事实上,有两种不同的剪贴板接口机制。第一种机制是使用Windows剪贴板API,第二种机制则是使用OLE。以为剪贴板API是迄今为止最常用的方法,一次本章中主要介绍使用Windows剪贴板API。
1、相关函数的介绍
1)为数据分配内存空间:
HGLOBAL GlobalAlloc(UINT uFlags,SIZE_T dwBytes),uFlags参数用来说明Windows如何分配内存(有GHND、GMEM_FIXED(默认值,可以用0或NULL代替)、GMEM_MOVEABLE和GPTR)。参数dwBytes是一个双字节(DWORD)值,用来指定分配的缓冲区的大小。
2)锁定全局内存:LPVOID GlobalLock(HGLOBAL hMem)
3)解除对全局内存的锁定:BOOL GlobalUnlock(HGLOBAL hMem)
4)打开剪贴板:BOOL OpenClipboard(HWND hWndNewOwner)
5)清空剪贴板:BOOL EmptyClipboard()
6)设置剪贴板数据:HANDLE SetClipboardData(UINT uFormat,HANDLE hMem),uFormat值常见的有(CF_BITMAP、CF_TEXT、CF_OMETEXT)
7)关闭剪贴板:BOOL CloseClipboard()
2、示例程序
相关变量没给出,自己看程序创建
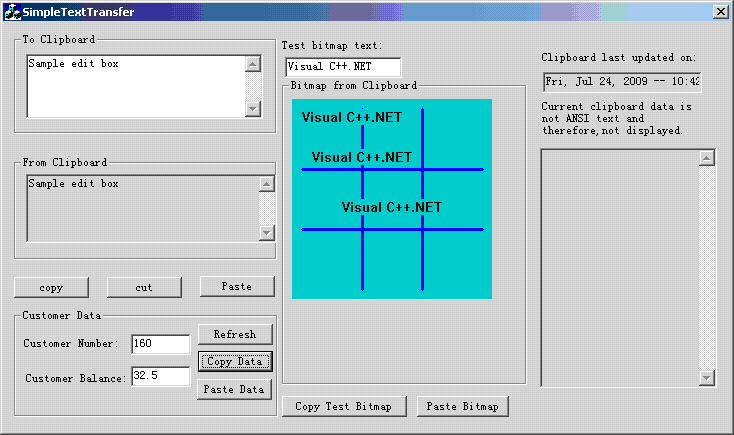
1)传递纯文本
//传递纯文本
void CSimpleTextTransferDlg::OnButton2() //按下Copy按钮事件
{
// TODO: Add your control notification handler code here
if(UpdateData())
{
CString strData;
m_edtToClipboard.GetWindowText(strData);
if(OpenClipboard())
{
EmptyClipboard();
HGLOBAL hClipboardData;
hClipboardData=GlobalAlloc(GMEM_DDESHARE,strData.GetLength()+1);
char* pchData;
pchData=(char*)GlobalLock(hClipboardData);
strcpy(pchData,LPCSTR(strData));
GlobalUnlock(hClipboardData);
SetClipboardData(CF_TEXT,hClipboardData);
CloseClipboard();
}
}
}
void CSimpleTextTransferDlg::OnButton3() //按下cut按钮事件
{
// TODO: Add your control notification handler code here
OnButton2();
m_edtToClipboard.SetWindowText("");
}
void CSimpleTextTransferDlg::OnButton4()//按下Paste按钮事件
{
// TODO: Add your control notification handler code here
if (OpenClipboard())
{
HANDLE hClipboardData=GetClipboardData(CF_TEXT);
char* pchData=(char*)GlobalLock(hClipboardData);
CString StrFromClipboard=pchData;
m_edtFromClipboard.SetWindowText(StrFromClipboard);
GlobalUnlock(hClipboardData);
CloseClipboard();
}
}
2)转移位图图像
//按Alt+Print Screen组合键,可以把当前窗口的位图图像复制到剪贴板上
void CSimpleTextTransferDlg::OnButton7()//按下Paste Bitmap按钮事件,粘帖剪贴板中的位图图像
{
// TODO: Add your control notification handler code here
if(OpenClipboard())
{
if(::IsClipboardFormatAvailable(CF_BITMAP))
{
HBITMAP handle=(HBITMAP)GetClipboardData(CF_BITMAP);
CBitmap* bm=CBitmap::FromHandle(handle);
CClientDC cdc(this);
CDC dc;
dc.CreateCompatibleDC(&cdc);
dc.SelectObject(bm);
RECT rect;
m_grpBitmapFromClipboard.GetWindowRect(&rect);
ScreenToClient(&rect);
cdc.BitBlt(rect.left+10,rect.top+20,(rect.right-rect.left)-10,(rect.bottom-rect.top)-40,&dc,0,0,SRCCOPY);
}
else
{
AfxMessageBox("There is no BITMAP data on the Clipboard");
}
CloseClipboard();
}
}
void CSimpleTextTransferDlg::OnButton6()//按下Copy Test Bitmap按钮事件,吧位图图像复制到剪贴板上
{
// TODO: Add your control notification handler code here
if(OpenClipboard())
{
EmptyClipboard();
CBitmap* pNewBitmap=new CBitmap();
CClientDC cdc(this);
CDC dc;
dc.CreateCompatibleDC(&cdc);
CRect client(0,0,200,200);
pNewBitmap->CreateCompatibleBitmap(&cdc,client.Width(),client.Height());
dc.SelectObject(pNewBitmap);
CString strBitmapText;
m_edtBitmapText.GetWindowText(strBitmapText);
DrawImage(&dc,strBitmapText);
SetClipboardData(CF_BITMAP,pNewBitmap->m_hObject);
CloseClipboard();
delete pNewBitmap;
}
//MessageBox("The bitmap has been copied to clipboard.haha",NULL,MB_OK);
}
void CSimpleTextTransferDlg::DrawImage(CDC *pDC, CString pText)//进行GDI调用进行做图
{
CRect rectDrawingArea(0,0,200,200);
pDC->FillSolidRect(rectDrawingArea,RGB(0,208,200));
CPen pen;
pen.CreatePen(PS_SOLID,3,RGB(0,0,255));
