Loading Meshes from .X
Now that you've got a firm grip on how the .X file format works, consider how
Microsoft first intended for you to use it−to contain 3D mesh information for
your games. The D3DX library comes with a number of functions you can use to
load mesh data from an .X file. With the addition of the .X parser developed in
this chapter, you have even more options available to you. Check out just how
easy it is to work with D3DX to load mesh data.
Loading Meshes Using D3DX
The D3DX library defines the handy ID3DXMesh object that contains and renders
3D meshes for you. Although you can use your own custom mesh storage containers,
I find sticking to the ID3DXMesh object perfectly sensible. That's the object
I'll use for the rest of this chapter (with the exception of the also−handy
ID3DXSkinMesh object, which you'll see in a moment).
The fastest way to load mesh data using D3DX is to call on the
D3DXLoadMeshFromX and D3DXLoadMeshFromXof functions. Both of these meshes will
take the data contained within an .X file and convert it to an ID3DXMesh object.
The D3DXLoadMeshFromX file loads an entire .X file at once (compressing all
meshes into a single output mesh), whereas the D3DXLoadMeshFromXof function
loads a single mesh pointed at by an IDirectXFileData object.
The D3DXLoadMeshFromX function provides a file name to load, some flags to
control loading aspects, a 3D device pointer, pointers to buffers for containing
material data, and some miscellaneous data pointers that you can ignore for now.
Loads a mesh from a DirectX .x file.
HRESULT D3DXLoadMeshFromX(
LPCTSTR pFilename,
DWORD Options,
LPDIRECT3DDEVICE9 pD3DDevice,
LPD3DXBUFFER * ppAdjacency,
LPD3DXBUFFER * ppMaterials,
LPD3DXBUFFER * ppEffectInstances,
DWORD * pNumMaterials,
LPD3DXMESH * ppMesh
);
Parameters
- pFilename
- [in] Pointer to a string that specifies the
filename. If the compiler settings require Unicode, the data type LPCTSTR
resolves to LPCWSTR. Otherwise, the string data type resolves to LPCSTR. See
Remarks.
- Options
- [in] Combination of one or more flags from the
D3DXMESH enumeration, which specifies creation options for the mesh.
- pD3DDevice
- [in] Pointer to an IDirect3DDevice9 interface, the
device object associated with the mesh.
- ppAdjacency
- [out] Pointer to a buffer that contains adjacency
data. The adjacency data contains an array of three DWORDs per face that
specify the three neighbors for each face in the mesh. For more information
about accessing the buffer, see ID3DXBuffer.
- ppMaterials
- [out] Pointer to a buffer containing materials
data. The buffer contains an array of D3DXMATERIAL structures, containing
information from the DirectX file. For more information about accessing the
buffer, see ID3DXBuffer.
- ppEffectInstances
- [out] Pointer to a buffer containing an array of
effect instances, one per attribute group in the returned mesh. An effect
instance is a particular instance of state information used to initialize an
effect. See D3DXEFFECTINSTANCE. For more information about accessing the
buffer, see ID3DXBuffer.
- pNumMaterials
- [out] Pointer to the number of D3DXMATERIAL
structures in the ppMaterials array, when the method returns.
- ppMesh
- [out] Address of a pointer to an ID3DXMesh
interface, representing the loaded mesh.
Return Values
If the function succeeds, the return value is D3D_OK.
If the function fails, the return value can be one of the following values:
D3DERR_INVALIDCALL, E_OUTOFMEMORY.
Remarks
The compiler setting also determines the function
version. If Unicode is defined, the function call resolves to
D3DXLoadMeshFromXW. Otherwise, the function call resolves to D3DXLoadMeshFromXA
because ANSI strings are being used.
All the meshes in the file will be collapsed into one
output mesh. If the file contains a frame hierarchy, all the transformations
will be applied to the mesh.
For mesh files that do not contain effect instance
information, default effect instances will be generated from the material
information in the .x file. A default effect instance will have default values
that correspond to the members of the D3DMATERIAL9 structure.
The default texture name is also filled in, but is
handled differently. The name will be Texture0@Name, which corresponds to an
effect variable by the name of "Texture0" with an annotation called "Name." This
will contain the string file name for the texture.
First, you need to instance an ID3DXMesh object.
ID3DXMesh *Mesh = NULL;
From there, suppose you want to load the .X file called test.x. Simple
enough−you just need to specify the file name in the call to D3DXLoadMeshFromX
but wait! What's with the Options parameter? You use it to tell D3DX how to load
the mesh−into system memory or video memory, using write−only memory, and so on.
A flag represents each option. Table 3.3 lists some of the most common macros.
