GacUI在结束了文本框的介绍之后,开始进入列表的介绍。列表内容丰富,包含各种预定义的列表控件、用来显示和操作大量对象的虚拟模式、MVC分离、修改列表样式等内容。今天先从文本列表的简单操作开始。这个Demo展示了如何对列表进行添加和删除。窗口里面有一个列表,然后有添加和删除两个按钮,分别用于把文本框的内容添加到列表内,和删除掉选中的列表项的。在这个Demo里面只允许列表项单选,并且水平滚动条默认不出现。先看图:
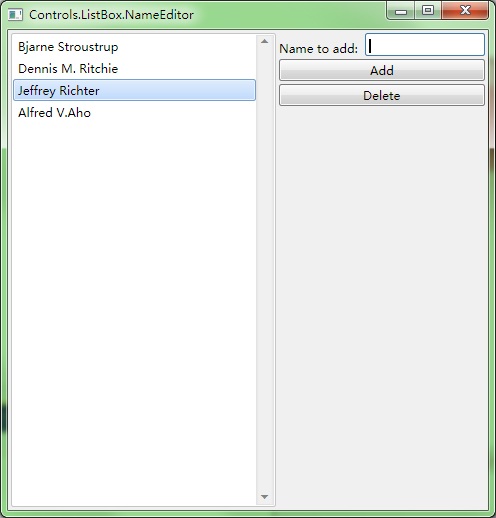
空间如何布局,我就不再赘述了,明显是一个四行三列的表格。代码如下:
#include "..\..\Public\Source\GacUIIncludes.h"
#include <Windows.h>
// for SortedList, CopyFrom and Select
using namespace vl::collections;
int CALLBACK WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int CmdShow)
{
return SetupWindowsDirect2DRenderer();
}
class NameEditorWindow : public GuiWindow
{
private:
GuiTextList* listBox;
GuiSinglelineTextBox* textBox;
GuiButton* buttonAdd;
GuiButton* buttonRemove;
void buttonAdd_Clicked(GuiGraphicsComposition* sender, GuiEventArgs& arguments)
{
// add the specified name at the end of the list box
listBox->GetItems().Add(textBox->GetText());
textBox->SelectAll();
textBox->SetFocus();
}
void buttonRemove_Clicked(GuiGraphicsComposition* sender, GuiEventArgs& arguments)
{
// remove the selected items using item index
listBox->GetItems().RemoveAt(listBox->GetSelectedItems()[0]);
}
void listBox_SelectionChanged(GuiGraphicsComposition* sender, GuiEventArgs& arguments)
{
// disable the button if no item is selected
buttonRemove->SetEnabled(listBox->GetSelectedItems().Count()>0);
}
public:
NameEditorWindow()
:GuiWindow(GetCurrentTheme()->CreateWindowStyle())
{
this->SetText(L"Controls.ListBox.NameEditor");
GuiTableComposition* table=new GuiTableComposition;
table->SetRowsAndColumns(4, 3);
table->SetCellPadding(3);
table->SetAlignmentToParent(Margin(0, 0, 0, 0));
table->SetRowOption(0, GuiCellOption::MinSizeOption());
table->SetRowOption(1, GuiCellOption::MinSizeOption());
table->SetRowOption(2, GuiCellOption::MinSizeOption());
table->SetRowOption(3, GuiCellOption::PercentageOption(1.0));
table->SetColumnOption(0, GuiCellOption::PercentageOption(1.0));
table->SetColumnOption(1, GuiCellOption::MinSizeOption());
table->SetColumnOption(2, GuiCellOption::MinSizeOption());
this->GetContainerComposition()->AddChild(table);
{
GuiCellComposition* cell=new GuiCellComposition;
table->AddChild(cell);
cell->SetSite(0, 0, 4, 1);
listBox=g::NewTextList();
listBox->GetBoundsComposition()->SetAlignmentToParent(Margin(0, 0, 0, 0));
listBox->SetHorizontalAlwaysVisible(false);
listBox->SelectionChanged.AttachMethod(this, &NameEditorWindow::listBox_SelectionChanged);
cell->AddChild(listBox->GetBoundsComposition());
}
{
GuiCellComposition* cell=new GuiCellComposition;
table->AddChild(cell);
cell->SetSite(0, 1, 1, 1);
GuiLabel* label=g::NewLabel();
label->SetText(L"Name to add: ");
label->GetBoundsComposition()->SetAlignmentToParent(Margin(0, -1, 0, 0));
