封装Common Control Library 6.0的API越来越顺手了。虽说要消灭BEGIN_MESSAGE_MAP之类的代码,不过写起来也不容易。BEGIN_MESSAGE_MAP不能动态替换,所以我换成了类似C#的Event和Delegate那样子的东西。如果不需要动态替换的话,实际上并没有什么大的区别,唯一的区别就在于你可以利用VC++的Intellisense去查看自己想要的事件,而不是将什么WM_LBUTTONDOWN之类的消息记住了。
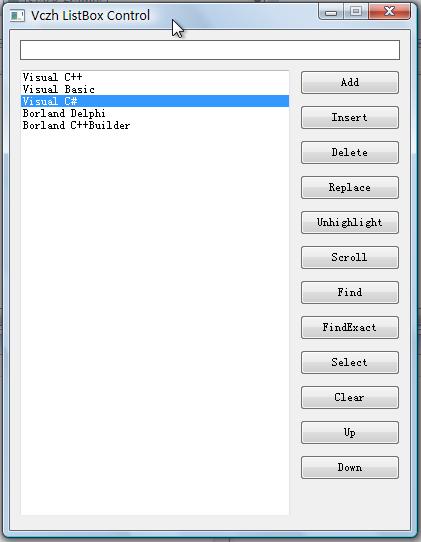
1 #include "..\..\..\..\VL++\Library\Windows\VL_WinMain.h"
2 #include "..\..\..\..\VL++\Library\Windows\Commctrl\VL_WinButton.h"
3 #include "..\..\..\..\VL++\Library\Windows\Commctrl\VL_WinContainers.h"
4 #include "..\..\..\..\VL++\Library\Windows\Commctrl\VL_WinText.h"
5
6 using namespace vl;
7 using namespace vl::windows;
8
9 const VInt _ClientWidth=400;
10 const VInt _ClientHeight=500;
11 const VInt _TextHeight=20;
12 const VInt _ButtonWidth=100;
13 const VInt _ButtonHeight=25;
14 const VInt _Border=10;
15
16 #define MakeButtonInit VInt __ButtonCount=0
17
18 #define MakeButton(Name,Text) \
19 Name=new VL_WinButton(this); \
20 Name->SetLeft(_ClientWidth-_Border-_ButtonWidth); \
21 Name->SetTop(_Border*2+_TextHeight+(_ButtonHeight+_Border)*__ButtonCount++);\
22 Name->SetWidth(_ButtonWidth); \
23 Name->SetHeight(_ButtonHeight); \
24 Name->SetText(Text); \
25 Name->OnClick.Bind(this,&MyForm::##Name##_Click);
26
27 class MyForm : public VL_WinForm
28 {
29 protected:
30 VL_WinEdit* TextBox;
31 VL_WinListBox* ListBox;
32 VL_WinButton* BtnAdd;
33 VL_WinButton* BtnInsert;
34 VL_WinButton* BtnDelete;
35 VL_WinButton* BtnReplace;
36 VL_WinButton* BtnUnhighlight;
37 VL_WinButton* BtnScroll;
38 VL_WinButton* BtnFind;
39 VL_WinButton* BtnFindExact;
40 VL_WinButton* BtnSelect;
41 VL_WinButton* BtnClear;
42 VL_WinButton* BtnUp;
43 VL_WinButton* BtnDown;
44
45 void ShowMessage(VUnicodeString Message)
46 {
47 VL_WinMsgbox(this,Message,GetText(),vmbOK);
48 }
49
50 void InitControls()
51 {
52 TextBox=new VL_WinEdit(this,false);
53 TextBox->SetLeft(_Border);
54 TextBox->SetTop(_Border);
55 TextBox->SetWidth(_ClientWidth-2*_Border);
56 TextBox->SetHeight(_TextHeight);
57
58 ListBox=new VL_WinListBox(this);
59 ListBox->SetLeft(_Border);
60 ListBox->SetTop(_Border*2+_TextHeight);
61 ListBox->SetWidth(_ClientWidth-3*_Border-_ButtonWidth);
62 ListBox->SetHeight(_ClientHeight-_Border*3-_TextHeight);
63
64 MakeButtonInit;
65 MakeButton(BtnAdd,L"Add");
66 MakeButton(BtnInsert,L"Insert");
67 MakeButton(BtnDelete,L"Delete");
68 MakeButton(BtnReplace,L"Replace");
69 MakeButton(BtnUnhighlight,L"Unhighlight");
70 MakeButton(BtnScroll,L"Scroll");
71 MakeButton(BtnFind,L"Find");
72 MakeButton(BtnFindExact,L"FindExact");
73 MakeButton(BtnSelect,L"Select");
74 MakeButton(BtnClear,L"Clear");
75 MakeButton(BtnUp,L"Up");
76 MakeButton(BtnDown,L"Down");
77 }
78
79 void BtnAdd_Click(VL_Base* Sender)
80 {
81 ListBox->AddString(TextBox->GetText());
82 TextBox->SetText(L"");
83 TextBox->SetFocused();
84 }
85
86 void BtnInsert_Click(VL_Base* Sender)
87 {
88 if(ListBox->GetSelectedIndex()==-1)
