Loading Frame Hierarchies from .X
Skeletal animation systems require a frame hierarchy (which represents the
bone structure) to orient each bone during rendering. The .X file format defines
a frame−of−reference data template that you can use to define your bone
hierarchy. This template, Frame, is merely a placeholder of sorts. It allows any
type of data object to be embedded in it so you can reference the instanced
Frame object by its assigned instance name and allow all contained objects to be
addressed as well.
Building a frame hierarchy involves parsing an .X file, in which you link
each Frame object to another. The relationship is very important−a Frame object
can either be a sibling or a child to another Frame object, and it can have an
unlimited number of siblings and/or child objects linked to it.
In DirectX 9, you have access to a special DirectX structure called D3DXFRAME
to contain each frame in your hierarchy. In this section, you'll use D3DXFRAME
to contain a frame hierarchy.
The exact way you parse and build your Frame objects is really up to you. You
can use the D3DX library to help, or you can parse/build the hierarchy yourself
using your custom .X parser. Which is better for you? Whereas the D3DX library
is great to use, I find the methods of using the library to load a frame
hierarchy overly complicated, with no less than two interfaces to use and an
entire memory−handling class of your own to be written. Why bother when you can
load a frame hierarchy using one small .X parser class you created?
Instead, load up your trusty .X parser (which you developed earlier in this
chapter, in the "Constructing an .X Parser Class" section) and derive a version
that scans for Frame data objects. I'll start you off by showing you the derived
class you can use.
class cXFrameParser : public cXParser
{
public:
// declare an extended version of D3DXFRAME
// that contains a constructor and destructor
struct D3DXFRAME_EX : public D3DXFRAME
{
D3DXFRAME_EX()
{
Name = NULL;
pFrameSibling = NULL; pFrameFirstChild = NULL;
}
~D3DXFRAME_EX()
{
delete [] Name;
delete pFrameFirstChild;
delete pFrameSibling;
}
} D3DXFRAME_EX;
// Instance the root frame of the hierarchy
D3DXFRAME_EX *m_RootFrame;
public:
cXFrameParser() { m_RootFrame = NULL; }
~cXFrameParser() { delete m_RootFrame; }
BOOL BeginParse(void **Data)
{
// Clear hierarchy
delete m_RootFrame; m_RootFrame = NULL;
}
BOOL ParseObject(IDirectXFileData *pDataObj,
IDirectXFileData *pParentDataObj,
DWORD Depth,
void **Data,
BOOL Reference)
{
// Skip reference frames
if(Reference == TRUE)
return TRUE;
// Make sure template being parsed is a frame
if(*GetObjectGUID(pDataObj) == TID_D3DRMFrame)
{
// Allocate a frame structure
D3DXFRAME_EX *Frame = new D3DXFRAME_EX();
// Get frame name (assign one if none found)
if((Frame−>Name = GetObjectName(pDataObj)) == NULL)
Frame−>Name = strdup("No Name");
// Link frame structure into list
if(Data == NULL)
{
// Link as sibling of root
Frame−>pFrameSibling = m_RootFrame;
m_RootFrame = Frame;
Data = (void**)&m_RootFrame;
}
else
{
// Link as child of supplied frame
D3DXFRAME_EX *FramePtr = (D3DXFAME_EX*)*Data;
Frame−>pFrameSibling = FramePtr−>pFrameFirstChild;
FramePtr−>pFrameFirstChild = Frame;
Data = (void**)&Frame;
}
}
return ParseChildObjects(pDataObj,Depth,Data,Reference);
}
};
cXFrameParser Parser;
Parser.Parse("frames.x");
// Parser.m_RootFrame now points to root frame of hierarchy
There you have it. Once the cXFrameParser::Parse function is complete, you'll
have a self−contained frame hierarchy ready to use in your project. The
ParseFrame demo loads an .X file of your choice and displays the frame hierarchy
inside a list box.
ParseFrame Demo
This demo uses the information on loading a frame hierarchy from an .X file.
As shown in Figure 3.2, the ParseFrame demo has a button labeled Select .X File.
Click that button, locate an .X file to load, and click Open.
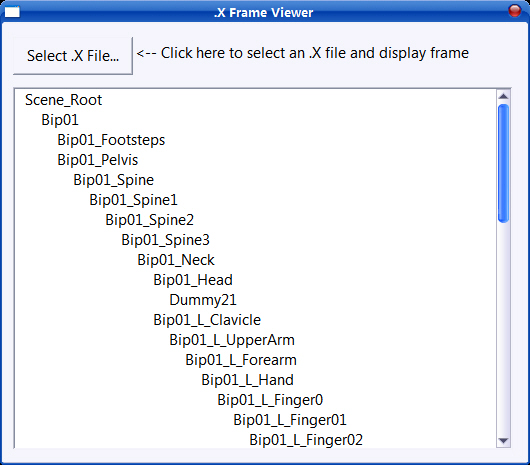
Figure 3.2: The ParseFrame demo's dialog box contains two controls−a button
you click to load an .X file and a list box that displays the frame hierarchy.
