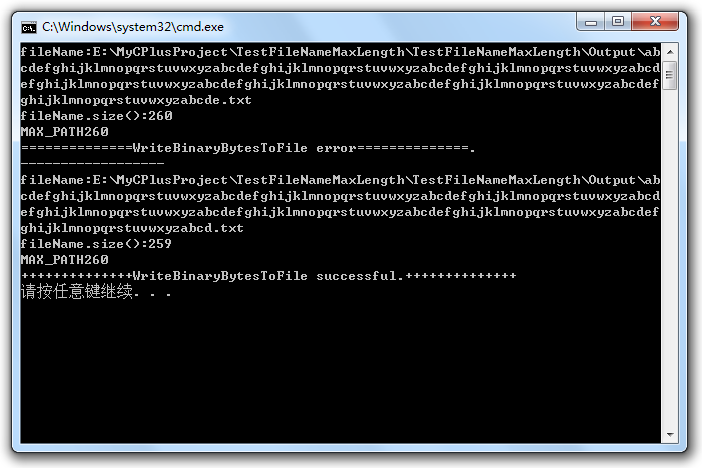
#include "stdafx.h"
#include <atlbase.h>
#include <windows.h>
#include <atlfile.h>
#include <atlstr.h>
#include <iostream>
#include <string>
BOOL IsFileExist( LPCTSTR lpszFileName )
{
DWORD dwAttr = ::GetFileAttributes( lpszFileName );
if ( dwAttr == 0xFFFFFFFF )
{
return FALSE;
}
if ( ( dwAttr & FILE_ATTRIBUTE_DIRECTORY ) > 0 )
{
return FALSE;
}
return TRUE;
}
BOOL DeleteFiles( LPCTSTR lpszPath )
{
TCHAR szFrom[_MAX_PATH+1] = {_T( '\0' )};
lstrcpy( szFrom , lpszPath );
SHFILEOPSTRUCT shf;
memset( &shf, 0, sizeof( SHFILEOPSTRUCT ) );
shf.hwnd = NULL;
shf.pFrom = szFrom;
shf.wFunc = FO_DELETE;
shf.fFlags = FOF_NOCONFIRMMKDIR | FOF_NOCONFIRMATION |
FOF_NOERRORUI | FOF_SILENT;
return SHFileOperation( &shf ) == 0;
}
BOOL WriteBinaryBytesToFile( LPCTSTR fileName, unsigned char* data,
unsigned int datasize , BOOL bAppend )
{
CAtlFile file;
if( !bAppend && IsFileExist( fileName ))
DeleteFiles( fileName );
HRESULT ret = file.Create( fileName, FILE_WRITE_DATA, FILE_SHARE_WRITE, bAppend?OPEN_ALWAYS:CREATE_ALWAYS );
if ( !SUCCEEDED( ret ) )
return FALSE;
if( bAppend )
{
file.Seek( 0, FILE_END );
}
unsigned char *pos = data;
while( datasize > 0 )
{
DWORD dwWrite = 0;
if ( file.Write( pos , datasize , &dwWrite ) != S_OK )
{
file.Close();
return FALSE;
}
datasize -= dwWrite;
pos += dwWrite;
}
file.Close();
return TRUE;
}
BOOL GCreateFile(std::string& fileNamePath,
std::string& fileNameExt,
int shortNameLength,
std::string& data)
{
char c[] = "a";
std::string fileName;
fileName.append(fileNamePath);
while( shortNameLength-- )
{
fileName.append(c);
++*c;
if( *c > 'z' )
{
*c = 'a';
}
}
fileName.append(fileNameExt);
std::cout << "fileName:" << fileName << std::endl;
std::cout << "fileName.size():"<< fileName.size() << std::endl;
std::cout << "MAX_PATH" << MAX_PATH << std::endl;
if( WriteBinaryBytesToFile(fileName.c_str(), (unsigned char*)data.data(), data.size(), FALSE ) )
{
std::cout << "++++++++++++++WriteBinaryBytesToFile successful.++++++++++++++" << std::endl;
return TRUE;
}
else
{
std::cout << "==============WriteBinaryBytesToFile error==============." << std::endl;
return FALSE;
}
}
int _tmain(int argc, _TCHAR* argv[])
{
std::string fileNamePath = "E:\\MyCPlusProject\\TestFileNameMaxLength\\TestFileNameMaxLength\\Output\\";
std::string fileNameExt = ".txt";
std::string data("This is a content.");
int shortNameLength;
shortNameLength = MAX_PATH - fileNameExt.size() - fileNamePath.size();
GCreateFile(fileNamePath, fileNameExt, shortNameLength, data);
std::cout << "------------------" << std::endl;
shortNameLength = MAX_PATH - fileNameExt.size() - fileNamePath.size() - 1;
GCreateFile(fileNamePath, fileNameExt, shortNameLength, data);
return 0;
}
提示:右键项目属性,将字符集设置为“未设置”。