强制性引导UI控件,关键区域镂空,自动调整关键区域大小。
效果如图:
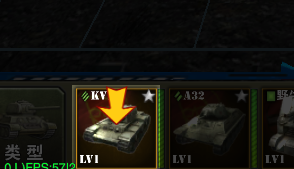
使用结构:
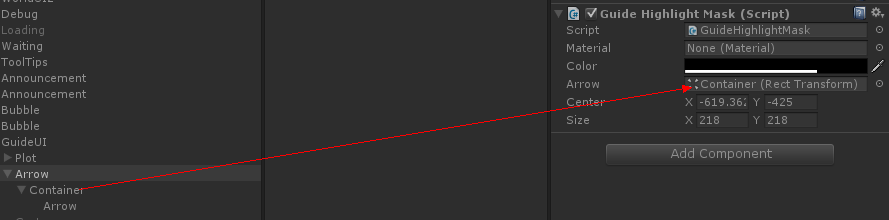
代
1
using UnityEngine;
2
using System.Collections;
3
using UnityEngine.UI;
4
5
using System.Collections.Generic;
6
using UnityEngine.Serialization;
7
8
namespace UnityEngine.UI
9

{
10
11
public class GuideHighlightMask : MaskableGraphic, UnityEngine.ICanvasRaycastFilter
12
{
13
public RectTransform arrow;
14
public Vector2 center = Vector2.zero;
15
public Vector2 size = new Vector2(100, 100);
16
17
public void DoUpdate()
18
{
19
// 当引导箭头位置或者大小改变后更新,注意:未处理拉伸模式
20
if (arrow && center != arrow.anchoredPosition || size != arrow.sizeDelta)
21
{
22
this.center = arrow.anchoredPosition;
23
this.size = arrow.sizeDelta;
24
SetAllDirty();
25
}
26
}
27
28
public bool IsRaycastLocationValid(Vector2 sp, Camera eventCamera)
29
{
30
// 点击在箭头框内部则无效,否则生效
31
return !RectTransformUtility.RectangleContainsScreenPoint(arrow, sp, eventCamera);
32
}
33
34
protected override void OnFillVBO(List<UIVertex> vbo)
35
{
36
Vector4 outer = new Vector4(-rectTransform.pivot.x * rectTransform.rect.width,
37
-rectTransform.pivot.y * rectTransform.rect.height,
38
(1 - rectTransform.pivot.x) * rectTransform.rect.width,
39
(1 - rectTransform.pivot.y) * rectTransform.rect.height);
40
41
Vector4 inner = new Vector4(center.x - size.x / 2,
42
center.y - size.y / 2,
43
center.x + size.x * 0.5f,
44
center.y + size.y * 0.5f);
45
46
vbo.Clear();
47
48
var vert = UIVertex.simpleVert;
49
50
// left
51
vert.position = new Vector2(outer.x, outer.y);
52
vert.color = color;
53
vbo.Add(vert);
54
55
vert.position = new Vector2(outer.x, outer.w);
56
vert.color = color;
57
vbo.Add(vert);
58
59
vert.position = new Vector2(inner.x, outer.w);
60
vert.color = color;
61
vbo.Add(vert);
62
63
vert.position = new Vector2(inner.x, outer.y);
64
vert.color = color;
65
vbo.Add(vert);
66
67
// top
68
vert.position = new Vector2(inner.x, inner.w);
69
vert.color = color;
70
vbo.Add(vert);
71
72
vert.position = new Vector2(inner.x, outer.w);
73
vert.color = color;
74
vbo.Add(vert);
75
76
vert.position = new Vector2(inner.z, outer.w);
77
vert.color = color;
78
vbo.Add(vert);
79
80
vert.position = new Vector2(inner.z, inner.w);
81
vert.color = color;
82
vbo.Add(vert);
83
84
// right
85
vert.position = new Vector2(inner.z, outer.y);
86
vert.color = color;
87
vbo.Add(vert);
88
89
vert.position = new Vector2(inner.z, outer.w);
90
vert.color = color;
91
vbo.Add(vert);
92
93
vert.position = new Vector2(outer.z, outer.w);
94
vert.color = color;
95
vbo.Add(vert);
96
97
vert.position = new Vector2(outer.z, outer.y);
98
vert.color = color;
99
vbo.Add(vert);
100
101
// bottom
102
vert.position = new Vector2(inner.x, outer.y);
103
vert.color = color;
104
vbo.Add(vert);
105
106
vert.position = new Vector2(inner.x, inner.y);
107
vert.color = color;
108
vbo.Add(vert);
109
110
vert.position = new Vector2(inner.z, inner.y);
111
vert.color = color;
112
vbo.Add(vert);
113
114
vert.position = new Vector2(inner.z, outer.y);
115
vert.color = color;
116
vbo.Add(vert);
117
}
118
119
private void Update()
120
{
121
DoUpdate();
122
}
123
}
124
}
125
码: