11.4 外接体(Bounding Volumes)
有时我们需要计算mesh的外接体(边界范围),常用的有两种类型:立方体和球。也有使用其它方法的,如圆柱体,椭球体,菱形体,胶囊形。图11.4演示了对同一个mesh分别使用立方体和球体类型。
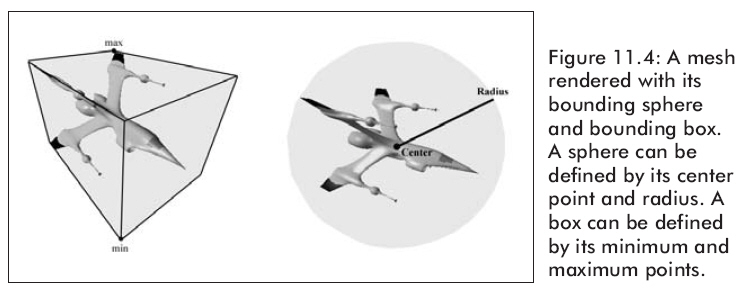
边界盒/球常常被用来加速可见性测试,碰撞检测等。假如一个mesh的边界盒/球不可见,那么我们就说mesh不可见。一个盒/球可见性测试是比分别测试 mesh中的每个三角形要廉价的多。对于一个碰撞检测例子,如果一枚导弹点火起飞,我们需要检测它是否击中了同一场景中的目标。由于这些物体都是由大量三角形构成,我们可以依次检测每个对象的每个三角形,来测试导弹(可以用射线数学模型)是否碰撞到了这些三角形。这个方法需要进行多次的射线/三角形交点的运算。一个更好的方法是使用边界盒或边界球,计算射线与场景中的每个对象的边界盒/边界球的交点。如果射线与对象的边界范围相交,可以认为该对象被击中了。这是一个公平的近似方法,如果需要更高的精度,可以用边界范围法先去除那些明显不会相撞的对象,然后用更精确地方法检测很可能相撞的对象。如果边界范围检测发现相撞,则该对象就很有可能相撞。
D3DX库提供了计算mesh的边界盒和边界球的函数。这些函数使用顶点数组作为输入计算边界盒/球。这些函数本来就是设计的很灵活的,它们可以使用各种顶点格式:
HRESULT D3DXComputeBoundingSphere(
LPD3DXVECTOR3 pFirstPosition,
DWORD NumVertices,
DWORD dwStride,
D3DXVECTOR3* pCenter,
FLOAT* pRadius
);
|
pFirstPosition——指向在顶点数组中第一个顶点的向量,描述顶点位置。
NumVertices——在顶点数组中的的顶点数。
dwStride——每个顶点的字节大小。这很重要,因为顶点结构可能有一些额外信息如法向量和纹理坐标,函数需要知道应该跳过多少字节来得到下一个顶点的位置。
pCenter——返回边界球的中心。
pRadius——返回边界球的半径。
HRESULT D3DXComputeBoundingBox(
LPD3DXVECTOR3 pFirstPosition,
DWORD NumVertices,
DWORD dwStride,
D3DXVECTOR3* pMin,
D3DXVECTOR3* pMax
);
|
前三个参数和D3DXComputeBoundingSphere的前三个参数是完全一样的。最后两个参数分别用来返回边界盒的最小和最大点。< /p>
11.4.1一些新的特殊常量
const float INFINITY = FLT_MAX;
const float EPSILON = 0.001f;
常量INFINITY是用来表示一个浮点数所能存储的最大数。因为我们找不到一个比FLT_MAX还要大的浮点数,我们可以将它视为无穷大。常量 EPSILON是一个很小的值,我们这样定义它,凡是比它小的数就视为0。这也是很有必要的,因为得到的浮点是不精确的。因此,让它和0比较相等肯定会失败。我们因此可以通过把该值与0的差值与EPSILON比较来确定是否相等:
