This is the 4Th Arcticle: Builder PATTERN
Separates the construction of a complex object from its representation so that the same construction process can create different representations. --Gof
The builder pattern can separate the representation and construction easily , while you building a big-pang object , and some of the object itself is
diversified and could be build separately , considering this pattern.
Participants:
Builder.
- specifies an abstract interface for creating parts of a Product object
ConcreteBuilder.
- constructs and assembles parts of the product by implementing the Builder interface
- defines and keeps track of the representation it creates
- provides an interface for retrieving the product
Director.
- constructs an object using the Builder interface
Product.
- represents the complex object under construction. ConcreteBuilder builds the product's internal representation and defines the process by which it's assembled
- includes classes that define the constituent parts, including interfaces for assembling the parts into the final result
The diagram goes here:
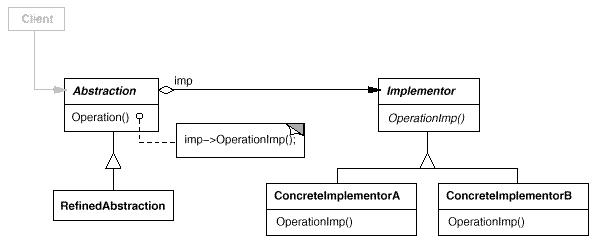
Code is the most clearly lauguage for out programmers , so I will use code to expree this pattern clearly as follows:
1
2
/**//* this is the diversfied part*/
3
4
//abstract
5
class House
6

{
7
8
};
9
//abstract
10
class Door
11

{
12
13
};
14
15
//concrete, transformation
16
class FeDoor: public Door
17

{
18
19
};
20
//concreate, transformation
21
class WoodDoor: public Door
22

{
23
24
};
25
26
//abstract
27
class Window
28

{
29
30
};
31
//concreate , transformation
32
class FeWindow :public Window
33

{
34
35
};
36
//concreate, transformation
37
class WoodWindow :public Window
38

{
39
40
};
41
42
/**//*this is the builder the stable part*/
43
class Builder
44

{
45
46
protected:
47
//build every part here
48
virtual void BuildeDoor();
49
virtual void BuildeWindow();
50
virtual void BuildFloor();
51
//to get the result
52
public:
53
virtual void GetHouse();
54
55
}
56
57
class FeHouseBuilder :public Builder
58

{
59
public:
60
GetHouse()
61
{
62
return /**//*window + door */
63
}
64
protected:
65
void BuildeDoor()
66
{
67
68
aDoor=new FeDoor();
69
}
70
void BuildWindow()
71
{
72
aRedWindow=new FeWindow();
73
}
74
private:
75
Window aWindow;
76
Door aDoor;
77
78
}
79
80
class WoodHouseBuilder :public Builder
81

{
82
public:
83
House GetHouse()
84
{
85
return /**//*window + door */
86
}
87
protected:
88
void BuildeDoor()
89
{
90
91
aDoor=new WoodDoor(); //can change
92
}
93
void BuildWindow()
94
{
95
aRedWindow=new WoodWindow(); //can change too
96
}
97
private:
98
Window aWindow;
99
Door aDoor;
100
101
}
102
103
class Director
104

{
105
public:
106
//Create a house that has two doors and a window , this is the creator part , It's usually Stable too
107
static House Construct(Builder builder)
108
{
109
builder.BuildeDoor();
110
builder.BuildeDoor();
111
builder.BuildeWindow();
112
113
return builder.GetHouse();
114
}
115
116
};
117
118
//client
119
int main(int argc , char * argv[])
120

{
121
//read the config file
122
//build a fe House with two doors and a window
123
Director::Construct(new FeHouseBuilder());
124
//builder a wood house with two doors and a window
125
Director::Construct(new WoodHouseBuilder());
126
127
/**//*two doors and a window is stable , and the house 's architecture is stable too ,but the material*/
128
129
}
1-40 is the diversification , the house's architecture is stable , you create a mixed house easily if you like , simply add a class called mixedhouseBuidler and use the right part(like the FeDoor and WoodDoor ) to create the mixed house ,and use the mixedHouseBuilder to builde you work.
simple and practible!