偶然从邮箱里发现的古董。。。
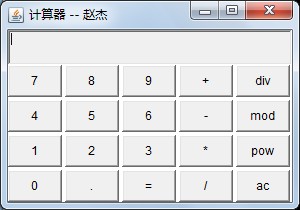
我的代码:
1 // windows
2
3
4 /*
5 版本 1.0
6
7 显示一行数字
8 简单二元运算
9 数字为 double 型
10 无输入数字位数限制
11 可输入过多的小数点
12
13 界面:
14 7 8 9 + div
15 4 5 6 - mod
16 1 2 3 * pow
17 0 . = / ac
18 */
19
20 import java.awt.*;
21 import java.awt.event.*;
22
23 public class Calculator extends Frame implements ActionListener {
24
25 public static void main(String[] args) {
26 new Calculator();
27 }
28
29 public Calculator() {
30 super( "计算器 -- 赵杰" );
31
32 // keyBoard and key
33 key = new Button[ 300 ];
34 Panel kb = new Panel();
35 kb.setLayout( new GridLayout( 4, 5, 3, 3 ) );
36 int i;
37
38 key[ i = (int)'7' ] = new Button( "7" );
39 key[ i ].addActionListener( this );
40 kb.add( key[ i ] );
41
42 key[ i = (int)'8' ] = new Button( "8" );
43 key[ i ].addActionListener( this );
44 kb.add( key[ i ] );
45
46 key[ i = (int)'9' ] = new Button( "9" );
47 key[ i ].addActionListener( this );
48 kb.add( key[ i ] );
49
50 key[ i = (int)'+' ] = new Button( "+" );
51 key[ i ].addActionListener( this );
52 kb.add( key[ i ] );
53
54 key[ i = (int)'d' ] = new Button( "div" );
55 key[ i ].addActionListener( this );
56 kb.add( key[ i ] );
57
58 key[ i = (int)'4' ] = new Button( "4" );
59 key[ i ].addActionListener( this );
60 kb.add( key[ i ] );
61
62 key[ i = (int)'5' ] = new Button( "5" );
63 key[ i ].addActionListener( this );
64 kb.add( key[ i ] );
65
66 key[ i = (int)'6' ] = new Button( "6" );
67 key[ i ].addActionListener( this );
68 kb.add( key[ i ] );
69
70 key[ i = (int)'-' ] = new Button( "-" );
71 key[ i ].addActionListener( this );
72 kb.add( key[ i ] );
73
74 key[ i = (int)'m' ] = new Button( "mod" );
75 key[ i ].addActionListener( this );
76 kb.add( key[ i ] );
77
78 key[ i = (int)'1' ] = new Button( "1" );
79 key[ i ].addActionListener( this );
80 kb.add( key[ i ] );
81
82 key[ i = (int)'2' ] = new Button( "2" );
83 key[ i ].addActionListener( this );
84 kb.add( key[ i ] );
85
86 key[ i = (int)'3' ] = new Button( "3" );
87 key[ i ].addActionListener( this );
88 kb.add( key[ i ] );
89
90 key[ i = (int)'*' ] = new Button( "*" );
91 key[ i ].addActionListener( this );
92 kb.add( key[ i ] );
93
94 key[ i = (int)'p' ] = new Button( "pow" );
95 key[ i ].addActionListener( this );
96 kb.add( key[ i ] );
97
98 key[ i = (int)'0' ] = new Button( "0" );
99 key[ i ].addActionListener( this );
100 kb.add( key[ i ] );
101
102 key[ i = (int)'.' ] = new Button( "." );
103 key[ i ].addActionListener( this );
104 kb.add( key[ i ] );
105
106 key[ i = (int)'=' ] = new Button( "=" );
107 key[ i ].addActionListener( this );
108 kb.add( key[ i ] );
109
110 key[ i = (int)'/' ] = new Button( "/" );
111 key[ i ].addActionListener( this );
112 kb.add( key[ i ] );
113
114 key[ i = (int)'a' ] = new Button( "ac" );
115 key[ i ].addActionListener( this );
116 kb.add( key[ i ] );
117
118 this.add( kb );
119
120 // window
121 this.addWindowListener( new WindowAdapter() {
122 public void windowClosing( WindowEvent e ) {
123 System.exit( 0 );
124 }
125 } );
126 this.setBounds( 200, 200, 300, 210 );
127 this.setLayout( new BorderLayout() );
128 dis = new TextArea( "", 1, 20, TextArea.SCROLLBARS_NONE );
129 dis.setEditable( false );
130 this.add( dis, BorderLayout.NORTH );
131 this.add( kb, BorderLayout.CENTER );
132 this.setVisible( true );
133 this.validate();
134
135 // data
136 bError = false;
137 totInput = 0;
138 lastAns = false;