bool Equals(float lhs, float rhs)
{
// if lhs == rhs their difference should be zero
return fabs(lhs - rhs) < EPSILON ? true : false;
}
|
11.4.2外接体类型
为了更容易的使用边界盒和边界球,我们将它们分别封装到两个类中。
class cBoundingBox
{
public:
D3DXVECTOR3 m_min, m_max;
cBoundingBox();
bool is_point_inside(D3DXVECTOR3& point);
};
class cBoundingSphere
{
public:
D3DXVECTOR3 m_center;
float m_radius;
cBoundingSphere();
};
cBoundingBox::cBoundingBox()
{
m_min.x = INFINITY;
m_min.y = INFINITY;
m_min.z = INFINITY;
m_max.x = -INFINITY;
m_max.y = -INFINITY;
m_max.z = -INFINITY;
}
bool cBoundingBox::is_point_inside(D3DXVECTOR3 &point)
{
return (point.x >= m_min.x && point.y >= m_min.y && point.z >= m_min.z &&
point.x <= m_max.x && point.y <= m_max.y && point.z <= m_max.z);
}
cBoundingSphere::cBoundingSphere()
{
m_radius = 0.0f;
}
11.4.3实例程序:外接体
该实例程序主要演示使用D3DXComputeBoundingSphere和 D3DXComputeBoundingBox。程序读取一个X文件并且计算该mesh的边界球,它创建两个ID3DXMesh对象,一个用来作为边界球模型一个用来作为边界盒模型(如下图所示)。你能够通过敲空格键在边界球和边界盒之间切换。
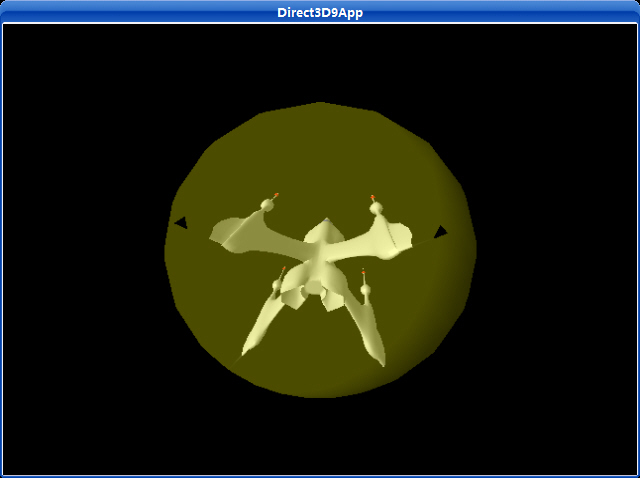
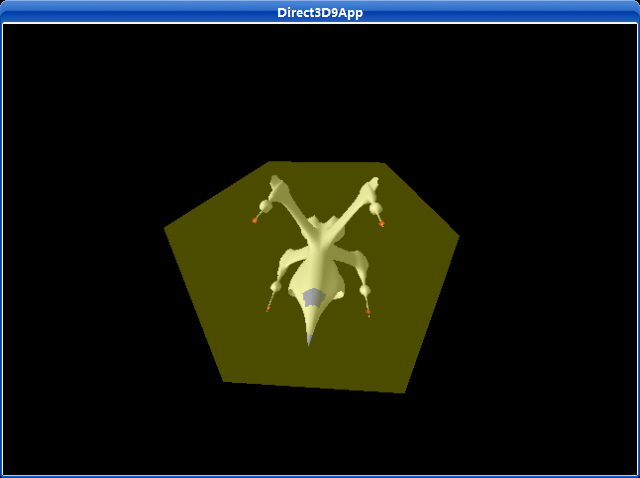
主程序:
/**************************************************************************************
Demonstrates how to use D3DXComputeBoundingSphere and D3DXComputeBoundingBox.
The spacebar key switches between rendering the mesh's bounding sphere and box.