After selecting an .X file to load and clicking Open, you'll be treated to a
display of the frame hierarchy contained within the file. To make things easier
to understand, the frames are indented by their level in the hierarchy.
Because IDirectXFile interface has been deprecated, I
translate this interface into ID3DXFile.
WinMain.cpp:
#include <stdio.h>
#include <windows.h>
#include <d3d9.h>
#include <d3dx9.h>
#include <rmxfguid.h>
#include "Direct3D.h"
#include "XParser.h"
#include "resource.h"
#pragma warning(disable : 4996)
extern unsigned char D3DRM_XTEMPLATES[];
D3DXFRAME_EX* g_root_frame;
const char g_class_name[] = "ParseFrameClass";
const char g_caption[] = ".X Frame Parser Demo";
////////////////////////////////////////////////////////////////////////////////////////////////
LRESULT FAR PASCAL window_proc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam);
bool do_init(HWND hwnd);
void do_shutdown();
void do_frame();
void add_frames_to_list(D3DXFRAME_EX* frame, DWORD indent, HWND list_handle);
//////////////////////////////////////////////////////////////////////////////////////////////
class cXFrameParser : public cXParser
{
protected:
D3DXFRAME_EX* m_root_frame;
protected:
virtual bool parse_objects(ID3DXFileData* xfile_data,
ID3DXFileData* parent_xfile_data,
DWORD depth,
void** data,
bool force_ref)
{
GUID type;
get_object_guid(xfile_data, &type);
// make sure template being parsed is a mesh (non-referenced)
if(type == TID_D3DRMFrame && force_ref == false)
{
D3DXFRAME_EX* frame = new D3DXFRAME_EX;
// get frame name (assign one if none found)
frame->Name = get_object_name(xfile_data);
if(frame->Name == NULL)
frame->Name = strdup("NoNameFrame");
// link frame structure into list
if(data == NULL)
{
// link as sibling of root
frame->pFrameSibling = m_root_frame;
m_root_frame = frame;
data = (void**) &m_root_frame;
}
else
{
// link as child of supplied frame
D3DXFRAME_EX* frame_ptr = (D3DXFRAME_EX*) (*data);
frame->pFrameSibling = frame_ptr->pFrameFirstChild;
frame_ptr->pFrameFirstChild = frame;
data = (void**) &frame_ptr->pFrameFirstChild;
}
frame = NULL; // clear frame pointer since it's been assigned
}
return parse_child_objects(xfile_data, depth, data, force_ref);
}
public:
bool load(const char* filename, D3DXFRAME_EX** ret_frame)
{
bool rv = false;
m_root_frame = NULL;
if(parse(filename, NULL))
{
*ret_frame = m_root_frame;
rv = true;
}
else
{
*ret_frame = NULL;
}
return rv;
}
};
//////////////////////////////////////////////////////////////////////////////////////////////
int PASCAL WinMain(HINSTANCE inst, HINSTANCE, LPSTR, int cmd_show)
{
CoInitialize(NULL); // Initialize the COM system
// Create the window class here and register it
WNDCLASSEX win_class;
win_class.cbSize = sizeof(win_class);
win_class.style = CS_HREDRAW | CS_VREDRAW;
win_class.lpfnWndProc = window_proc;
win_class.cbClsExtra = 0;
win_class.cbWndExtra = DLGWINDOWEXTRA;
win_class.hInstance = inst;
win_class.hIcon = LoadIcon(NULL, IDI_APPLICATION);
win_class.hCursor = LoadCursor(NULL, IDC_ARROW);
win_class.hbrBackground = (HBRUSH)(COLOR_BTNFACE + 1);
win_class.lpszMenuName = NULL;
win_class.lpszClassName = g_class_name;
win_class.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
if(!RegisterClassEx(&win_class))
return -1;
// create the dialog box window and show it
HWND hwnd = CreateDialog(inst, MAKEINTRESOURCE(IDD_FRAMEVIEW), 0, NULL);
ShowWindow(hwnd, cmd_show);
UpdateWindow(hwnd);
MSG msg;
ZeroMemory(&msg, sizeof(MSG));
// Start message pump, waiting for user to exit
while(msg.message != WM_QUIT)
{
if(PeekMessage(&msg, NULL, 0, 0, PM_REMOVE))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
}
UnregisterClass(g_class_name, inst);
CoUninitialize();
return 0;
}