139 }
140
141 public void actionPerformed( ActionEvent e ) {
142 Object ob = e.getSource();
143 if ( bError && ( ob != key[ (int)'a' ] ) ) {
144 return;
145 }
146 for ( char ch = '0'; ch <= '9'; ++ch ) {
147 if ( ob == key[ (int)ch ] ) {
148 if ( ( totInput == 0 ) || ( totInput == 2 ) ) {
149 dis.setText( "" );
150 ++totInput;
151 }
152 if ( lastAns ) {
153 dis.setText( "" );
154 lastAns = false;
155 totInput = 1;
156 }
157 dis.append( new Character( ch ).toString() );
158 this.validate();
159 return;
160 }
161 }
162 if ( ob == key[ (int)'.' ] ) {
163 if ( ( totInput == 0 ) || ( totInput == 2 ) ) {
164 return;
165 }
166 dis.append( "." );
167 this.validate();
168 return;
169 }
170 if ( ob == key[ (int)'a' ] ) {
171 dis.setText( "" );
172 totInput = 0;
173 bError = false;
174 lastAns = false;
175 this.validate();
176 return;
177 }
178 lastAns = false;
179 if ( totInput == 0 ) {
180 return;
181 }
182 if ( ( totInput != 3 ) && ( ob == key[ (int)'=' ] ) ) {
183 return;
184 }
185 if ( ( totInput == 1 ) || ( totInput == 3 ) ) {
186 if ( cantStrToNum( totInput == 1 ) ) {
187 dis.setText( "Error" );
188 bError = true;
189 this.validate();
190 return;
191 }
192 if ( totInput == 3 ) {
193 if ( cantCalcAns() ) {
194 dis.setText( "Error" );
195 bError = true;
196 this.validate();
197 return;
198 }
199 dis.setText( new Double( ans ).toString() );
200 totInput = 1;
201 this.validate();
202 lastAns = true;
203 return;
204 }
205 ++totInput;
206 }
207 if ( ob == key[ (int)'+' ] ) {
208 op = '+';
209 dis.setText( "+" );
210 this.validate();
211 return;
212 }
213 if ( ob == key[ (int)'d' ] ) {
214 op = 'd';
215 dis.setText( "div" );
216 this.validate();
217 return;
218 }
219 if ( ob == key[ (int)'-' ] ) {
220 op = '-';
221 dis.setText( "-" );
222 this.validate();
223 return;
224 }
225 if ( ob == key[ (int)'m' ] ) {
226 op = 'm';
227 dis.setText( "mod" );
228 this.validate();
229 return;
230 }
231 if ( ob == key[ (int)'*' ] ) {
232 op = '*';
233 dis.setText( "*" );
234 this.validate();
235 return;
236 }
237 if ( ob == key[ (int)'p' ] ) {
238 op = 'p';
239 dis.setText( "pow" );
240 this.validate();
241 return;
242 }
243 if ( ob == key[ (int)'/' ] ) {
244 op = '/';
245 dis.setText( "/" );
246 this.validate();
247 return;
248 }
249 }
250
251 private boolean cantCalcAns() {
252 switch ( op ) {
253 case '+' : ans = first + second; break;
254 case '-' : ans = first - second; break;
255 case '*' : ans = first * second; break;
256 case '/' :
257 if ( second == 0 ) {
258 return true;
259 }
260 ans = first / second; break;
261 case 'd' :
262 if ( second == 0 ) {
263 return true;
264 }
265 ans = ((int)first) / ((int)second); break;
266 case 'm' :
267 if ( second == 0 ) {
268 return true;
269 }
270 ans = ((int)first) % ((int)second); break;
271 case 'p' : ans = Math.pow( first, second ); break;
272 }
273 return false;
274 }
275
276 private boolean cantStrToNum( boolean toFirst ) {
277 try {
278 if ( toFirst ) {
279 first = Double.parseDouble( dis.getText() );
280 }
281 else {
282 second = Double.parseDouble( dis.getText() );
283 }
284 }
285 catch ( Exception ex ) {
286 return true;
287 }
288 return false;
289 }
290
291 private TextArea dis;
292 private Button[] key;
293 private double first, second, ans;
294 private char op;
295 private int totInput;
296 private boolean bError, lastAns;
297 // private final int MAX_NUM_LEN = 13;
298
299 }
300