**************************************************************************************/
#include <vector>
#include "d3dUtility.h"
#pragma warning(disable : 4100)
using namespace std;
const int WIDTH = 640;
const int HEIGHT = 480;
IDirect3DDevice9* g_device;
ID3DXMesh* g_mesh;
ID3DXMesh* g_sphere_mesh;
ID3DXMesh* g_box_mesh;
vector<D3DMATERIAL9> g_materials;
vector<IDirect3DTexture9*> g_textures;
bool g_is_render_bounding_sphere = true;
bool compute_bounding_sphere(ID3DXMesh* mesh, cBoundingSphere* sphere);
bool compute_bounding_box(ID3DXMesh* mesh, cBoundingBox* box);
////////////////////////////////////////////////////////////////////////////////////////////////////
bool setup()
{
// load the XFile data
ID3DXBuffer* adjacency_buffer = NULL;
ID3DXBuffer* material_buffer = NULL;
DWORD num_material = 0;
HRESULT hr = D3DXLoadMeshFromX("bigship1.x", D3DXMESH_MANAGED, g_device, &adjacency_buffer, &material_buffer,
NULL, &num_material, &g_mesh);
if(FAILED(hr))
{
MessageBox(NULL, "D3DXLoadMeshFromX() - FAILED", "ERROR", MB_OK);
return false;
}
// extract the materials, and load textures.
if(material_buffer != NULL && num_material != 0)
{
D3DXMATERIAL* materials = (D3DXMATERIAL*) material_buffer->GetBufferPointer();
for(DWORD i = 0; i < num_material; i++)
{
// the MatD3D property doesn't have an ambient value set when it load, so set it now.
materials[i].MatD3D.Ambient = materials[i].MatD3D.Diffuse;
// save the ith material
g_materials.push_back(materials[i].MatD3D);
// check if the ith material has an associative texture
if(materials[i].pTextureFilename != NULL)
{
// yes, load the texture for the ith subset.
IDirect3DTexture9* texture;
D3DXCreateTextureFromFile(g_device, materials[i].pTextureFilename, &texture);
// save the loaded texture
g_textures.push_back(texture);
}
else
{
// no texture for the ith subset
g_textures.push_back(NULL);
}
}
}
safe_release<ID3DXBuffer*>(material_buffer);
// optimize the mesh
hr = g_mesh->OptimizeInplace(D3DXMESHOPT_ATTRSORT | D3DXMESHOPT_COMPACT | D3DXMESHOPT_VERTEXCACHE,
(DWORD*) adjacency_buffer->GetBufferPointer(), NULL, NULL, NULL);
safe_release<ID3DXBuffer*>(adjacency_buffer);
if(FAILED(hr))
{
MessageBox(NULL, "OptimizeInplace() - FAILED", "ERROR", MB_OK);
return false;
}
// compute bounding sphere and bounding box
cBoundingSphere bounding_sphere;
cBoundingBox bounding_box;
compute_bounding_sphere(g_mesh, &bounding_sphere);
compute_bounding_box(g_mesh, &bounding_box);
D3DXCreateSphere(g_device, bounding_sphere.m_radius, 20, 20, &g_sphere_mesh, NULL);
float width = bounding_box.m_max.x - bounding_box.m_min.x;
float height = bounding_box.m_max.y - bounding_box.m_min.y;
float depth = bounding_box.m_max.z - bounding_box.m_min.z;
D3DXCreateBox(g_device, width, height, depth, &g_box_mesh, NULL);
// set texture filters
g_device->SetSamplerState(0, D3DSAMP_MAGFILTER, D3DTEXF_LINEAR);
g_device->SetSamplerState(0, D3DSAMP_MINFILTER, D3DTEXF_LINEAR);
g_device->SetSamplerState(0, D3DSAMP_MIPFILTER, D3DTEXF_LINEAR);
// set lights
D3DXVECTOR3 dir(1.0f, -1.0f, 1.0f);
D3DXCOLOR color(1.0f, 1.0f, 1.0f, 1.0f);
D3DLIGHT9 light = init_directional_light(&dir, &color);
g_device->SetLight(0, &light);
g_device->LightEnable(0, TRUE);
g_device->SetRenderState(D3DRS_NORMALIZENORMALS, TRUE);
g_device->SetRenderState(D3DRS_SPECULARENABLE, TRUE);
// set camera
D3DXVECTOR3 pos(4.0f, 12.0f, -20.0f);
D3DXVECTOR3 target(0.0f, 0.0f, 0.0f);
