先简单介绍一下命令行编译C++程序的方法。
编写如下代码,输出参数的个数和各个参数:
- #include<stdio.h>
- #include<stdlib.h>
- int main(int argc,char* argv[])
- {
- printf("argc:%d\n",argc);
- for(int i = 0;i < argc; ++i)
- printf("%s\n",argv[i]);
- system("pause");
- }
代码中,
argc包括命令行选项的个数,argv包含argc个C风格字符串,代表了由空格分隔的命令选项。(程序中可以使用这个为标志来做相应的处理)例如,对于如下命令行:prog -d -o ofile data0,argc被设置为5,且argv被设置为下列C风格字符串:
- argv[0] = "prog";
- argv[0] = "-d";
- argv[0] = "-o";
- argv[0] = "ofile";
- argv[0] = "data0";
aegv[0]总是被设置为当前正在被调用的命令(程序运行生成的exe文件名,不包括扩展名)。从索引1到argc-1表示被传递给命令的实际选项。
命令行编译程序的具体步骤:
先编译运行上述代码,在将生成的exe文件拷贝至C盘目录下(自己设置)。然后打开命令提示符:
输入cd C:\切换工作目录至C盘目录。

再输入prog hust whu whut,运行结果:
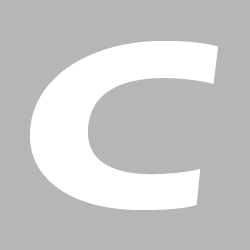
下面看一下如何取出在argv中的命令行选项。在例子中,将支持下列用法:
program_name [-d] [-h] [-v] [-o output_file] [-l limit_value] file_name [file_name [file_name [...]]]。
加括号的内容是可选的。具体步骤见程序:
- //C/C++处理main()函数命令行
- #include <iostream>
- #include <vector>
- #include <string>
- #include <ctype.h>//调用atoi函数
- using namespace std;
-
- const char* const prog_name = "prog";
- const char* const prog_version = "version 1.0 (2011/8/24)";
-
- //退出函数
- inline void usage(int exit_value = 0){
- cerr<<prog_name<<" usage!"<<endl;
- exit(exit_value);
- }
-
- int main(int argc,char* argv[]){
- //声明用于记录用户指定选项的变量
- bool debug_on = false;
- bool ofile_on = false;
- bool limit_on = false;
-
- string ofile_name;//记录出现的输出文件名
- int limit = -1;//限制值
- vector <string> file_names;//记录文件名
-
- cout<<"argc:"<<argc<<endl;
- for(int i = 1;i < argc; ++i){//读取argv中的每个选项
- //输出第i+1个参量
- cout<<"argv["<<i<<"]:"<<argv[i]<<endl;
-
- char *pchar = argv[i];
- switch(pchar[0]){//确定选项类型:-h,-d,-v,-l,-o;或者其他
- case '-':{
- cout<<"case \'-\' found"<<endl;
- switch(pchar[1]){//确定用户指定的选项:h,d,v,l,o
- case 'd'://处理调试:
- cout<<"-d found:debugging turned on!"<<endl;
- debug_on = true;
- break;
- case 'v'://处理版本请求
- cout<<"-v found:version info displayed!"<<endl;
- cout<<prog_name<<":"<<prog_version<<endl;
- return 0;
- case 'h'://处理帮助
- cout<<"-h found:help info!"<<endl;
- usage();
- case 'o'://处理输出文件
- cout<<"-o found:output file!"<<endl;
- ofile_on = true;
- break;
- case 'l'://处理限制量
- cout<<"-l found:resorce limit!"<<endl;
- limit_on = true;
- break;
- default://无法识别的选项
- cerr<<prog_name<<":error:unrecognition option -:"<<pchar<<endl;
- usage(-1);
- }
- break;
- }
- default://不以'-'开头,是文件名
- if(ofile_on){//输出文件名
- cout<<"file name:"<<pchar<<endl;
- ofile_name = pchar;
- ofile_on = false;//复位
- }
- else if(limit_on){//限制值
- limit_on = false;
- limit = atoi(pchar);
- if(limit<0){
- cerr<<prog_name<<":error:negative value for limit!"<<endl;
- usage(-2);
- }
- }
- else{//文件名
- file_names.push_back(pchar);
- }
- break;
- }
- }
- if(file_names.empty()){
- cerr<<prog_name<<":error:no file for processing!"<<endl;
- usage(3);
- }
- else{
- cout<<(file_names.size() == 1 ? "File":"Files")<<
- " to be processed are the followed:"<<endl;
- for(int i = 0;i < file_names.size();++i){
- cout<<file_names[i]<<"\t"<<endl;
- }
- }
- if(limit != -1){
- cout<<"user-specified limit:"<<limit<<endl;
- }
- if(!ofile_name.empty()){
- cout<<"user-specified ofile:"<<ofile_name<<endl;
- }
-
- }