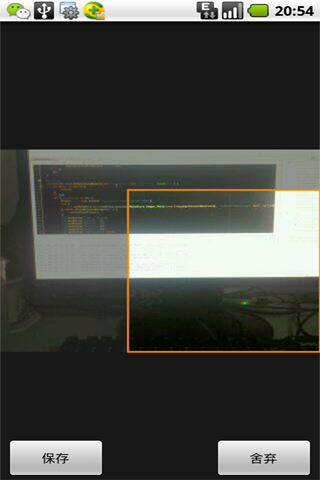
003 | import java.io.ByteArrayOutputStream; |
005 | import android.app.Activity; |
006 | import android.content.Intent; |
007 | import android.graphics.Bitmap; |
008 | import android.net.Uri; |
009 | import android.os.Bundle; |
010 | import android.os.Environment; |
011 | import android.provider.MediaStore; |
012 | import android.view.View; |
013 | import android.view.View.OnClickListener; |
014 | import android.widget.Button; |
015 | import android.widget.ImageView; |
017 | public class testActivity extends Activity { |
019 | public static final int NONE = 0 ; |
020 | public static final int PHOTOHRAPH = 1 ; // 拍照 |
021 | public static final int PHOTOZOOM = 2 ; // 缩放 |
022 | public static final int PHOTORESOULT = 3 ; // 结果 |
024 | public static final String IMAGE_UNSPECIFIED = "image/*" ; |
025 | ImageView imageView = null ; |
026 | Button button0 = null ; |
027 | Button button1 = null ; |
030 | public void onCreate(Bundle savedInstanceState) { |
031 | super .onCreate(savedInstanceState); |
032 | setContentView(R.layout.main); |
033 | imageView = (ImageView) findViewById(R.id.imageID); |
034 | button0 = (Button) findViewById(R.id.btn_01); |
035 | button1 = (Button) findViewById(R.id.btn_02); |
037 | button0.setOnClickListener( new OnClickListener() { |
039 | public void onClick(View v) { |
040 | Intent intent = new Intent(Intent.ACTION_PICK, null ); |
041 | intent.setDataAndType(MediaStore.Images.Media.EXTERNAL_CONTENT_URI, IMAGE_UNSPECIFIED); |
042 | startActivityForResult(intent, PHOTOZOOM); |
046 | button1.setOnClickListener( new OnClickListener() { |
049 | public void onClick(View v) { |
050 | Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); |
051 | intent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile( new File(Environment.getExternalStorageDirectory(), "temp.jpg" ))); |
052 | startActivityForResult(intent, PHOTOHRAPH); |
058 | protected void onActivityResult( int requestCode, int resultCode, Intent data) { |
059 | if (resultCode == NONE) |
062 | if (requestCode == PHOTOHRAPH) { |
064 | File picture = new File(Environment.getExternalStorageDirectory() + "/temp.jpg" ); |
065 | startPhotoZoom(Uri.fromFile(picture)); |
072 | if (requestCode == PHOTOZOOM) { |
073 | startPhotoZoom(data.getData()); |
076 | if (requestCode == PHOTORESOULT) { |
077 | Bundle extras = data.getExtras(); |
078 | if (extras != null ) { |
079 | Bitmap photo = extras.getParcelable( "data" ); |
080 | ByteArrayOutputStream stream = new ByteArrayOutputStream(); |
081 | photo.compress(Bitmap.CompressFormat.JPEG, 75 , stream); // (0 - 100)压缩文件 |
082 | imageView.setImageBitmap(photo); |
087 | super .onActivityResult(requestCode, resultCode, data); |
090 | public void startPhotoZoom(Uri uri) { |
091 | Intent intent = new Intent( "com.android.camera.action.CROP" ); |
092 | intent.setDataAndType(uri, IMAGE_UNSPECIFIED); |
093 | intent.putExtra( "crop" , "true" ); |
094 | // aspectX aspectY 是宽高的比例 |
095 | intent.putExtra( "aspectX" , 1 ); |
096 | intent.putExtra( "aspectY" , 1 ); |
097 | // outputX outputY 是裁剪图片宽高 |
098 | intent.putExtra( "outputX" , 64 ); |
099 | intent.putExtra( "outputY" , 64 ); |
100 | intent.putExtra( "return-data" , true ); |
101 | startActivityForResult(intent, PHOTORESOULT); |
01 | <? xml version = "1.0" encoding = "utf-8" ?> |
02 | < LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android" |
03 | android:orientation = "vertical" android:layout_width = "fill_parent" |
04 | android:layout_height = "fill_parent" > |
05 | < TextView android:layout_width = "fill_parent" |
06 | android:layout_height = "wrap_content" android:text = "@string/hello" /> |
07 | < ImageView android:id = "@+id/imageID" |
08 | android:adjustViewBounds = "true" android:maxWidth = "50dip" |
09 | android:maxHeight = "50dip" android:layout_width = "wrap_content" |
10 | android:layout_height = "wrap_content" /> |
11 | < Button android:id = "@+id/btn_01" android:layout_height = "50dip" |
12 | android:text = "相册" android:layout_width = "150dip" /> |
13 | < Button android:id = "@+id/btn_02" android:layout_height = "50dip" |
14 | android:text = "拍照" android:layout_width = "150dip" /> |
posted on 2012-03-15 12:12
小果子 阅读(479)
评论(0) 编辑 收藏 引用