AbstractFactory模式解决的问题是创建一组相关或者相互依赖的对象。
我们以一个电脑产品的例子来说明。
我们现在要生产电脑产品,假设电脑产品现在只有台式机及笔记本两种,我们需要建一个工厂用来生产电脑产品,而工厂中可以生产不同品牌的电脑,对于每个品牌,我们分别建立相应的品牌工厂,负责生产各自的品牌产品,假设现在有DELL及IBM两个品牌工厂,那么现在每个工厂都可以生产各自的台式机及笔记本了。
其类图如下:
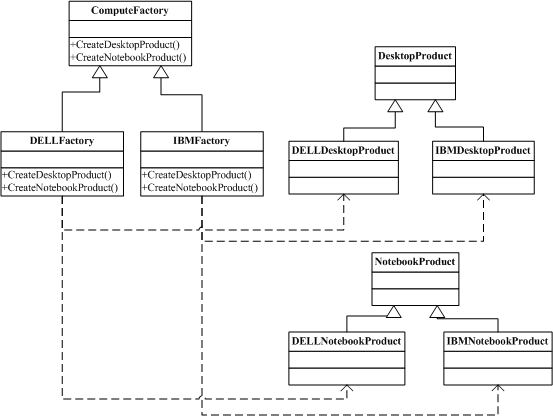
以下是实现代码:
//DesktopProduct.h
class DesktopProduct
{
public:
virtual ~DesktopProduct();
protected:
DesktopProduct();
};
//DesktopProduct.cpp
#include "stdafx.h"
#include "DesktopProduct.h"
DesktopProduct::DesktopProduct()
{
}
DesktopProduct::~DesktopProduct()
{
}
//DELLDesktopProduct.h
#include "DesktopProduct.h"
class DELLDesktopProduct : public DesktopProduct
{
public:
DELLDesktopProduct();
virtual ~DELLDesktopProduct();
};
//DELLDesktopProduct.cpp
#include "stdafx.h"
#include "DELLDesktopProduct.h"
#include <iostream>
using namespace std;
DELLDesktopProduct::DELLDesktopProduct()
{
cout << "创建DELL台式机" << endl;
}
DELLDesktopProduct::~DELLDesktopProduct()
{
}
//IBMDesktopProduct.h
#include "DesktopProduct.h"
class IBMDesktopProduct : public DesktopProduct
{
public:
IBMDesktopProduct();
virtual ~IBMDesktopProduct();
};
//IBMDesktopProduct.cpp
#include "stdafx.h"
#include "IBMDesktopProduct.h"
#include <iostream>
using namespace std;
IBMDesktopProduct::IBMDesktopProduct()
{
cout << "创建IBM台式机" << endl;
}
IBMDesktopProduct::~IBMDesktopProduct()
{
}
//NotebookProduct.h
class NotebookProduct
{
public:
virtual ~NotebookProduct();
protected:
NotebookProduct();
};
//NotebookProduct.cpp
#include "stdafx.h"
#include "NotebookProduct.h"
NotebookProduct::NotebookProduct()
{
}
NotebookProduct::~NotebookProduct()
{
}
//DELLNotebookProduct.h
#include "NotebookProduct.h"
class DELLNotebookProduct : public NotebookProduct
{
public:
DELLNotebookProduct();
virtual ~DELLNotebookProduct();
};
//DELLNotebookProduct.cpp
#include "stdafx.h"
#include "DELLNotebookProduct.h"
#include <iostream>
using namespace std;
DELLNotebookProduct::DELLNotebookProduct()
{
cout << "创建DELL笔记本" << endl;
}
DELLNotebookProduct::~DELLNotebookProduct()
{
}
//IBMNotebookProduct.h
#include "NotebookProduct.h"
class IBMNotebookProduct : public NotebookProduct
{
public:
IBMNotebookProduct();
virtual ~IBMNotebookProduct();
};
//IBMNotebookProduct.cpp
using namespace std;
IBMNotebookProduct::IBMNotebookProduct()
{
cout << "创建IBM笔记本" << endl;
}
IBMNotebookProduct::~IBMNotebookProduct()
{
}
//AbstractFactory.h
class DesktopProduct;
class NotebookProduct;
class AbstractFactory
{
public:
virtual ~AbstractFactory();
virtual DesktopProduct* CreateDesktopProduct() = 0;
virtual NotebookProduct* CreateNotebookProduct() = 0;
protected:
AbstractFactory();
};
//AbstractFactory.cpp
#include "stdafx.h"
#include "AbstractFactory.h"
AbstractFactory::AbstractFactory()
{
}
AbstractFactory::~AbstractFactory()
{
}
//DELLFactory.h
#include "AbstractFactory.h"
class DELLFactory : public AbstractFactory
{
public:
DELLFactory();
virtual ~DELLFactory();
DesktopProduct* CreateDesktopProduct();
NotebookProduct* CreateNotebookProduct();
};
//DELLFactory.cpp
#include "stdafx.h"
#include "DELLFactory.h"
#include "DELLDesktopProduct.h"
#include "DELLNotebookProduct.h"
DELLFactory::DELLFactory()
{
}
DELLFactory::~DELLFactory()
{
}
DesktopProduct* DELLFactory::CreateDesktopProduct()
{
return new DELLDesktopProduct;
}
NotebookProduct* DELLFactory::CreateNotebookProduct()
{
return new DELLNotebookProduct;
}
//IBMFactory.h
#include "AbstractFactory.h"
class IBMFactory : public AbstractFactory
{
public:
IBMFactory();
virtual ~IBMFactory();
DesktopProduct* CreateDesktopProduct();
NotebookProduct* CreateNotebookProduct();
};
//IBMFactory.cpp
#include "stdafx.h"
#include "IBMFactory.h"
#include "IBMDesktopProduct.h"
#include "IBMNotebookProduct.h"
IBMFactory::IBMFactory()
{
}
IBMFactory::~IBMFactory()
{
}
DesktopProduct* IBMFactory::CreateDesktopProduct()
{
return new IBMDesktopProduct;
}
NotebookProduct* IBMFactory::CreateNotebookProduct()
{
return new IBMNotebookProduct;
}
//Main.cpp
#include "stdafx.h"
#include "AbstractFactory.h"
#include "DELLFactory.h"
#include "IBMFactory.h"
#include "DesktopProduct.h"
#include "DELLDeskProduct.h"
#include "IBMDesktopProduct.h"
#include "NotebookProduct.h"
#include "DELLNotebookProduct.h"
#include "IBMNotebookProduct.h"
int main(int argc, char* argv[])
{
AbstractFactory* fac = new DELLFactory();
fac->CreateDesktopProduct();
fac->CreateNotebookProduct();
delete fac;
fac = new IBMFactory();
fac->CreateDesktopProduct();
fac->CreateNotebookProduct();
delete fac;
return 0;
}
最后输出为:
创建DELL台式机
创建DELL笔记本
创建IBM台式机
创建IBM笔记本