刚开始学习Luabind,所以算是一些简单的笔记。
使用Luabind前要包含相关的头文件,引入luabind命名空间。注意包含luabind.hpp并不会自动包含lua相关头文件,要根据需要自己添加。
#include <luabind/luabind.hpp>
extern "C"
{
#include <lua.h>
#include <lualib.h>
}
using namespace luabind;
假设有以下类定义:
1 // TestClass.h
2 class TestClass
3 {
4 public:
5
6 TestClass(string s);
7
8 static TestClass* Singleton();
9
10 void Print();
11
12 private:
13
14 static TestClass* mSingleton;
15
16 string mString;
17 };
18
19 // TestClass.cpp
20 TestClass* TestClass::mSingleton = NULL;
21
22 TestClass::TestClass(string s)
23 {
24 mString = s;
25 }
26
27 TestClass* TestClass::Singleton()
28 {
29 if (TestClass::mSingleton == NULL)
30 {
31 return new TestClass("Hello");
32 }
33 else
34 {
35 return mSingleton;
36 }
37 }
38
39 void TestClass::Print()
40 {
41 cout << mString << endl;
42 }
创建一个bindClass函数,用来进行导出类的相关工作
1 int bindClass(lua_State* L)
2 {
3 open(L);
4
5 module(L)
6 [
7 class_<TestClass>("TestClass")
8 .def(constructor<string>())
9 .def("Print", &TestClass::Print),
10 def("Singleton", TestClass::Singleton) // 请注意static成员函数Singleton()导出时和非静态成员函数的写法区别,
// 和全局函数的导出写法一样。
11 ];
12
13 return 0;
14 }
def
模版类中定义导出函数时,成员函数指针一定要用取地址符&,
如TestClass::Print()。
而自由函数和静态函数可用可不用,如TestClass::Singleton()。
现在就可以写代码测试了:
// test.lua
1 testClass = Singleton()
2 testClass:Print()
// main.cpp
1 int _tmain(int argc, _TCHAR* argv[])
2 {
3 TestClass testClass("Hello from lua.");
4
5 lua_State* L = luaL_newstate();
6
7 init(L);
8
9 luaL_dofile(L, "add.lua");
10
11 lua_close(L);
12
13 return 0;
14 }
15
运行结果:
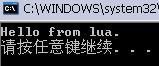