Posted on 2013-06-24 17:40
kongkongzi 阅读(6244)
评论(0) 编辑 收藏 引用 所属分类:
c++ network library
POCO:
参考:
1.
http://pocoproject.org/2.
https://github.com/pocoproject解析版本:poco-1.4.6p1
测试代码--TestServer.cpp:
1
#include "Poco/Net/HTTPServer.h"
2
#include "Poco/Net/HTTPRequestHandler.h"
3
#include "Poco/Net/HTTPRequestHandlerFactory.h"
4
#include "Poco/Net/HTTPServerParams.h"
5
#include "Poco/Net/HTTPServerRequest.h"
6
#include "Poco/Net/HTTPServerResponse.h"
7
#include "Poco/Net/HTTPServerParams.h"
8
#include "Poco/Net/ServerSocket.h"
9
#include "Poco/Timestamp.h"
10
#include "Poco/DateTimeFormatter.h"
11
#include "Poco/DateTimeFormat.h"
12
#include "Poco/Exception.h"
13
#include "Poco/ThreadPool.h"
14
#include "Poco/Util/ServerApplication.h"
15
#include "Poco/Util/Option.h"
16
#include "Poco/Util/OptionSet.h"
17
#include "Poco/Util/HelpFormatter.h"
18
#include <iostream>
19
20
using Poco::Net::ServerSocket;
21
using Poco::Net::HTTPRequestHandler;
22
using Poco::Net::HTTPRequestHandlerFactory;
23
using Poco::Net::HTTPServer;
24
using Poco::Net::HTTPServerRequest;
25
using Poco::Net::HTTPServerResponse;
26
using Poco::Net::HTTPServerParams;
27
using Poco::Timestamp;
28
using Poco::DateTimeFormatter;
29
using Poco::DateTimeFormat;
30
using Poco::ThreadPool;
31
using Poco::Util::ServerApplication;
32
using Poco::Util::Application;
33
using Poco::Util::Option;
34
using Poco::Util::OptionSet;
35
using Poco::Util::OptionCallback;
36
using Poco::Util::HelpFormatter;
37
38
class TimeRequestHandler: public HTTPRequestHandler
39

{
40
public:
41
TimeRequestHandler(const std::string& format): _format(format)
42
{
43
}
44
45
void handleRequest(HTTPServerRequest& request,
46
HTTPServerResponse& response)