LRESULT FAR PASCAL window_proc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
OPENFILENAME ofn;
char filename[MAX_PATH] = {0};
// Only handle window destruction messages
switch(msg)
{
case WM_COMMAND:
switch(LOWORD(wParam))
{
case IDC_SELECT:
ZeroMemory(&ofn, sizeof(OPENFILENAME));
ofn.lStructSize = sizeof(OPENFILENAME);
ofn.nMaxFile = MAX_PATH;
ofn.nMaxFileTitle = MAX_PATH;
ofn.Flags = OFN_HIDEREADONLY | OFN_CREATEPROMPT | OFN_OVERWRITEPROMPT;
ofn.hwndOwner = hwnd;
ofn.lpstrFile = filename;
ofn.lpstrTitle = "Load and Parse .x file";
ofn.lpstrFilter = ".X Files (*.x)\0*.x\0All Files (*.*)\0*.*\0\0";
ofn.lpstrDefExt = "x";
if(! GetOpenFileName(&ofn))
return 0;
// get rid of last loaded mesh list
delete g_root_frame;
g_root_frame = NULL;
// parse .x file and display hierarchy
cXFrameParser mesh_parser;
if(mesh_parser.load(filename, &g_root_frame))
{
SendMessage(GetDlgItem(hwnd, IDC_FRAMELIST), LB_RESETCONTENT, 0, 0);
add_frames_to_list(g_root_frame, 2, GetDlgItem(hwnd, IDC_FRAMELIST));
}
break;
}
break;
case WM_DESTROY:
delete g_root_frame;
g_root_frame = NULL;
PostQuitMessage(0);
break;
case WM_KEYDOWN:
if(wParam == VK_ESCAPE)
DestroyWindow(hwnd);
break;
}
return DefWindowProc(hwnd, msg, wParam, lParam);
}
void add_frames_to_list(D3DXFRAME_EX* frame, DWORD indent, HWND list_handle)
{
if(frame == NULL)
return;
// add siblings to list first
if(frame->pFrameSibling)
add_frames_to_list((D3DXFRAME_EX*) frame->pFrameSibling, indent, list_handle);
// build text to add to list
char text[1024];
memset(text, ' ', indent);
text[indent] = '\0';
strcat(text, frame->Name);
SendMessage(list_handle, LB_ADDSTRING, 0, (LPARAM) text);
// add children to list
if(frame->pFrameFirstChild)
add_frames_to_list((D3DXFRAME_EX*) frame->pFrameFirstChild, indent+4, list_handle);
}
download
source file
Loading Custom Data from .X
As I've expressed throughout this chapter, the .X file format is completely
open−ended; there is no limit to the type of data you can store. With that in
mind, you can create any type of data storage you want, and accessing that data
will be just as easy as accessing the mesh and frame data we've already covered.
Jump back to your ContactEntry template and see just how to parse an .X file
and display every instance of ContactEntry. Again, a single small derived class
of cXParser will work perfectly here.
#include "initguid.h"
// First declare the ContactEntry GUID
DEFINE_GUID(ContactEntry, \
0x4c9d055b, 0xc64d, 0x4bfe, 0xa7, 0xd9, 0x98, \
0x1f, 0x50, 0x7e, 0x45, 0xff);
// Now, define the .X parser class
class cXContactParser : public cXParser
{
public:
BOOL ParseObject(IDirectXFileData *pDataObj,
IDirectXFileData *pParentDataObj,
DWORD Depth,
void **Data,
BOOL Reference)
{
// Skip reference objects
if(Reference == TRUE)
return TRUE;
// Make sure object being parsed is ContactEntry
if(*GetObjectGUID(pDataObj) == CONTACTENTRY)
{
// Get the data pointer and size
DWORD DataSize;
DWORD *DataPtr;
DataPtr = (DWORD*)GetObjectData(pDataObj, &DataSize);
// Get name from object data
char *Name = (char*)*DataPtr++;
// Get phone # from object data
char *PhoneNum = (char*)*DataPtr++;
// Get age from object data
DWORD Age = *DataPtr++;
// Display contact information
char Text[1024];
sprintf(Text, "Name: %s\r\nPhone #: %s\r\nAge: %lu", Name, PhoneNum, Age);
MessageBox(NULL, Text, "Contact", MB_OK);
}
return ParseChildObjects(pDataObj,Depth,Data,Reference);
}
};
cXContactParser Parser;
Parser.Parse("contacts.x");
With a single call to cXContactParser::Parse, you're treated to a list of
people's names, phone numbers, and ages−all contained in the contacts.x file!
Now wasn't that easy? See, you don't have to be afraid of the .X file format. I
personally use .X to store as much custom data as I can.