来自于《大话设计模式》
命令模式(Command):将一个请求封装为一个对象,从而使你可用不同的请求对客户进行参数化;对请求排队或记录请求日志,以及支持可撤销的操作。
行为型
UML 类图:
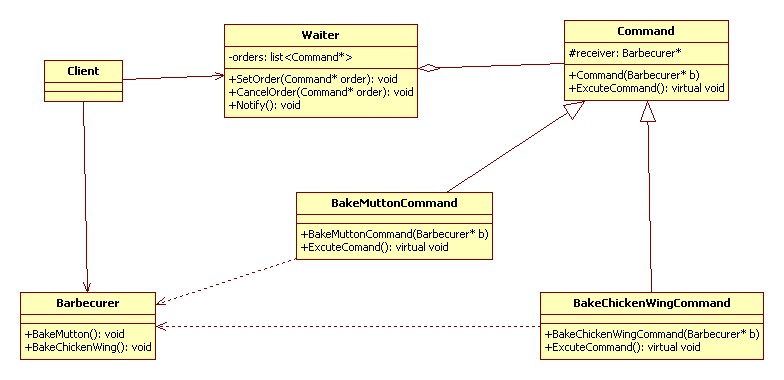
代码实现 C++:
1 #include <iostream>
2 #include <list>
3 #include <algorithm>
4 using namespace std;
5
6 class Barbecurer
7 {
8 public:
9 void BakeMutton()
10 {
11 cout << "烤羊肉串!" << endl;
12 }
13 void BakeChickenWing()
14 {
15 cout << "烤鸡翅!" << endl;
16 }
17 };
18
19 class Command
20 {
21 protected:
22 Barbecurer* receiver;
23 public:
24 Command(Barbecurer* b)
25 {
26 receiver = b;
27 }
28 virtual void ExcuteCommand() = 0;
29 };
30
31 class BakeMuttonCommand : public Command
32 {
33 public:
34 BakeMuttonCommand(Barbecurer* b) : Command(b) {}
35 virtual void ExcuteCommand()
36 {
37 receiver->BakeMutton();
38 }
39 };
40
41 class BakeChickenWingCommand : public Command
42 {
43 public:
44 BakeChickenWingCommand(Barbecurer* b) : Command(b) {}
45 virtual void ExcuteCommand()
46 {
47 receiver->BakeChickenWing();
48 }
49 };
50
51 class Waiter
52 {
53 private:
54 list<Command*> orders;
55 public:
56 void SetOrder(Command* order)
57 {
58 cout << "增加订单!" << endl;
59 orders.push_back(order);
60 }
61 void CancelOrder(Command* order)
62 {
63 list<Command*>::iterator iter = find(orders.begin(), orders.end(), order);
64 if (iter != orders.end())
65 {
66 cout << "取消订单!" << endl;
67 orders.erase(iter);
68 }
69 }
70 void Notify()
71 {
72 for (list<Command*>::iterator iter = orders.begin(); iter != orders.end(); ++iter)
73 {
74 (*iter)->ExcuteCommand();
75 }
76 }
77 };
78
79 int main()
80 {
81 Barbecurer* boy = new Barbecurer;
82
83 Command* bakeMuttonCommand1 = new BakeMuttonCommand(boy);
84 Command* bakeMuttonCommand2 = new BakeMuttonCommand(boy);
85 Command* bakeChickenWingCommand1 = new BakeChickenWingCommand(boy);
86
87 Waiter* girl = new Waiter;
88
89 girl->SetOrder(bakeMuttonCommand1);
90 girl->SetOrder(bakeMuttonCommand2);
91 girl->SetOrder(bakeChickenWingCommand1);
92
93 girl->Notify();
94
95
96 girl->CancelOrder(bakeMuttonCommand2);
97 girl->Notify();
98
99 return 0;
100 }
posted on 2011-04-29 21:15
unixfy 阅读(370)
评论(0) 编辑 收藏 引用