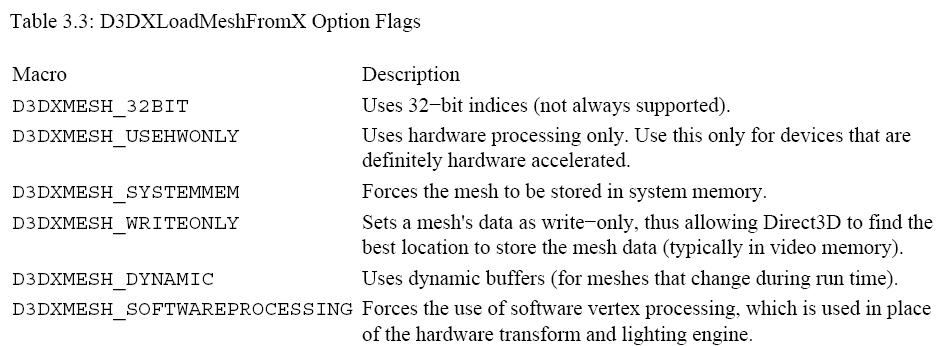
From Table 3.3, you can see there are really not many options for loading
meshes. In fact, I recommend using only D3DXMESH_SYSTEMMEM or D3DXMESH_WRITEONLY
The first option, D3DXMESH_SYSTEMMEM, forces your mesh's data to be stored in
system memory, making access to the mesh data faster for both read and write
operations.
Specifying D3DXMESH_DYNAMIC means you are going to change the mesh data
periodically. It's best to specify this flag if you plan to periodically change
the mesh's data (such as vertices) in any way during run time.
If speed is your need, then I suggest using the D3DXMESH_WRITEONLY flag,
which tells D3DX to use memory that will not allow read access. Most often that
means you will use video memory because it is usually (but not always)
write−only. If you're not going to read a mesh's vertex data, then I suggest
using this flag.
Tip If you're not using system memory or write−only access, what's
left to use? Just specify a value of 0 for Options in the call to
D3DXLoadMeshFromX, and you'll be fine.
Getting back to the parameters of D3DXLoadMeshFromX, you'll see the pointer
to a 3D device interface. No problem−you should have one of those hanging around
in your project! Next is the ID3DXBUFFER pointer, ppAdjacency. Set that to
NULL−you won't be using it here.
The next three parameters, ppMaterials, ppEffectInstance, and pNumMaterials,
contain the mesh's material information, such as material color values and
texture file names, as well as effects data. If you're using DirectX 8, you can
safely exclude the ppEffectInstance reference−it doesn't exist in that version.
If you're using DirectX 9, you can set ppEffectInstance to NULL because you
don't require any effects information.
The ppMaterials pointer points to an ID3DXBuffer interface, which is merely a
container for data. pNumMaterials is a pointer to a DWORD variable that will
contain the number of materials in a mesh that was loaded. You'll see how to use
material information in a moment.
Finishing up with D3DXLoadMeshFromX, you see the actual ID3DXMesh object
pointer−ppMesh. This is the interface you supply to contain your newly loaded
mesh data. And there you have it! Now put all of this stuff together into a
working example of loading a mesh.
Load a mesh, again called test.x, using write−only memory. After you've
instanced the mesh object pointer, you need to instance an ID3DXBuffer object to
contain material data and a DWORD variable to contain the number of materials.
ID3DXBuffer *pMaterials = NULL;
DWORD NumMaterials;
From here, call D3DXLoadMeshFromX.
// pDevice = pointer to a valid IDirect3DDevice9 object
D3DXLoadMeshFromX("test.x", D3DXMESH_WRITEONLY, pDevice, NULL, &pMaterials,
NULL, &NumMaterials, &Mesh);
Great! If everything went as expected, D3DXLoadMeshFromX will return a
success code, and your mesh will have been loaded in the ID3DXMesh interface
Mesh! Of course, every single mesh contained in the .X file was crunched into a
single mesh object, so how about those times when you want access to each
separately−defined mesh in the file?
This is where the D3DXLoadMeshFromXof file comes in. You use the
D3DXLoadMeshFromXof function in conjunction with your .X parser to load mesh
data from an enumerated Mesh object. Just take a look at the D3DXLoadMeshFromXof
function prototype to see what this entails.
Loads a mesh from an ID3DXFileData object.
HRESULT D3DXLoadMeshFromXof(
LPD3DXFILEDATA pxofMesh,
DWORD Options,
LPDIRECT3DDEVICE9 pDevice,
LPD3DXBUFFER * ppAdjacency,
LPD3DXBUFFER * ppMaterials,
LPD3DXBUFFER * ppEffectInstances,
DWORD * pNumMaterials,
LPD3DXMESH * ppMesh
);
Parameters
- pxofMesh
- [in] Pointer to an ID3DXFileData interface,
representing the file data object to load.