CPen * oldpen=pDC->SelectObject(&pen);
pDC->MoveTo(70,10);
pDC->LineTo(70,190);
pDC->MoveTo(130,10);
pDC->LineTo(130,190);
pDC->MoveTo(10,70);
pDC->LineTo(190,70);
pDC->MoveTo(10,130);
pDC->LineTo(190,130);
pDC->SelectObject(oldpen);
pen.DeleteObject();
pDC->TextOut(10,10,pText);
pDC->TextOut(20,50,pText);
pDC->TextOut(50,100,pText);
}
3)转移自定义数据
在把自定义数据复制到剪贴板上之前,首先要把该数据注册为Windows可以识别的格式。UINT RegisterClipboardFormat(LPCTSTR lpszFormat),lpszFormat是要被注册的格式的名称,该名称可以用任何合法的字符串来表示。
把自定义数据复制到剪贴板上首先,在头文件中添加CCustomer类,接着为Copy Data按钮添加按下事件。
class CCustomer
{
public:
int iCustomerNumber;
float dCustomerBalance;
};
void CSimpleTextTransferDlg::OnButton5()
{
// TODO: Add your control notification handler code here
if(UpdateData())
{
UINT uiCUstomerDataFormat=RegisterClipboardFormat("CUSTOMER_DATA");
if(OpenClipboard())
{
EmptyClipboard();
CCustomer customer;
customer.iCustomerNumber=m_iCustomerNumber;
customer.dCustomerBalance=m_dCustomerBalance;
HGLOBAL hClipboardData;
hClipboardData=GlobalAlloc(GMEM_DDESHARE,sizeof(CCustomer));
CCustomer * pchCustomerData=(CCustomer*)GlobalLock(hClipboardData);
*pchCustomerData=customer;
GlobalUnlock(hClipboardData);
SetClipboardData(uiCUstomerDataFormat,hClipboardData);
CloseClipboard();
}
}
}
从剪贴板上粘贴自定义数据
void CSimpleTextTransferDlg::OnButton8()
{
// TODO: Add your control notification handler code here
UINT uiCUstomerDataFormat=RegisterClipboardFormat("CUSTOMER_DATA");
if(OpenClipboard())
{
if(::IsClipboardFormatAvailable(uiCUstomerDataFormat))
{
HANDLE hData=GetClipboardData(uiCUstomerDataFormat);
CCustomer * pchCustomerData=(CCustomer*)GlobalLock(hData);
CCustomer customer=*pchCustomerData;
m_iCustomerNumber=customer.iCustomerNumber;
m_dCustomerBalance=customer.dCustomerBalance;
UpdateData(FALSE);
GlobalUnlock(hData);
CloseClipboard();
}
}
}
4)接收剪贴板上内容已被修改的通知消息
添加DispalyClipboardData函数来显示剪贴板中的内容
void CSimpleTextTransferDlg::DisplayClipboardData()
{
m_strClipboardText="";
if(OpenClipboard())
{
if(::IsClipboardFormatAvailable(CF_TEXT)||::IsClipboardFormatAvailable(CF_OEMTEXT)||::IsClipboardFormatAvailable(CF_BITMAP))
{
m_strNotExt.ShowWindow(SW_HIDE);
HANDLE hClipboardData=GetClipboardData(CF_TEXT);
char *pchData=(char*)GlobalLock(hClipboardData);
m_strClipboardText=pchData;
GlobalUnlock(hClipboardData);
}
else
{
m_strNotExt.ShowWindow(SW_SHOW);
}
CloseClipboard();
UpdateData(FALSE);
}
}
初始化两个编辑栏的内容
CSimpleTextTransferDlg::CSimpleTextTransferDlg(CWnd* pParent /*=NULL*/)
: CDialog(CSimpleTextTransferDlg::IDD, pParent)
,m_strLastUpdated("<Unknown>")
,m_strClipboardText("")
{
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
在OnInitDialog函数中添加DisplayClipboardData函数和SetClipboardViewer函数(每当剪贴板的内容发生变化时,这个函数将窗口加入被通知的窗口链(通过WM_DRAWCLIPBOARD消息))
BOOL CSimpleTextTransferDlg::OnInitDialog()
{

DisplayClipboardData();
SetClipboardViewer();//每当剪贴板的内容发生变化时,这个函数将窗口加入被通知的窗口链(通过WM_DRAWCLIPBOARD消息)。
return TRUE;
}
手工添加WM_DRAWCLIPBOARD事件,相关函数为OnClipboardChange。
afx_msg LRESULT OnClipboardChange(WPARAM,LPARAM);

ON_MESSAGE(WM_DRAWCLIPBOARD,OnClipboardChange)

LRESULT CSimpleTextTransferDlg::OnClipboardChange(WPARAM,LPARAM)


{
CTime time=CTime::GetCurrentTime();
m_strLastUpdated=time.Format("%a, %b %d, %Y -- %H:%M:%S");
DisplayClipboardData();
return 0L;
}
posted on 2009-07-24 10:55
The_Moment 阅读(5272)
评论(2) 编辑 收藏 引用 所属分类:
VC实践