来自于《大话设计模式》
访问者模式(Visitor):
表示一个作用于某对象结构中的个元素的操作。它是你可以在不改变各元素的类的前提下定义作用于这些元素的新操作。
行为型
UML 类图:
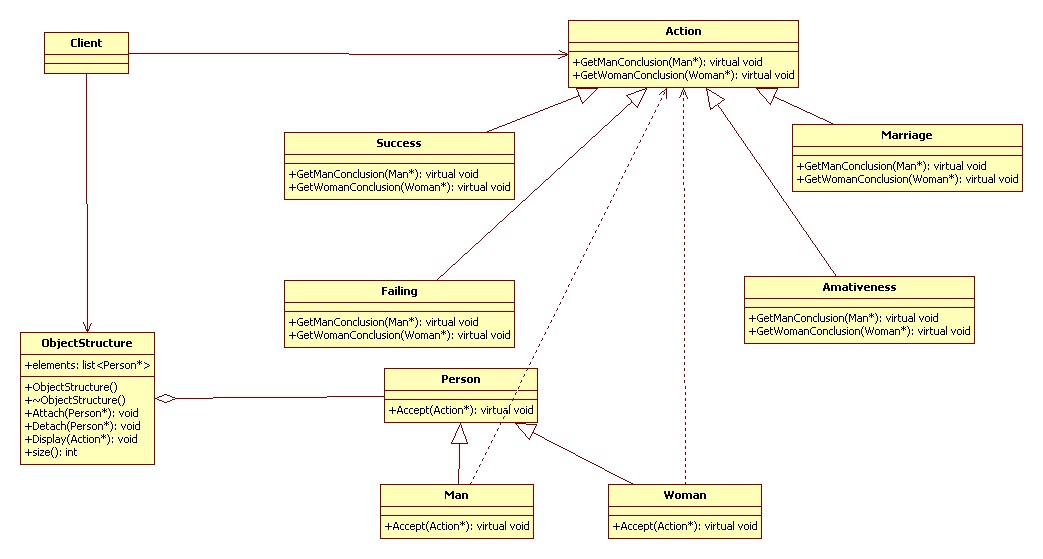
代码实现 C++:
1 #include <iostream>
2 #include <list>
3 #include <algorithm>
4 #include <string>
5 using namespace std;
6
7 class Action;
8
9 class Person
10 {
11 protected:
12 string name;
13 public:
14 Person(const string& s = "Person") : name(s) {}
15 virtual void Accept(Action* visitor) = 0;
16 virtual string getName()
17 {
18 return name;
19 }
20 };
21
22 class Man;
23 class Woman;
24
25 class Action
26 {
27 protected:
28 string name;
29 public:
30 Action(const string& s = "Action") : name(s) {}
31 virtual void GetManConclusion(Man* m) = 0;
32 virtual void GetWomanConclusion(Woman* w) = 0;
33 };
34
35 class Man : public Person
36 {
37 public:
38 Man(const string& s = "Man") : Person(s) {}
39 virtual void Accept(Action* visitor)
40 {
41 visitor->GetManConclusion(this);
42 }
43 };
44
45 class Woman : public Person
46 {
47 public:
48 Woman(const string& s = "Woman") : Person(s) {}
49 virtual void Accept(Action* visitor)
50 {
51 visitor->GetWomanConclusion(this);
52 }
53 };
54
55 class Success : public Action
56 {
57 public:
58 Success(const string& s = "Success") : Action(s) {}
59 virtual void GetManConclusion(Man* m)
60 {
61 cout << name << endl;
62 cout << m->getName() << endl;
63 cout << "1" << endl;
64 }
65 virtual void GetWomanConclusion(Woman* w)
66 {
67 cout << name << endl;
68 cout << w->getName() << endl;
69 cout << "2" << endl;
70 }
71 };
72
73 class Failing : public Action
74 {
75 public:
76 Failing(const string& s = "Failing") : Action(s) {}
77 virtual void GetManConclusion(Man* m)
78 {
79 cout << name << endl;
80 cout << m->getName() << endl;
81 cout << "3" << endl;
82 }
83 virtual void GetWomanConclusion(Woman* w)
84 {
85 cout << name << endl;
86 cout << w->getName() << endl;
87 cout << "4" << endl;
88 }
89 };
90
91 class Amativeness : public Action
92 {
93 public:
94 Amativeness(const string& s = "Amativeness") : Action(s) {}
95 virtual void GetManConclusion(Man* m)
96 {
97 cout << name << endl;
98 cout << m->getName() << endl;
99 cout << "5" << endl;
100 }
101 virtual void GetWomanConclusion(Woman* w)
102 {
103 cout << name << endl;
104 cout << w->getName() << endl;
105 cout << "6" << endl;
106 }
107 };
108
109 class Marriage : public Action
110 {
111 public:
112 Marriage(const string& s = "Marriage") : Action(s) {}
113 virtual void GetManConclusion(Man* m)
114 {
115 cout << name << endl;
116 cout << m->getName() << endl;
117 cout << "7" << endl;
118 }
119 virtual void GetWomanConclusion(Woman* w)
120 {
121 cout << name << endl;
122 cout << w->getName() << endl;
123 cout << "8" << endl;
124 }
125 };
126
127 class ObjectStructure
128 {
129 private:
130 list<Person*> elements;
131 public:
132 ObjectStructure() {}
133 ~ObjectStructure()
134 {
135 for (list<Person*>::iterator iter = elements.begin(); iter != elements.end(); ++iter)
136 {
137 delete (*iter);
138 }
139 }
140 void Attach(Person* element)
141 {
142 elements.push_back(element);
143 }
144 void Detach(Person* element)
145 {
146 list<Person*>::iterator iter = find(elements.begin(), elements.end(), element);
147 if (iter != elements.end())
148 {
149 elements.erase(iter);
150 }
151 }
152 void Display(Action* visitor)
153 {
154 for (list<Person*>::iterator iter = elements.begin(); iter != elements.end(); ++iter)
155 {
156 (*iter)->Accept(visitor);
157 }
158 }
159 int size()
160 {
161 return elements.size();
162 }
163 };
164
165 int main()
166 {
167 ObjectStructure o;
168 o.Attach(new Man);
169 o.Attach(new Woman);
170
171 cout << o.size() << endl;
172
173 Success* v1 = new Success;
174 o.Display(v1);
175 Failing* v2 = new Failing;
176 o.Display(v2);
177 Amativeness* v3 = new Amativeness;
178 o.Display(v3);
179 Marriage* v4 = new Marriage;
180 o.Display(v4);
181
182 delete v4;
183 delete v3;
184 delete v2;
185 delete v1;
186
187 return 0;
188 }
posted on 2011-04-30 15:21
unixfy 阅读(458)
评论(0) 编辑 收藏 引用