- Options
- [out] Combination of one or more flags from the
D3DXMESH enumeration, specifying creation options for the mesh.
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, the
device object associated with the mesh.
- ppAdjacency
- [out] Pointer to a buffer that contains adjacency
data. The adjacency data contains an array of three DWORDs per face that
specify the three neighbors for each face in the mesh. For more information
about accessing the buffer, see ID3DXBuffer.
- ppMaterials
- [in, out] Address of a pointer to an
ID3DXBuffer interface. When the method returns, this parameter is filled
with an array of D3DXMATERIAL structures.
- ppEffectInstances
- [out] Pointer to a buffer containing an array of
effect instances, one per attribute group in the returned mesh. An effect
instance is a particular instance of state information used to initialize an
effect. See D3DXEFFECTINSTANCE. For more information about accessing the
buffer, see ID3DXBuffer.
- pNumMaterials
- [in, out] Pointer to the number of D3DXMATERIAL
structures in the ppMaterials array, when the method returns.
- ppMesh
- [out, retval] Address of a pointer to an ID3DXMesh
interface, representing the loaded mesh.
Return Values
If the function succeeds, the return value is D3D_OK.
If the function fails, the return value can be one of the following:
D3DERR_INVALIDCALL.
D3DXERR_INVALIDDATA E_OUTOFMEMORY
Remarks
For mesh files that do not contain effect instance
information, default effect instances will be generated from the material
information in the .x file. A default effect instance will have default values
that correspond to the members of the D3DMATERIAL9 structure.
The default texture name is also filled in, but is
handled differently. The name will be Texture0@Name, which corresponds to an
effect variable by the name of "Texture0" with an annotation called "Name." This
will contain the string file name for the texture.
Now wait a sec! D3DXLoadMeshFromXof looks almost exactly like
D3DXLoadMeshFromX! The only difference is the first parameter; instead of a
pointer to an .X file name to load, D3DXLoadMeshFromXof has a pointer to an
ID3DXFileData object. By providing the pointer to a currently enumerated
ID3DXFileData object, D3DX will take over and load all of the appropriate mesh
data for you! And
since every other parameter is the same as in D3DXLoadMeshFromX, you'll have no
trouble using D3DXLoadMeshFromXof in your .X parser class!
Regardless of which function you use to load the mesh data (D3DXLoadMeshFromX
or D3DXLoadMeshFromXof), all you have left to do is process the material
information once a mesh has been loaded into an ID3DXMesh object.
To process the material information, you need to retrieve a pointer to the
material's ID3DXBuffer's data buffer (used in the call to D3DXLoadMeshFromX or
D3DXLoadMeshFromXof) and cast it to a D3DXMATERIAL type. From there, iterate all
materials, using the number of materials set in NumMaterials as your index. Then
you need to instance an array of D3DMATERIAL9 structures and IDirect3DTexture9
interfaces to contain the mesh's material data. Use the following code to
process the material information:
// Objects to hold material and texture data
D3DMATERIAL9 *Materials = NULL;
IDirect3DTexture9 *Textures = NULL;
// Get a pointer to the material data
D3DXMATERIAL *pMat;
pMat = (D3DXMATERIAL*)pMaterials−>GetBufferPointer();
// Allocate material storage space
if(NumMaterials)
{
Materials = new D3DMATERIAL9[NumMaterials];
Textures = new IDirect3DTexture9*[NumMaterials];
// Iterate through all loaded materials
for(DWORD i=0;i<NumMaterials;i++)
{
// Copy over the material information
Materials[i] = pMat[i].MatD3D;
// Copy diffuse color over to ambient color
Materials[i].Ambient = Materials[i].Diffuse;
// Load a texture if one is specified
Textures[i] = NULL;
if(pMat[i].pTextureFilename)
D3DXCreateTextureFromFile(pDevice, pMat[i].pTextureFilename, &Textures[i]);
}
}
else
{
// Allocate a default material if none were loaded
Materials = new D3DMATERIAL9[1];
Textures = new IDirect3DTexture9*[1];
// Set a default white material and no texture
Textures[0] = NULL;
ZeroMemory(&Materials[0], sizeof(D3DMATERIAL9));
Materials[0].Diffuse.r = Materials[0].Ambient.r = 1.0f;
Materials[0].Diffuse.g = Materials[0].Ambient.g = 1.0f;
Materials[0].Diffuse.b = Materials[0].Ambient.b = 1.0f;
Materials[0].Diffuse.a = Materials[0].Ambient.a = 1.0f;
}
// Free material data buffer
pMaterials−>Release();
You can see in the preceding code that I added a case to check whether no
materials were loaded, in which case you need to create a default material to
use. Once those materials are loaded, you're ready to begin using the mesh
interface to render.