89 {
90 ShowMessage(L"请在列表中选中一项。");
91 }
92 else
93 {
94 ListBox->InsertString(ListBox->GetSelectedIndex(),TextBox->GetText());
95 TextBox->SetText(L"");
96 TextBox->SetFocused();
97 }
98 }
99
100 void BtnDelete_Click(VL_Base* Sender)
101 {
102 if(ListBox->GetSelectedIndex()==-1)
103 {
104 ShowMessage(L"请在列表中选中一项。");
105 }
106 else
107 {
108 ListBox->DeleteString(ListBox->GetSelectedIndex());
109 TextBox->SetText(L"");
110 TextBox->SetFocused();
111 }
112 }
113
114 void BtnReplace_Click(VL_Base* Sender)
115 {
116 if(ListBox->GetSelectedIndex()==-1)
117 {
118 ShowMessage(L"请在列表中选中一项。");
119 }
120 else
121 {
122 ListBox->SetString(ListBox->GetSelectedIndex(),TextBox->GetText());
123 TextBox->SetText(L"");
124 TextBox->SetFocused();
125 }
126 }
127
128 void BtnUnhighlight_Click(VL_Base* Sender)
129 {
130 if(ListBox->GetSelectedIndex()==-1)
131 {
132 ShowMessage(L"请在列表中选中一项。");
133 }
134 else
135 {
136 ListBox->SetSelected(ListBox->GetSelectedIndex(),false);
137 TextBox->SetText(L"");
138 TextBox->SetFocused();
139 }
140 }
141
142 void BtnScroll_Click(VL_Base* Sender)
143 {
144 if(ListBox->GetSelectedIndex()==-1)
145 {
146 ShowMessage(L"请在列表中选中一项。");
147 }
148 else
149 {
150 ListBox->SetFocusedIndex(ListBox->GetSelectedIndex());
151 TextBox->SetText(L"");
152 TextBox->SetFocused();
153 }
154 }
155
156 void BtnFind_Click(VL_Base* Sender)
157 {
158 VInt Index=ListBox->FindStringPrefix(TextBox->GetText());
159 if(Index!=-1)
160 {
161 ListBox->SetSelectedIndex(Index);
162 ListBox->SetFocusedIndex(Index);
163 }
164 else
165 {
166 ShowMessage(L"找不到。");
167 }
168 TextBox->SetText(L"");
169 TextBox->SetFocused();
170 }
171
172 void BtnFindExact_Click(VL_Base* Sender)
173 {
174 VInt Index=ListBox->FindString(TextBox->GetText());
175 if(Index!=-1)
176 {
177 ListBox->SetSelectedIndex(Index);
178 ListBox->SetFocusedIndex(Index);
179 }
180 else
181 {
182 ShowMessage(L"找不到。");
183 }
184 TextBox->SetText(L"");
185 TextBox->SetFocused();
186 }
187
188 void BtnSelect_Click(VL_Base* Sender)
189 {
190 ListBox->SelectPrefix(TextBox->GetText());
191 TextBox->SetText(L"");
192 TextBox->SetFocused();
193 }
194
195 void BtnClear_Click(VL_Base* Sender)
196 {
197 ListBox->Clear();
198 }
199
200 void BtnUp_Click(VL_Base* Sender)
201 {
202 ListBox->SetSelectedIndex(ListBox->GetSelectedIndex()-1);
203 }
204
205 void BtnDown_Click(VL_Base* Sender)
206 {
207 ListBox->SetSelectedIndex(ListBox->GetSelectedIndex()+1);
208 }
209
210 public:
211
212 MyForm():VL_WinForm(true)
213 {
214 SetBorder(vwfbSingle);
215 SetMaximizeBox(false);
216 SetClientWidth(_ClientWidth);
217 SetClientHeight(_ClientHeight);
218 SetText(L"Vczh ListBox Control");
219 MoveCenter();
220 InitControls();
221 Show();
222 }
223 };
224
225 void main()
226 {
227 new MyForm;
228 GetApplication()->Run();
229 }
posted on 2008-08-03 08:48
陈梓瀚(vczh) 阅读(1964)
评论(8) 编辑 收藏 引用 所属分类:
C++