来自于《大话设计模式》
解释器模式(Interpreter):
给定一个语言,定义它的文法的一种表示,并定义一个解释器,这个解释器使用该表示来解释语言中的句子。
行为型
UML 类图:
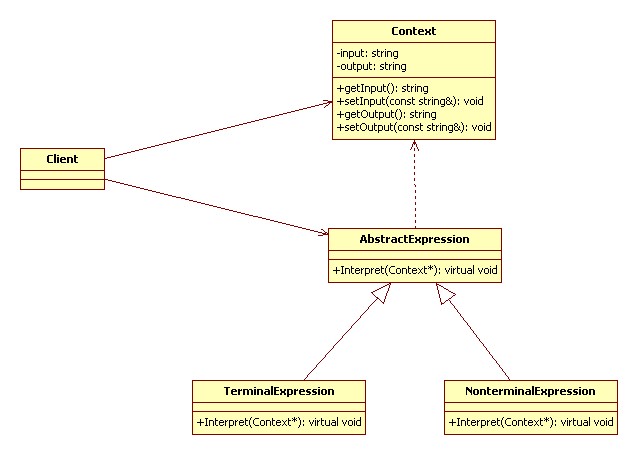
代码实现 C++:
1 #include <iostream>
2 #include <string>
3 #include <list>
4 using namespace std;
5
6 class Context
7 {
8 private:
9 string input;
10 string output;
11 public:
12 string getInput()
13 {
14 return input;
15 }
16 void setInput(const string& s)
17 {
18 input = s;
19 }
20 string getOutput()
21 {
22 return output;
23 }
24 void setOutput(const string& s)
25 {
26 output = s;
27 }
28 };
29
30 class AbstractExpression
31 {
32 public:
33 virtual void Interpret(Context* context) = 0;
34 };
35
36 class TerminalExpression : public AbstractExpression
37 {
38 public:
39 virtual void Interpret(Context* context)
40 {
41 context->setOutput("终端解释器:" + context->getInput());
42 cout << context->getOutput() << endl;
43 }
44 };
45
46 class NonterminalExpression : public AbstractExpression
47 {
48 public:
49 virtual void Interpret(Context* context)
50 {
51 context->setOutput("非终端解释器:" + context->getInput());
52 cout << context->getOutput() << endl;
53 }
54 };
55
56 int main()
57 {
58 Context* context = new Context;
59 context->setInput("abc");
60 list<AbstractExpression*> lae;
61
62 lae.push_back(new TerminalExpression);
63 lae.push_back(new NonterminalExpression);
64 lae.push_back(new TerminalExpression);
65 lae.push_back(new TerminalExpression);
66
67 for (list<AbstractExpression*>::iterator iter = lae.begin(); iter != lae.end(); ++iter)
68 {
69 (*iter)->Interpret(context);
70 }
71
72 for (list<AbstractExpression*>::iterator iter = lae.begin(); iter != lae.end(); ++iter)
73 {
74 delete (*iter);
75 }
76 delete context;
77
78 return 0;
79 }
posted on 2011-04-30 14:32
unixfy 阅读(918)
评论(2) 编辑 收藏 引用