cell->AddChild(label->GetBoundsComposition());
}
{
GuiCellComposition* cell=new GuiCellComposition;
table->AddChild(cell);
cell->SetSite(0, 2, 1, 1);
textBox=g::NewTextBox();
textBox->GetBoundsComposition()->SetPreferredMinSize(Size(120, 23));
textBox->GetBoundsComposition()->SetAlignmentToParent(Margin(0, 0, 0, 0));
cell->AddChild(textBox->GetBoundsComposition());
}
{
GuiCellComposition* cell=new GuiCellComposition;
table->AddChild(cell);
cell->SetSite(1, 1, 1, 2);
buttonAdd=g::NewButton();
buttonAdd->SetText(L"Add");
buttonAdd->GetBoundsComposition()->SetAlignmentToParent(Margin(0, 0, 0, 0));
buttonAdd->Clicked.AttachMethod(this, &NameEditorWindow::buttonAdd_Clicked);
cell->AddChild(buttonAdd->GetBoundsComposition());
}
{
GuiCellComposition* cell=new GuiCellComposition;
table->AddChild(cell);
cell->SetSite(2, 1, 1, 2);
buttonRemove=g::NewButton();
buttonRemove->SetText(L"Delete");
buttonRemove->SetEnabled(false);
buttonRemove->GetBoundsComposition()->SetAlignmentToParent(Margin(0, 0, 0, 0));
buttonRemove->Clicked.AttachMethod(this, &NameEditorWindow::buttonRemove_Clicked);
cell->AddChild(buttonRemove->GetBoundsComposition());
}
// set the preferred minimum client size
this->GetBoundsComposition()->SetPreferredMinSize(Size(480, 480));
// call this to calculate the size immediately if any indirect content in the table changes
// so that the window can calcaulte its correct size before calling the MoveToScreenCenter()
this->ForceCalculateSizeImmediately();
// move to the screen center
this->MoveToScreenCenter();
}
};
void GuiMain()
{
GuiWindow* window=new NameEditorWindow;
GetApplication()->Run(window);
delete window;
}
这里需要注意的几点就是,为了实现在列表没有选中内容的时候禁用删除按钮,我们需要监听GuiTextList::SelectionChanged事件。核心的代码就是下面这几行:
void buttonAdd_Clicked(GuiGraphicsComposition* sender, GuiEventArgs& arguments)
{
// add the specified name at the end of the list box
listBox->GetItems().Add(textBox->GetText());
textBox->SelectAll();
textBox->SetFocus();
}
void buttonRemove_Clicked(GuiGraphicsComposition* sender, GuiEventArgs& arguments)
{
// remove the selected items using item index
listBox->GetItems().RemoveAt(listBox->GetSelectedItems()[0]);
}
void listBox_SelectionChanged(GuiGraphicsComposition* sender, GuiEventArgs& arguments)
{
// disable the button if no item is selected
buttonRemove->SetEnabled(listBox->GetSelectedItems().Count()>0);
}
GuiTextList控件的GetItems函数返回所有的列表项。这个对象有Add、Insert、Clear、IndexOf、Remove、RemoveAt、Contains、Count等函数,可以用来操作列表项。GuiTextList还有GetSelectedItems函数(其实是定义在GuiSelectableListControl里面的),可以用来获得所有选中的列表项的下标(从0开始)。每当列表内容被修改的时候,GetSelectedItems的结果就会被自动清空。
下一个Demo将是关于如何处理允许多选的列表的操作方法。
posted on 2012-05-23 04:42
陈梓瀚(vczh) 阅读(2327)
评论(4) 编辑 收藏 引用 所属分类:
GacUI