D3DXVECTOR3 up(0.0f, 1.0f, 0.0f);
D3DXMATRIX view_matrix;
D3DXMatrixLookAtLH(&view_matrix, &pos, &target, &up);
g_device->SetTransform(D3DTS_VIEW, &view_matrix);
// set the projection matrix
D3DXMATRIX proj;
D3DXMatrixPerspectiveFovLH(&proj, D3DX_PI * 0.5f, (float)WIDTH/HEIGHT, 1.0f, 1000.0f);
g_device->SetTransform(D3DTS_PROJECTION, &proj);
return true;
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
void cleanup()
{
safe_release<ID3DXMesh*>(g_mesh);
safe_release<ID3DXMesh*>(g_sphere_mesh);
safe_release<ID3DXMesh*>(g_box_mesh);
for(DWORD i = 0; i < g_textures.size(); i++)
safe_release<IDirect3DTexture9*>(g_textures[i]);
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
bool display(float time_delta)
{
// update: rotate the mesh
static float y = 0.0f;
D3DXMATRIX y_rot_matrix;
D3DXMatrixRotationY(&y_rot_matrix, y);
g_device->SetTransform(D3DTS_WORLD, &y_rot_matrix);
y += time_delta;
if(y >= 6.28f)
y = 0.0f;
// render now
g_device->Clear(0, NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0x00000000, 1.0f, 0);
g_device->BeginScene();
for(DWORD i = 0; i < g_materials.size(); i++)
{
g_device->SetMaterial(&g_materials[i]);
g_device->SetTexture(0, g_textures[i]);
g_mesh->DrawSubset(i);
}
// draw bounding volume in yellow and at 30% opacity
D3DMATERIAL9 blue_material = YELLOW_MATERIAL;
blue_material.Diffuse.a = 0.30f; // 30% opacity
g_device->SetMaterial(&blue_material);
g_device->SetRenderState(D3DRS_ALPHABLENDENABLE, TRUE);
g_device->SetRenderState(D3DRS_SRCBLEND, D3DBLEND_SRCALPHA);
g_device->SetRenderState(D3DRS_DESTBLEND, D3DBLEND_INVSRCALPHA);
if(g_is_render_bounding_sphere)
g_sphere_mesh->DrawSubset(0);
else
g_box_mesh->DrawSubset(0);
g_device->SetRenderState(D3DRS_ALPHABLENDENABLE, FALSE);
g_device->EndScene();
g_device->Present(NULL, NULL, NULL, NULL);
return true;
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
LRESULT CALLBACK wnd_proc(HWND hwnd, UINT msg, WPARAM word_param, LPARAM long_param)
{
switch(msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_KEYDOWN:
if(word_param == VK_ESCAPE)
DestroyWindow(hwnd);
if(word_param == VK_SPACE)
g_is_render_bounding_sphere = !g_is_render_bounding_sphere;
break;
}
return DefWindowProc(hwnd, msg, word_param, long_param);
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
int WINAPI WinMain(HINSTANCE inst, HINSTANCE, PSTR cmd_line, int cmd_show)
{
if(! init_d3d(inst, WIDTH, HEIGHT, true, D3DDEVTYPE_HAL, &g_device))
{
MessageBox(NULL, "init_d3d() - failed.", 0, MB_OK);
return 0;
}
if(! setup())
{
MessageBox(NULL, "Steup() - failed.", 0, MB_OK);
return 0;
}
enter_msg_loop(display);
cleanup();
g_device->Release();
return 0;
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
bool compute_bounding_sphere(ID3DXMesh* mesh, cBoundingSphere* sphere)
{
BYTE* v;
mesh->LockVertexBuffer(0, (void**)&v);
HRESULT hr = D3DXComputeBoundingSphere((D3DXVECTOR3*)v, mesh->GetNumVertices(),
D3DXGetFVFVertexSize(mesh->GetFVF()), &sphere->m_center, &sphere->m_radius);
mesh->UnlockVertexBuffer();
if(FAILED(hr))
return false;
return true;
}
bool compute_bounding_box(ID3DXMesh* mesh, cBoundingBox* box)
{
BYTE* v;
mesh->LockVertexBuffer(0, (void**)&v);
HRESULT hr = D3DXComputeBoundingBox((D3DXVECTOR3*)v, mesh->GetNumVertices(),
D3DXGetFVFVertexSize(mesh->GetFVF()), &box->m_min, &box->m_max);
mesh->UnlockVertexBuffer();
if(FAILED(hr))
return false;
return true;
}
下载源代码