47
{
48
Application& app = Application::instance();
49
app.logger().information("Request from "
50
+ request.clientAddress().toString());
51
52
Timestamp now;
53
std::string dt(DateTimeFormatter::format(now, _format));
54
55
response.setChunkedTransferEncoding(true);
56
response.setContentType("text/html");
57
58
std::ostream& ostr = response.send();
59
ostr << "<html><head><title>HTTPTimeServer powered by "
60
"POCO C++ Libraries</title>";
61
ostr << "<meta http-equiv=\"refresh\" content=\"1\"></head>";
62
ostr << "<body><p style=\"text-align: center; "
63
"font-size: 48px;\">";
64
ostr << dt;
65
ostr << "</p></body></html>";
66
}
67
68
private:
69
std::string _format;
70
};
71
72
class TimeRequestHandlerFactory: public HTTPRequestHandlerFactory
73

{
74
public:
75
TimeRequestHandlerFactory(const std::string& format):
76
_format(format)
77
{
78
}
79
80
HTTPRequestHandler* createRequestHandler(
81
const HTTPServerRequest& request)
82
{
83
if (request.getURI() == "/")
84
return new TimeRequestHandler(_format);
85
else
86
return 0;
87
}
88
89
private:
90
std::string _format;
91
};
92
93
class HTTPTimeServer: public Poco::Util::ServerApplication
94

{
95
public:
96
HTTPTimeServer(): _helpRequested(false)
97
{
98
}
99
100
~HTTPTimeServer()
101
{
102
}
103
104
protected:
105
void initialize(Application& self)
106
{
107
loadConfiguration();
108
ServerApplication::initialize(self);
109
}
110
111
void uninitialize()
112
{
113
ServerApplication::uninitialize();
114
}
115
116
void defineOptions(OptionSet& options)
117
{
118
ServerApplication::defineOptions(options);
119
120
options.addOption(
121
Option("help", "h", "display argument help information")
122
.required(false)
