ACE中的流包装提供面向连接的通信。流数据传输包装类包括ACE_SOCK_Stream和ACE_LSOCK_Stream,它们分别包装TCP/IP和UNIX域socket协议数据传输功能。连接建立类包括针对TCP/IP的ACE_SOCK_Connector和ACE_SOCK_Acceptor,以及针对UNIX域socket的ACE_LSOCK_Connector和ACE_LSOCK_Acceptor。
Acceptor类用于被动地接受连接(使用BSD accept()调用),而Connector类用于主动地建立连接(使用BSD connect()调用)。
下面的例子演示接收器和连接器是怎样用于建立连接的。该连接随后将用于使用流数据传输类来传输数据。
server端代码:
// 环境VC6.0+ACE5.4.2
// proj:ACE stream server
// date:07-7-24
// robin
//
#include "stdafx.h"
#include "ace/Log_Msg.h"
#include "ace/Time_Value.h"
#include "ace/OS.h"
#include "ace/SOCK_Acceptor.h"
#include "ace/SOCK_Stream.h"
#define SIZE_DATA 18
#define SIZE_BUF 1024
#define NO_ITERATIONS 5
class Server
{
public:
Server (int port) : server_addr_(port),peer_acceptor_(server_addr_)
{
data_buf_ = new char[SIZE_BUF];
}
//Handle the connection once it has been established. Here the
//connection is handled by reading SIZE_DATA amount of data from the
//remote and then closing the connection stream down.
int handle_connection()
{
// Read data from client
for(int i=0;i<NO_ITERATIONS;i++)
{
int byte_count=0;
if( (byte_count = new_stream_.recv_n(data_buf_, SIZE_DATA, 0) ) == -1 )
ACE_ERROR ((LM_ERROR, "%p\n", "Error in recv"));
else
{
data_buf_[byte_count]=0;
ACE_DEBUG((LM_DEBUG,"Server received %s \n",data_buf_));
}
}
// Close new endpoint
if (new_stream_.close () == -1)
ACE_ERROR ((LM_ERROR, "%p\n", "close"));
return 0;
}
//Use the acceptor component peer_acceptor_ to accept the connection
//into the underlying stream new_stream_. After the connection has been
//established call the handle_connection() method.
int accept_connections ()
{
if (peer_acceptor_.get_local_addr (server_addr_) == -1)
ACE_ERROR_RETURN ((LM_ERROR,"%p\n","Error in get_local_addr"),1);
ACE_DEBUG ((LM_DEBUG,"Starting server at port %d\n",
server_addr_.get_port_number ()));
// Performs the iterative server activities.
while(1)
{
ACE_Time_Value timeout (ACE_DEFAULT_TIMEOUT);
if (peer_acceptor_.accept(new_stream_, &client_addr_, &timeout)== -1)
{
ACE_ERROR ((LM_ERROR, "%p\n", "accept"));
continue;
}
else
{
ACE_DEBUG((LM_DEBUG,
"Connection established with remote %s:%d\n",
client_addr_.get_host_name(),client_addr_.get_port_number()));
//Handle the connection
handle_connection();
}
}
}
private:
char *data_buf_;
ACE_INET_Addr server_addr_;
ACE_INET_Addr client_addr_;
ACE_SOCK_Acceptor peer_acceptor_;
ACE_SOCK_Stream new_stream_;
};
int main (int argc, char *argv[])
{
if(argc<2)
{
ACE_ERROR((LM_ERROR,"Usage %s <port_num>", argv[0]));
ACE_OS::exit(1);
}
// char *ip;
// ip = new char[strlen("192.168.1.160")];
// Server server(ACE_OS::atoi(ip)); //argv[1])
Server server(ACE_OS::atoi(argv[1]));
server.accept_connections();
return 0;
}
client端:
// proj:ACE stream client
// client
// date:7-24
// robin
#include "stdafx.h"
//******additional*******//
#include "ace/Log_Msg.h" //ACE_ERROR ACE_DEBUG
#include "ace/Time_Value.h" // ACE_Time_Value
#include "ace/OS.h" // ACE_OS::atoi exit
//******additional*******//
#include "ace/SOCK_Connector.h"
#include "ace/INET_Addr.h"
#define SIZE_BUF 128
#define NO_ITERATIONS 5
class Client
{
public:
Client(char *hostname, int port):remote_addr_(port,hostname)
{
data_buf_="Hello from Client";
}
//Uses a connector component `connector_’ to connect to a
//remote machine and pass the connection into a stream
//component client_stream_
int connect_to_server()
{
// Initiate blocking connection with server.
ACE_DEBUG ((LM_DEBUG, "(%P|%t) Starting connect to %s:%d\n",
remote_addr_.get_host_name(),remote_addr_.get_port_number()));
if (connector_.connect (client_stream_, remote_addr_) == -1)
ACE_ERROR_RETURN ((LM_ERROR,"(%P|%t) %p\n","connection failed"),-1);
else
ACE_DEBUG ((LM_DEBUG,"(%P|%t) connected to %s\n",
remote_addr_.get_host_name ()));
return 0;
}
//Uses a stream component to send data to the remote host.
int send_to_server()
{
// Send data to server
for(int i=0;i<NO_ITERATIONS; i++)
{
if (client_stream_.send_n (data_buf_,
ACE_OS::strlen(data_buf_)+1, 0) == -1)
{
ACE_ERROR_RETURN ((LM_ERROR,"(%P|%t) %p\n","send_n"),0);
break;
}
}
//Close down the connection
close();
}
//Close down the connection properly.
int close()
{
if (client_stream_.close () == -1)
ACE_ERROR_RETURN ((LM_ERROR,"(%P|%t) %p\n","close"),-1);
else
return 0;
}
private:
ACE_SOCK_Stream client_stream_;
ACE_INET_Addr remote_addr_;
ACE_SOCK_Connector connector_;
char *data_buf_;
};
int main(int argc, char* argv[])
{
if(argc<3)
{
ACE_DEBUG((LM_DEBUG,"Usage %s <hostname> <port_number>\n", argv[0]));
ACE_OS::exit(1);
}
Client client(argv[1],ACE_OS::atoi(argv[2]));
client.connect_to_server();
client.send_to_server();
return 0;
}
运行结果:
cmd里到exe目录下,先启动服务端server.exe 192.168.1.160
如图1:

正在轮询等待。
再到client服务端,同样的方法
运行命令行参数格式 client.exe 192.168.1.160 192
解析:ACE_DEBUG((LM_DEBUG,"Usage %s <hostname> <port_number>\n", argv[0]));
命令行参数为 <hostname><port> = <192.168.1.160><192>
端口是由图1的第二行显示,不是自己设定的。
如图2:
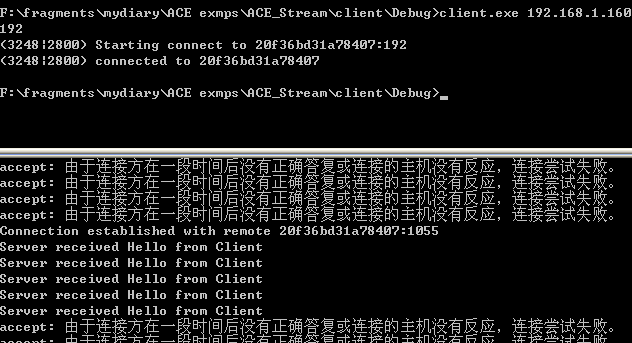
只是照搬书上的例子,后面打算自己分析一下。