适配器模式(Adapter):将一个类的接口转换成客户希望的另外一个接口。Adapter 模式使得原本由于接口不兼容而不能在一起工作的那些类可以一起工作。
结构型
·类
·对象
UML 类图:
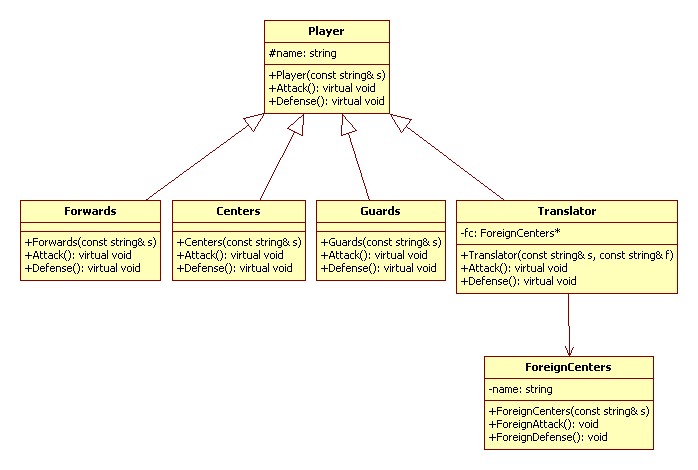
代码实现 C++:
1 #include <iostream>
2 #include <string>
3 using namespace std;
4
5 class Player
6 {
7 protected:
8 string name;
9 public:
10 Player(const string& s = "") : name(s) {}
11 virtual void Attack() = 0;
12 virtual void Defense() = 0;
13 };
14
15 class Forwards : public Player
16 {
17 public:
18 Forwards(const string& s = "") : Player(s) {}
19 virtual void Attack()
20 {
21 cout << "前锋 " << name << " 进攻!" << endl;
22 }
23 virtual void Defense()
24 {
25 cout << "前锋 " << name << " 防守!" << endl;
26 }
27 };
28
29 class Center : public Player
30 {
31 public:
32 Center(const string& s = "") : Player(s) {}
33 virtual void Attack()
34 {
35 cout << "中锋 " << name << " 进攻!" << endl;
36 }
37 virtual void Defense()
38 {
39 cout << "中锋 " << name << " 防守!" << endl;
40 }
41 };
42
43 class Guards : public Player
44 {
45 public:
46 Guards(const string& s) : Player(s) {}
47 virtual void Attack()
48 {
49 cout << "后卫 " << name << " 进攻!" << endl;
50 }
51 virtual void Defense()
52 {
53 cout << "后卫 " << name << " 防守!" << endl;
54 }
55 };
56
57 class ForeignCenters
58 {
59 private:
60 string name;
61 public:
62 ForeignCenters(const string& s = "") : name(s) {}
63 void ForeignAttack()
64 {
65 cout << "外籍中锋 " << name << " 进攻!" << endl;
66 }
67 void ForeignDefense()
68 {
69 cout << "外籍中锋 " << name << " 防守!" << endl;
70 }
71 };
72
73 class Translators : public Player
74 {
75 private:
76 ForeignCenters* fc;
77 public:
78 Translators(const string& s = "", const string& f = "") : Player(s), fc(new ForeignCenters(f)) {}
79 ~Translators()
80 {
81 delete fc;
82 }
83 virtual void Attack()
84 {
85 fc->ForeignAttack();
86 }
87 virtual void Defense()
88 {
89 fc->ForeignDefense();
90 }
91 };
92
93 int main()
94 {
95 Player* b = new Forwards("巴蒂尔");
96 b->Attack();
97 b->Defense();
98
99 Player* m = new Guards("麦克格雷迪");
100 m->Attack();
101 m->Defense();
102
103 Player* y = new Translators("翻译者", "姚明");
104 y->Attack();
105 y->Defense();
106
107 return 0;
108 }
posted on 2011-04-28 14:31
unixfy 阅读(109)
评论(0) 编辑 收藏 引用