123
.repeatable(false)
124
.callback(OptionCallback<HTTPTimeServer>(
125
this, &HTTPTimeServer::handleHelp)));
126
}
127
128
void handleHelp(const std::string& name,
129
const std::string& value)
130
{
131
HelpFormatter helpFormatter(options());
132
helpFormatter.setCommand(commandName());
133
helpFormatter.setUsage("OPTIONS");
134
helpFormatter.setHeader(
135
"A web server that serves the current date and time.");
136
helpFormatter.format(std::cout);
137
stopOptionsProcessing();
138
_helpRequested = true;
139
}
140
141
int main(const std::vector<std::string>& args)
142
{
143
if (!_helpRequested)
144
{
145
unsigned short port = (unsigned short)
146
config().getInt("HTTPTimeServer.port", 9980);
147
std::string format(
148
config().getString("HTTPTimeServer.format",
149
DateTimeFormat::SORTABLE_FORMAT));
150
151
ServerSocket svs(port);
152
HTTPServer srv(new TimeRequestHandlerFactory(format),
153
svs, new HTTPServerParams);
154
srv.start();
155
waitForTerminationRequest();
156
srv.stop();
157
}
158
return Application::EXIT_OK;
159
}
160
161
private:
162
bool _helpRequested;
163
};
164
165
int main(int argc, char** argv)
166

{
167
HTTPTimeServer app;
168
return app.run(argc, argv);
169
} 对应的配置文件--TestServer.xml:
<config>
<HTTPTimeServer>
<port>3112</port>
</HTTPTimeServer>
</config>图1:poco-acceptConnection
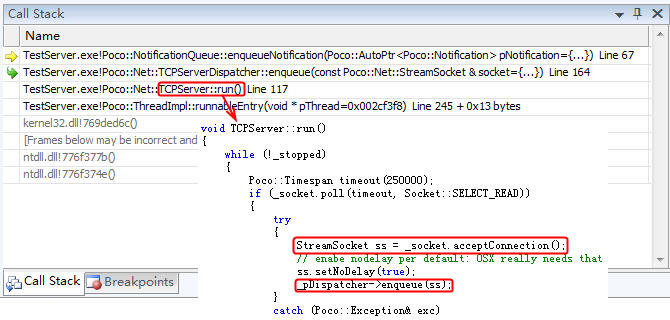
图2:poco-dispatchConnection
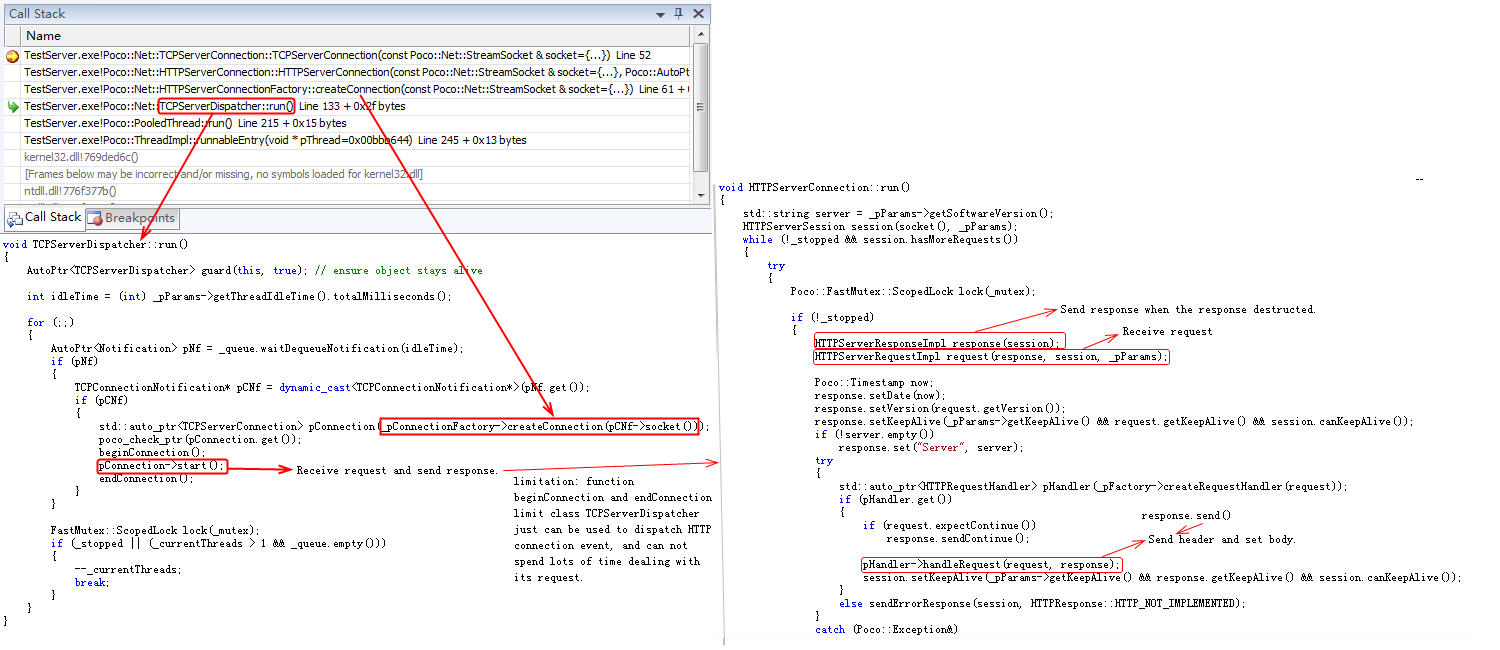
图3:poco-recvRequest
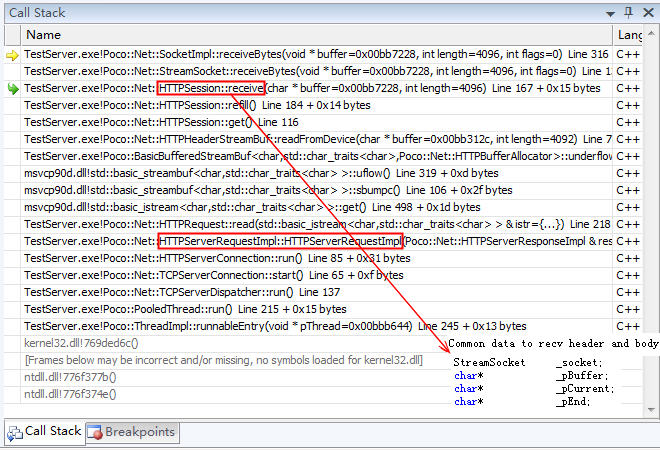
图4:poco-sendResponseHeader
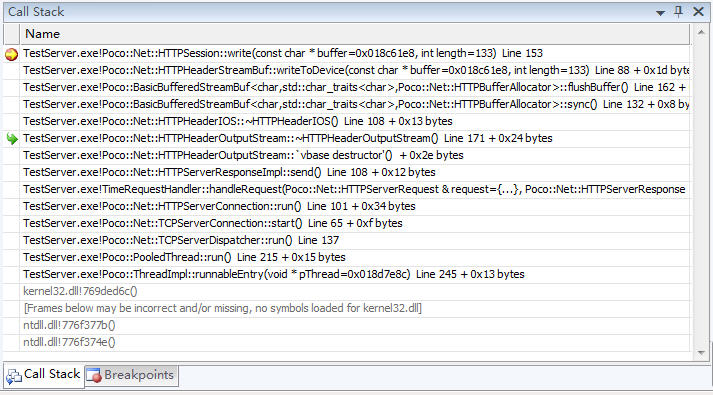
图5:poco-sendResponseBody
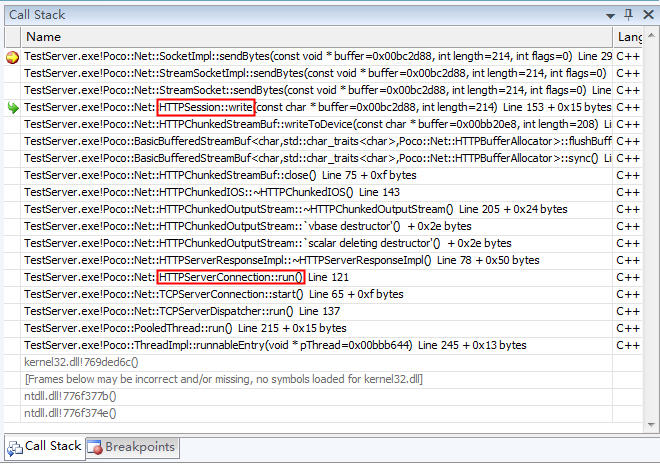
poco-HTTP message:
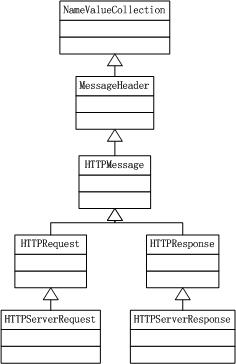